클래스에 Java 동기화 블록 사용
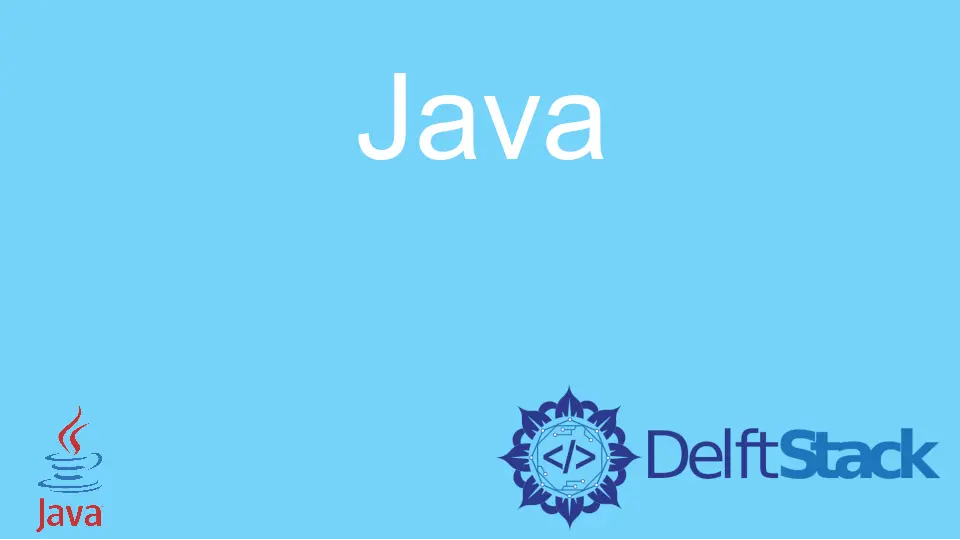
Java에서 동기화는 공유할 수 있는 리소스에 액세스하는 여러 스레드를 관리하는 것입니다. Java에서 동기화는 스레드가 서로 안정적으로 통신할 수 있도록 사용되며 단일 스레드가 공유 리소스에 액세스하는 데 가장 적합한 옵션으로 간주됩니다.
synchronized
키워드는 동기화된 블록을 표시하는 데 사용됩니다. 일부 개체에서는 Java 동기화 블록이 적용됩니다.
클래스에 Java 동기화 블록 사용
동일한 개체에 대한 동기화된 블록에 여러 스레드가 있는 경우 한 스레드만 블록에 들어가고 한 번에 하나의 스레드만 실행할 수 있습니다. 다른 모든 스레드는 차단된 상태로 유지됩니다.
클래스에 대한 Java 동기화 블록을 예제와 함께 설명하겠습니다.
예제 코드:
class Block {
// synchronized block starts from here
void printBlock(int b) {
synchronized (this) {
System.out.println("The block output is: ");
for (int j = 1; j <= 4; j++) {
System.out.println(b * j);
// handles exception
try {
Thread.sleep(500);
} catch (Exception e) {
System.out.println(e);
}
}
}
} // ending
}
class S1 extends Thread {
Block s;
S1(Block s) {
this.s = s;
}
public void run() {
s.printBlock(10);
}
}
class S2 extends Thread {
Block s;
S2(Block s) {
this.s = s;
}
public void run() {
s.printBlock(200);
}
}
class SyncBlock {
// main method
public static void main(String args[]) {
// object creation
Block obj = new Block();
S1 s1 = new S1(obj);
S2 s2 = new S2(obj);
// run the threads s1 and s2
s1.run();
s2.run();
}
}
출력:
The block output is:
10
20
30
40
The block output is:
200
400
600
800
위의 예에는 S1
과 S2
라는 두 개의 스레드가 있습니다. 두 스레드 모두 synchronized
메서드를 호출하는 데 도움이 되는 printBlock
메서드가 있습니다.
S1
스레드 입력 값은 10이고 S2
의 경우 200입니다. 그러면 S1
과 S2
스레드 출력 사이에 블록 출력이 인쇄됩니다.
예제 코드:
import java.util.*;
class synchronize {
String title = "";
public int c = 0;
public void result(String s, List<String> li) {
// only one thread is allowed to change the title simultaneously.
synchronized (this) {
title = s;
c++;
}
li.add(s);
}
}
class SyncBlock {
// main method
public static void main(String[] args) {
// for synchronize class, we have created an object
synchronize ob = new synchronize();
// we have created a list here
List<String> li = new ArrayList<String>();
// for calling the method, a string is passed to it
ob.result("Sprint Planning", li);
System.out.println(ob.title);
}
}
출력:
Sprint Planning
위의 예에서는 클래스 동기화가 생성되었습니다. 이 클래스에서는 synchronized
방법을 사용했습니다.
메서드를 호출하기 위한 입력 값으로 문자열이 전달됩니다. 즉, 스프린트 계획
.
위의 프로그램을 실행하면 출력으로 Sprint Planning
이 표시됩니다.
결론
Java에서 동기화 블록을 논의하는 방법은 여러 가지가 있지만 이 기사에서는 동기화, 동기화 블록 및 작동 방식에 대해 설명했습니다.
동기화 블록을 사용하여 객체에 대해 한 번에 여러 스레드
가 있는 경우 한 번에 하나의 스레드만 실행할 수 있습니다. 블록 내부의 스레드가 성공적으로 실행될 때까지 다른 스레드는 블록에 들어갈 수 없습니다.
따라서 Java의 동기화된 블록 클래스는 예를 들어 블록에 한 번에 하나의 스레드
만 있어야 합니다.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn