How to Stub in Java
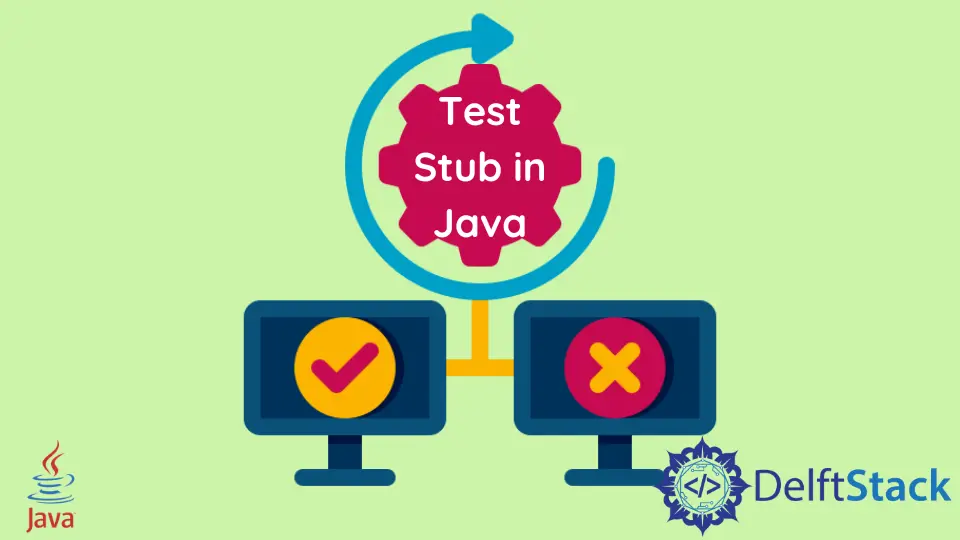
This tutorial teaches us how to create and use stubs that are a general part of testing, also called test doubles. In the following example, we will go through the steps to write a test stub using only JUnit.
Use JUnit to Test Stub in Java
A stub is a class or object that we use in unit tests to return fake data similar to the function’s data when it is in production. An example of this can be a call to an API that gives some data as a response, but when we use a test stub, we hard-code the data to test it.
Below is an example that creates a fake scenario to test in which we test a method that returns a list containing names of customers starting with a specific character.
We create an interface and two classes for this example. Service
is an interface with a function getCustomers()
that returns a list of string types.
Service.java
:
import java.util.List;
public interface Service {
List<String> getCustomers();
}
We create a class JavaExample
that implements the actual code from where the data will be returned. We create an instance of the Service
interface in the class and then initialize it in the constructor.
To return the data, we create a function getCustomersWhoseNamesStartsWithC()
that returns a list of string types. Inside the method, we initialize a list called customers
.
Now we get the list of customers using service.getCustomers()
and loop through it to check if the strings in the list contain the character C
, and if it does, we add that string to the customers
list. Finally, we return the list.
JavaExample.java
:
import java.util.ArrayList;
import java.util.List;
public class JavaExample {
Service service;
public JavaExample(Service service) {
this.service = service;
}
public List<String> getCustomersWhoseNamesStartsWithC() {
List<String> customers = new ArrayList<>();
for (String customerName : service.getCustomers()) {
if (customerName.contains("C"))
customers.add(customerName);
}
return customers;
}
}
Next, we create a class that contains all the test cases. In the class, we create a stub class called StubService
that implements the Service
interface, and in the class, we use the getCustomers()
method to create a list with a few fake names test and return it.
To create a test method, we create a method named whenCallServiceIsStubbed()
and annotate it with @Test
. Inside the method, we create an object of the JavaExample
class and pass the StubService
class as its argument in the constructor.
We test the result returned by getCustomersWhoseNamesStartsWithC()
function using the assertEquals()
method of junit
. In the first assert statement, we check the size of the returned list, and in the second statement, we check if the first item or name of the list is Cathy
.
The output shows that the test passes.
StubTestJava.java
:
import static org.junit.Assert.assertEquals;
import java.util.Arrays;
import java.util.List;
import org.junit.Test;
import testexample.services.Service;
public class StubTestJava {
@Test
public void whenCallServiceIsStubbed() {
JavaExample service = new JavaExample(new StubService());
assertEquals(4, service.getCustomersWhoseNamesStartsWithC().size());
assertEquals("Cathy", service.getCustomersWhoseNamesStartsWithC().get(0));
}
static class StubService implements Service {
public List<String> getCustomers() {
return Arrays.asList("Cathy", "Carla", "Kevin", "Denis", "Charles", "Caleb");
}
}
}
Output:
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn