How to Clear a StringBuilder in Java
-
Using the
setLength(0)
Method to Clear or Empty aStringBuilder
in Java -
Assign a New Object to a
StringBuilder
Variable to Clear or Empty It in Java -
Using the
delete()
Method to Clear or Empty aStringBuilder
in Java -
Converting
StringBuilder
to a String and Reassign a NewStringBuilder
Method to Clear or Empty aStringBuilder
in Java - Conclusion
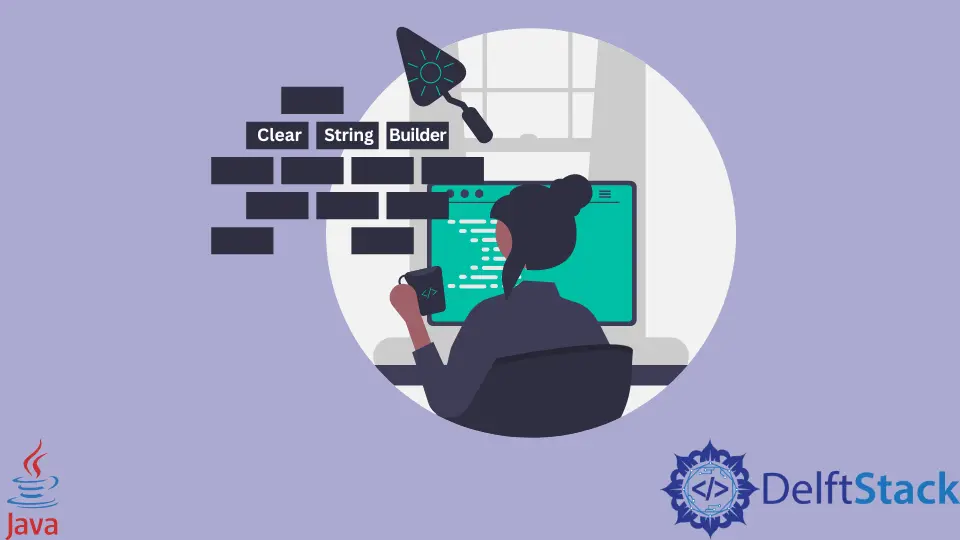
This Java guide will show you how to clear a StringBuilder
. It’s a class in Java that implements different interfaces.
Clearing a StringBuilder
is crucial for reusability and memory management. It allows developers to reset the content of the StringBuilder
efficiently, providing a clean slate for new data or operations.
This not only enhances code clarity but also avoids unnecessary memory consumption, ensuring optimal performance. Whether in iterative processes or dynamic string manipulation, clearing a StringBuilder
enables developers to maintain efficiency and readability in their Java programs.
Using the setLength(0)
Method to Clear or Empty a StringBuilder
in Java
Using setLength(0)
to clear a StringBuilder
is concise and efficient. It directly sets the length of the character sequence to 0, providing a straightforward way to reset the content without creating a new instance or iterating through characters.
This method is particularly advantageous for simplicity and performance in scenarios where a fresh start is needed.
Let’s explore the concept through a practical code example:
public class ClearingStringBuilder {
public static void main(String[] args) {
// Create a StringBuilder instance with some initial content
StringBuilder sb = new StringBuilder("Hello, World!");
// Display the original content
System.out.println("Original Content: " + sb);
// Clear the content using setLength(0)
sb.setLength(0);
// Display the cleared content
System.out.println("Cleared Content: " + sb);
}
}
In the initialization and display section, a StringBuilder
instance named sb
is created and loaded with the string Hello, World!
. The original content is then showcased using System.out.println()
.
Oon to the content clearing step, the setLength(0)
method is employed on the StringBuilder
instance sb
. By setting the length to 0
, this method efficiently clears the content, providing a clean slate for future use.
Subsequently, the cleared content is displayed, confirming the success of the setLength(0)
method in effectively resetting the StringBuilder
content.
The output of the code would be:
Original Content: Hello, World!
Cleared Content:
As expected, the original content is displayed, followed by the cleared content, demonstrating the effectiveness of using setLength(0)
to clear a StringBuilder
.
Assign a New Object to a StringBuilder
Variable to Clear or Empty It in Java
Creating a new StringBuilder
instance is a direct and effective method to clear content. It ensures simplicity by discarding the existing content and provides a fresh, empty instance, contributing to code clarity and efficient memory usage.
This approach is particularly advantageous when a clean slate is needed, offering a straightforward alternative to other clearing methods.
Let’s delve into a practical example to illustrate the process:
public class ClearingStringBuilder {
public static void main(String[] args) {
// Create a StringBuilder instance with some initial content
StringBuilder sb = new StringBuilder("Hello, World!");
// Display the original content
System.out.println("Original Content: " + sb);
// Clear the content by creating a new instance
sb = new StringBuilder();
// Display the cleared content
System.out.println("Cleared Content: " + sb);
}
}
In the initialization and display section, a StringBuilder
named sb
is initiated with the string Hello, World!
, and the original content is revealed through System.out.println()
.
Transitioning to content clearing, a new instance of StringBuilder
is created, replacing the existing one assigned to sb
. This straightforward method discards prior content, offering a fresh start for subsequent operations.
Consequently, the cleared content is exhibited, affirming the success of the new instance approach in effectively erasing the StringBuilder
content.
The output of the code would be:
Original Content: Hello, World!
Cleared Content:
As expected, the original content is displayed first, followed by the cleared content. This demonstrates the effectiveness of the approach, where creating a new StringBuilder
instance serves as a concise and efficient method to clear the content of the original instance.
Using the delete()
Method to Clear or Empty a StringBuilder
in Java
Utilizing the delete()
method in clearing a StringBuilder
is vital for precision and versatility. Specifying a range allows selective content removal, providing a targeted and efficient way to clear portions while preserving the rest.
This method stands out for its flexibility, making it valuable in scenarios where partial content clearance is advantageous over complete resetting or creating new instances.
The syntax for using the delete()
method in a StringBuilder
to clear a specific range of characters is as follows:
sb.delete(startIndex, endIndex);
In using the delete()
method in a StringBuilder
, the syntax involves specifying three parameters.
First is the StringBuilder
instance (sb
), which is the target object for the delete()
operation.
The second parameter is startIndex
, representing the inclusive starting index of the character range to be deleted.
Finally, endIndex
serves as the exclusive ending index, indicating where the deletion should stop.
Together, these parameters define the range of characters to be removed from the StringBuilder
. This syntax allows you to selectively remove characters from the StringBuilder
based on the specified range, leaving the rest of the content intact.
Let’s delve into a practical example:
public class ClearingStringBuilder {
public static void main(String[] args) {
// Create a StringBuilder instance with some initial content
StringBuilder sb = new StringBuilder("Hello, World!");
// Display the original content
System.out.println("Original Content: " + sb);
// Clear specific characters using delete() method
sb.delete(7, 12);
// Display the content after selective removal
System.out.println("Content after Deletion: " + sb);
}
}
In the initialization and display section, we set up a StringBuilder
named sb
with the string Hello, World!
and present the original content using System.out.println()
.
Moving to the content clearing step with the delete()
method, we apply it to sb
, specifying the range from index 7 to 11 (exclusive). This targeted approach selectively removes characters, effectively clearing a specific portion of the content.
Consequently, in the display content after selective removal, we exhibit the updated content of StringBuilder
post the selective removal operation. This confirms the efficacy of the delete()
method in clearing only the specified characters while leaving the rest of the content intact.
The output of the code would be:
Original Content: Hello, World!
Content after Deletion: Hello, !
As expected, the original content is displayed, followed by the content after the selective removal. This demonstrates how the delete()
method effectively clears specific characters, showcasing its flexibility in content management within a StringBuilder
.
Converting StringBuilder
to a String and Reassign a New StringBuilder
Method to Clear or Empty a StringBuilder
in Java
In Java programming, the StringBuilder
class is often used for dynamic string manipulations. When the need arises to clear the content of a StringBuilder
, an elegant approach is to convert it to a String
and then reassign a new StringBuilder
.
This method offers simplicity and clarity, allowing developers to effectively clear the content without the need for intricate operations. In this article, we will explore the concept of clearing a StringBuilder
using the convert to String and reassign
method, understanding its importance and practical applications.
Let’s dive into a practical example:
public class ClearingStringBuilder {
public static void main(String[] args) {
// Create a StringBuilder instance with some initial content
StringBuilder sb = new StringBuilder("Hello, World!");
// Display the original content
System.out.println("Original Content: " + sb);
// Clear the content by converting to String and reassigning
sb = new StringBuilder();
// Display the cleared content
System.out.println("Cleared Content: " + sb);
}
}
In the initialization and display phase, a StringBuilder
named sb
is set up with the string Hello, World!
and its original content is showcased using System.out.println()
.
Moving on to the content clearing step by converting to String and reassigning, a new instance of StringBuilder
is created, and sb
is reassigned to it. This straightforward method efficiently clears the content, offering a fresh start for subsequent operations.
Finally, in displaying the cleared content, we present the updated state of the StringBuilder
after the clearing operation. This confirms the success of the convert to String and reassign
method, demonstrating that the content has been effectively cleared, leaving the StringBuilder
empty.
The output of the code would be:
Original Content: Hello, World!
Cleared Content:
As expected, the original content is displayed first, followed by the cleared content. This demonstrates the effectiveness of using the convert to String and reassign
method to clear the content of a StringBuilder
, providing a concise and efficient way to reset the instance.
Conclusion
Clearing a StringBuilder
in Java offers multiple methods catering to diverse needs.
The setLength(0)
method provides a direct reset, creating a new instance offers simplicity, delete()
allows selective removal, setCharAt
in a loop provides targeted clearing, and converting to String and reassigning offers a concise reset. The choice among these methods depends on the specific requirements of the task, allowing developers flexibility in efficiently managing content within a StringBuilder
.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn