How to Make a Stopwatch in Java
-
Stopwatch in Java Using
System.nanoTime()
- Stopwatch in Java Using Apache Commons Lang
-
Stopwatch in Java Using
Instant
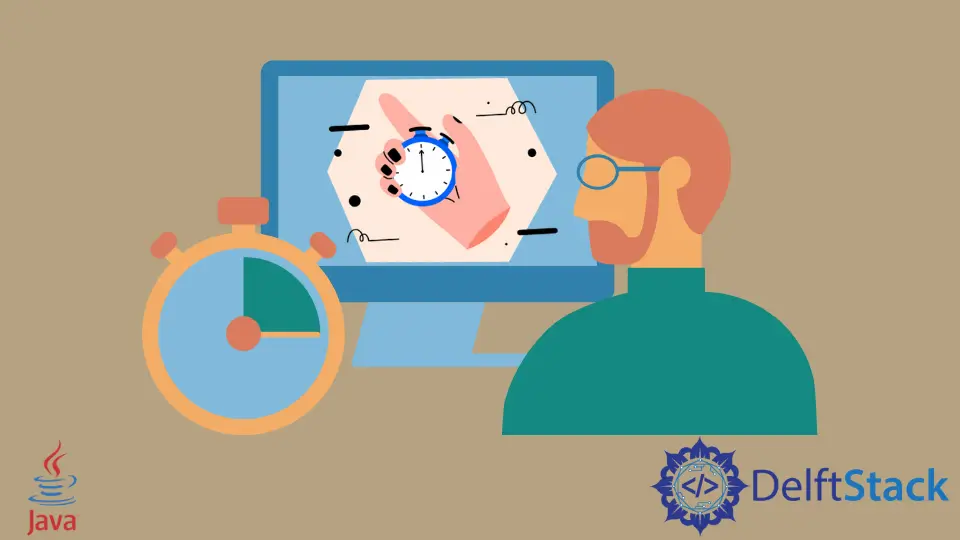
We can get the elapsed time of a specific task in Java using several methods that we are going to see below. A java stopwatch is needed when the task is time crucial, and we want to know the time taken by a long-running task like calculating a Fibonacci series.
Stopwatch in Java Using System.nanoTime()
We want to keep the stopwatch time as accurate as possible, and thus to do that, we use the System.nanotTime()
class that returns the nanoseconds value that is the most precise value of the system timer.
In the program, we create two classes, Stopwatch1
and Stopwatch
. In the Stopwatch1
two methods to start and stop the stopwatch, start()
initializes the stopWatchStartTime
with the current nanoseconds using System.nanoTime()
and set the value of stopWatchRunning
(checks if the stopwatch is running) as true
.
stop()
initializes the stopWatchStopTime
and sets stopWatchRunning
to false
. Then, we can use four functions to calculate the total elapsed time in milliseconds, seconds, minutes, and hours. Each method has a local variable called elapsedTime
that stores the value that is the value of System.nanoTime()
subtracted by the stopWatchStartTime
if the Stopwatch is running and it is not running, then we do stopWatchStopTime - stopWatchStartTime
.
Now, as the value of elapsedTime
in every function is in nanoseconds, we convert its value to different time units by dividing elapsedTime
by the nanoseconds it holds.
In the Stopwatch
class, we create an object of Stopwatch1
and call the start()
method. Then we call the method that calculates the Fibonacci series using the recursion method, and at last, we call the stop()
function. The time between the start()
and stop()
functions is the elapsed time shown in the output.
import java.math.BigInteger;
class Stopwatch1 {
private final long nanoSecondsPerMillisecond = 1000000;
private final long nanoSecondsPerSecond = 1000000000;
private final long nanoSecondsPerMinute = 60000000000L;
private final long nanoSecondsPerHour = 3600000000000L;
private long stopWatchStartTime = 0;
private long stopWatchStopTime = 0;
private boolean stopWatchRunning = false;
public void start() {
this.stopWatchStartTime = System.nanoTime();
this.stopWatchRunning = true;
}
public void stop() {
this.stopWatchStopTime = System.nanoTime();
this.stopWatchRunning = false;
}
public long getElapsedMilliseconds() {
long elapsedTime;
if (stopWatchRunning)
elapsedTime = (System.nanoTime() - stopWatchStartTime);
else
elapsedTime = (stopWatchStopTime - stopWatchStartTime);
return elapsedTime / nanoSecondsPerMillisecond;
}
public long getElapsedSeconds() {
long elapsedTime;
if (stopWatchRunning)
elapsedTime = (System.nanoTime() - stopWatchStartTime);
else
elapsedTime = (stopWatchStopTime - stopWatchStartTime);
return elapsedTime / nanoSecondsPerSecond;
}
public long getElapsedMinutes() {
long elapsedTime;
if (stopWatchRunning)
elapsedTime = (System.nanoTime() - stopWatchStartTime);
else
elapsedTime = (stopWatchStopTime - stopWatchStartTime);
return elapsedTime / nanoSecondsPerMinute;
}
public long getElapsedHours() {
long elapsedTime;
if (stopWatchRunning)
elapsedTime = (System.nanoTime() - stopWatchStartTime);
else
elapsedTime = (stopWatchStopTime - stopWatchStartTime);
return elapsedTime / nanoSecondsPerHour;
}
}
public class Stopwatch {
public static void main(String[] args) {
Stopwatch1 stopwatch1 = new Stopwatch1();
stopwatch1.start();
Fibonacci(45);
stopwatch1.stop();
System.out.println("Elapsed time in milliseconds: "
+ stopwatch1.getElapsedMilliseconds());
System.out.println("Elapsed time in seconds: "
+ stopwatch1.getElapsedSeconds());
System.out.println("Elapsed time in minutes: "
+ stopwatch1.getElapsedMinutes());
System.out.println("Elapsed time in hours: "
+ stopwatch1.getElapsedHours());
}
private static BigInteger Fibonacci(int n) {
if (n < 2)
return BigInteger.ONE;
else
return Fibonacci(n - 1).add(Fibonacci(n - 2));
}
}
Output:
Elapsed time in milliseconds: 31161
Elapsed time in seconds: 31
Elapsed time in minutes: 0
Elapsed time in hours: 0
Stopwatch in Java Using Apache Commons Lang
For this example, we use the Apache Commons library. Below is the dependency to include it in our project.
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.11</version>
</dependency>
We create an object of StopWatch
class stopWatch
that comes with the library and then call the start()
function. After starting the stopwatch we call the Fibonacci()
function and then stop the watch using stop()
. Now to get the elapsed time we call stopWatch.getTime()
that returns the elapsed time and in getTime()
we can pass different time units.
import java.math.BigInteger;
import java.util.concurrent.TimeUnit;
import org.apache.commons.lang3.time.StopWatch;
public class Stopwatch {
public static void main(String[] args) {
StopWatch stopWatch = new StopWatch();
stopWatch.start();
Fibonacci(40);
stopWatch.stop();
System.out.println(
"Get elapsed time in milliseconds: " + stopWatch.getTime(TimeUnit.MILLISECONDS));
System.out.println("Get elapsed time in seconds: " + stopWatch.getTime(TimeUnit.SECONDS));
System.out.println("Get elapsed time in minutes: " + stopWatch.getTime(TimeUnit.MINUTES));
System.out.println("Get elapsed time in hours: " + stopWatch.getTime(TimeUnit.HOURS));
}
private static BigInteger Fibonacci(int n) {
if (n < 2)
return BigInteger.ONE;
else
return Fibonacci(n - 1).add(Fibonacci(n - 2));
}
}
Output:
Get elapsed time in milliseconds: 3483
Get elapsed time in seconds: 3
Get elapsed time in minutes: 0
Get elapsed time in hours: 0
Stopwatch in Java Using Instant
Instant
is a part of the java.time
package. It returns the current instant from the system clock. We two instant values using Instant.now()
first is before the execution of Fibonacci()
and one after the execution. Then we calculate the difference between both the values using Duration.between()
where we pass the instatStarted
and instantStopped
values that returns a Duration
which we can convert to different time units like toMillis()
for milliseconds, toSeconds()
for seconds and for minutes we use toMinutes()
.
import java.math.BigInteger;
import java.time.Duration;
import java.time.Instant;
public class Stopwatch {
public static void main(String[] args) {
Instant instantStarted = Instant.now();
Fibonacci(40);
Instant instantStopped = Instant.now();
Duration durationBetween = Duration.between(instantStarted, instantStopped);
System.out.println("Get elapsed time in milliseconds: " + durationBetween.toMillis());
System.out.println("Get elapsed time in seconds: " + durationBetween.toSeconds());
System.out.println("Get elapsed time in minutes: " + durationBetween.toMinutes());
}
private static BigInteger Fibonacci(int n) {
if (n < 2)
return BigInteger.ONE;
else
return Fibonacci(n - 1).add(Fibonacci(n - 2));
}
}
Output:
Get elapsed time in milliseconds: 3510
Get elapsed time in seconds: 3
Get elapsed time in minutes: 0
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn