What Does Static Mean in Java
-
Use of the
static
Keyword Along With Member Variables in Java -
Use of the
static
Keyword Along With Member Functions in Java -
Use of the
static
Keyword in a Block in Java
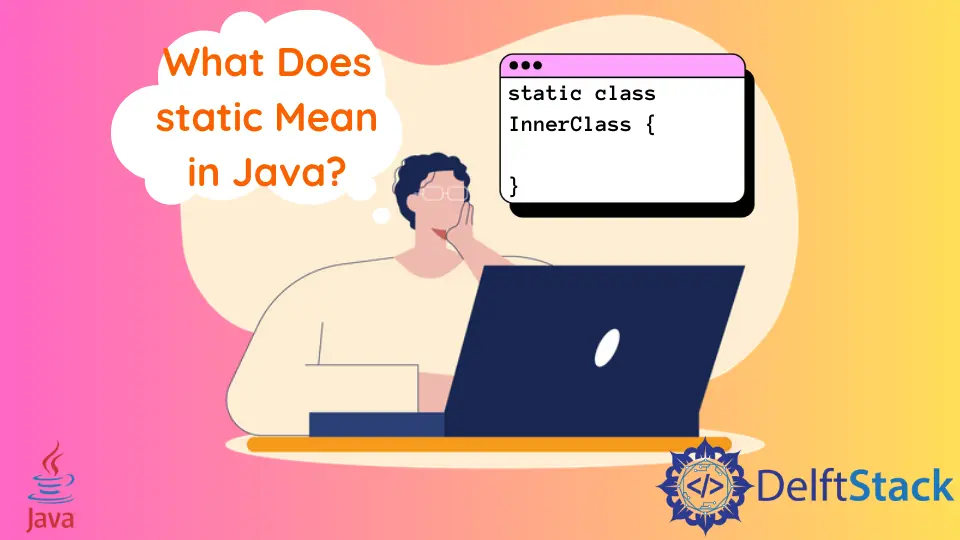
In Java language, static
is a keyword that can have its usage at various places. The static
keyword gets used along with a member variable, member functions, blocks, and nested classes. The application of static keywords is wherever we do not want to create a new instance every time. Instead, we use it at places where the single copy gets shared within the class. Static variables get stored in the heap memory, which is a type of permanent memory.
Use of the static
Keyword Along With Member Variables in Java
The code block explains how the static variable is different from non-static variables.
public class StaticVariableUsage {
public static void main(String[] args) {
InnerClass in = new InnerClass();
InnerClass in1 = new InnerClass();
InnerClass2 in2 = new InnerClass2();
InnerClass2 in3 = new InnerClass2();
}
static class InnerClass {
static int var4 = 0;
InnerClass() {
var4++;
System.out.println("static variable : " + var4);
}
}
static class InnerClass2 {
int var5 = 0;
InnerClass2() {
var5++;
System.out.println("non-static variable : " + var5);
}
}
}
In the above code block, there is a public class named the StaticVariableUsage
class. It has one static method and two inner classes. The inner class named InnerClass
has a static integer variable var4
and gets initiated with zero. The class has a constructor that increments the static integer variable and prints its value.
Another inner class has the name InnerClass2
and holds a non-static variable var5
. This class has a constructor too that increments the non-static variable and prints its value.
Moving to the main
method, here, InnerClass
and InnerClass2
are instantiated twice. The analysis can be derived when the constructor call happens an object gets instantiated with a new keyword.
As the output says, the static variable is initiated and gets preserved. The value is incremented for the first time and prints the value as 1
. Next time, when we create another instance, the constructor gets called again. But the static variable is not re-initialized with zero again. Instead, the previously stored value gets incremented, hence, making the static variable value two.
Similarly, when an instance of InnerClass2
gets created, each time non-static variable gets initialized to value 0
. So it prints value as 1
in output both the times.
static variable : 1
static variable : 2
non-static variable : 1
non-static variable : 1
Use of the static
Keyword Along With Member Functions in Java
We have an outer class named StaticFunctionUsage
class that holds the other inner class in the below class. InnerClass
has a static function. And another inner class named InnerClass1
has a non-static method.
Now, moving on to the main
method, it is seen that the static function is directly accessible with the class name. We do not have to instantiate the InnerClass
object. Similarly, when we try to access the non-static function function1
with the class name, a compile-time error is populated. If we want to access the function, the class instance needs to get created, which we created with the name in
variable. The use of the variable is to call function1
and is a valid statement.
public class StaticFunctionUsage {
public static void main(String[] args) {
InnerClass.function();
// InnerClass1.function1();
InnerClass1 in = new InnerClass1();
in.function1();
}
static class InnerClass {
static void function() {
System.out.println("In the static function");
}
}
static class InnerClass1 {
void function1() {
System.out.println("In the non-static function");
}
}
}
The output of the above code block is as below.
In the static function
In the non-static function
Use of the static
Keyword in a Block in Java
The use of static blocks is to initialize static variables. The execution of the program starts with the main
method. If a static block is present within a class, then it executes before the main method execution.
Below is the code explaining the static block.
public class StaticBlock {
static {
System.out.println("In the static block");
}
public static void main(String[] args) {
System.out.println("In the main method");
}
}
The output of the code is as below. As we can see, the statement in the static block gets firstly executed.
In the static block
In the main method
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn