How to Autowire in Java Spring Boot
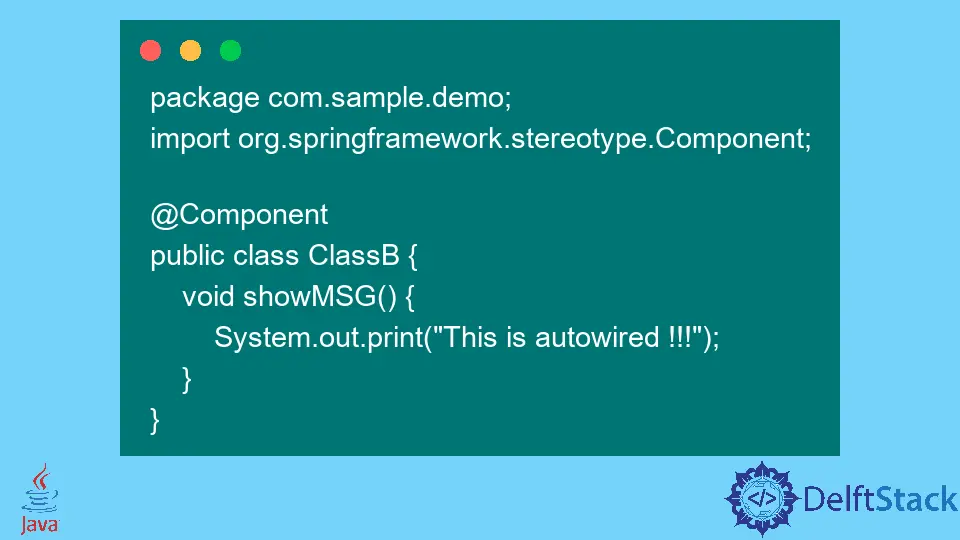
Spring Boot is a popular framework of Java. In Spring Boot, the most commonly used annotation is @Autowired
, mainly used to automatically collect the bean.
In this article, we will discuss the @Autowired
in Java Spring Boot.
Used Modes for @Autowired
Annotation
The following are the commonly used modes for the @Autowired
annotation.
Modes | Description |
---|---|
no |
This is the default mode of autowiring , and it disables the autowiring by default. |
byName |
This mode injects the object dependency according to the name of the bean. |
byType |
This mode injects the object dependency according to the type of the bean and is also called the setter method. |
constructor |
This mode injects the object dependency by calling the constructor . |
Utilize @Autowired
in Java Spring Boot
First, we will create a class named ClassB
which we want to autowired
from the parent class. The code example for this class will be like the following.
package com.sample.demo;
import org.springframework.stereotype.Component;
@Component
public class ClassB {
void showMSG() {
System.out.print("This is autowired !!!");
}
}
This child class contains the method named showMSG()
, and we declared it as a bean
component using the annotation @Componant
. Now we are going to look at its parent class.
Below is the code for our parent class.
package com.sample.demo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class ClassA {
@Autowired ClassB b;
void getMSG() {
b.showMSG();
}
}
In our parent class, we autowired
the ClassB
and this class contains a method named getMSG()
. We declared this class as a bean
component using the annotation @Componant
.
Now let’s take a look at our handler class.
package com.sample.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ApplicationContext;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
ApplicationContext context = SpringApplication.run(DemoApplication.class, args);
ClassA a = context.getBean(ClassA.class);
a.getMSG();
}
}
Here, we get the bean
of ClassA
from the context
. After that, we called the method getMSG()
from the bean
.
Output:
This is autowired
Please note that the code snippets shared in this article are written in the Java Spring Boot framework. You must install the necessary plugins for your IDE before executing this program.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn