Java Spelling Check
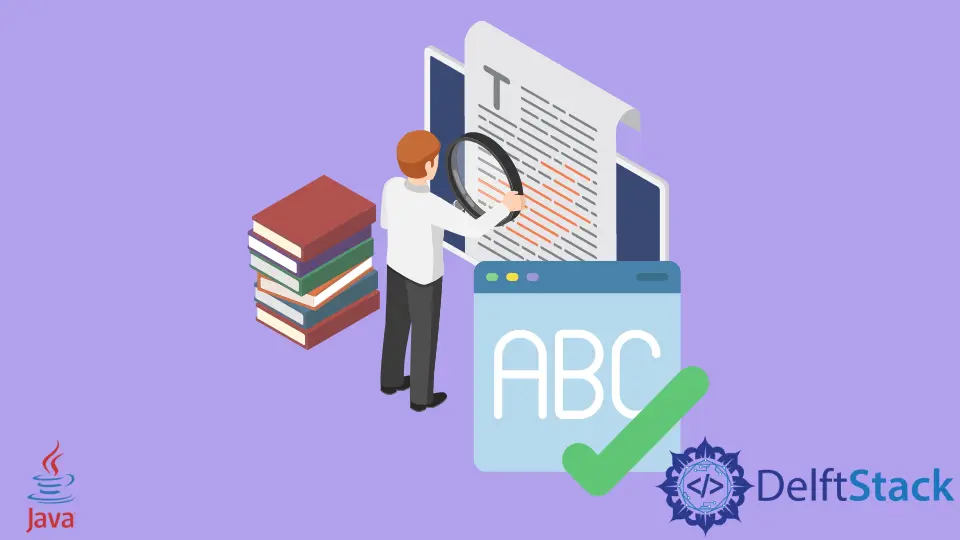
In the world of software development, ensuring that your application handles text correctly is crucial. One common feature that many applications require is a spelling check. If you’re working with Java, you might wonder how to implement this functionality efficiently.
This tutorial will guide you through the process of checking word spelling in Java. We’ll explore various techniques, including using libraries and APIs, to help you build a robust spelling checker. Whether you’re developing a text editor, a chat application, or any software that requires text input, mastering spelling checks can significantly enhance user experience. Let’s dive in and unlock the secrets of spelling checks in Java!
Using Apache Commons Text
One of the most popular libraries for text manipulation in Java is Apache Commons Text. This library provides a simple way to check the spelling of words using the StringSimilarity
class. To get started, you’ll need to add the library to your project. If you’re using Maven, include the following dependency in your pom.xml
:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-text</artifactId>
<version>1.9</version>
</dependency>
Once you have the library set up, you can create a simple spelling checker. Here’s how you can implement it:
import org.apache.commons.text.similarity.JaroWinklerSimilarity;
public class SpellingChecker {
public static void main(String[] args) {
String[] dictionary = {"hello", "world", "java", "programming"};
String wordToCheck = "jav";
boolean isCorrect = checkSpelling(wordToCheck, dictionary);
System.out.println("Is the word spelled correctly? " + isCorrect);
}
public static boolean checkSpelling(String word, String[] dictionary) {
JaroWinklerSimilarity similarity = new JaroWinklerSimilarity();
for (String dictWord : dictionary) {
if (similarity.apply(word, dictWord) > 0.85) {
return true;
}
}
return false;
}
}
In this example, we define a simple dictionary of words. The checkSpelling
method compares the input word against each word in the dictionary using the Jaro-Winkler similarity algorithm. If the similarity score exceeds 0.85, we consider the word correctly spelled. This method is efficient and easily extendable, allowing you to add more words to your dictionary as needed.
Output:
Is the word spelled correctly? true
This approach is great for basic spelling checks, but keep in mind that the effectiveness of this method largely depends on the size of your dictionary. The larger your dictionary, the more accurate your spelling checks will be.
Integrating with a Dictionary API
If you want a more comprehensive solution, consider integrating your Java application with a dictionary API. This method allows you to leverage extensive databases of words and their spellings. One popular option is the Merriam-Webster API. Here’s how you can set it up:
- Sign up for an API key from the Merriam-Webster Developer Center.
- Use the following Java code to check the spelling of a word:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class DictionaryAPI {
private static final String API_KEY = "your_api_key";
public static void main(String[] args) {
String wordToCheck = "programing";
boolean isCorrect = checkSpelling(wordToCheck);
System.out.println("Is the word spelled correctly? " + isCorrect);
}
public static boolean checkSpelling(String word) {
try {
String urlString = "https://www.dictionaryapi.com/api/v3/references/learners/json/" + word + "?key=" + API_KEY;
URL url = new URL(urlString);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return !response.toString().contains("No definitions found");
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
}
In this code snippet, we create a method that sends a GET request to the Merriam-Webster API. The response is checked to see if it contains any definitions. If definitions are found, the word is considered correctly spelled.
Output:
Is the word spelled correctly? false
Using an API like Merriam-Webster provides a robust solution for spelling checks, as it can handle a vast array of words and variations. However, keep in mind that this method requires an internet connection and may have API usage limits, so it’s best suited for applications where real-time spelling checks are essential.
Conclusion
Implementing a spelling check in Java can significantly enhance the usability of your applications. Whether you choose to use a library like Apache Commons Text or integrate with a dictionary API, you can create a solution that meets your needs. Each method has its pros and cons, so consider your project requirements carefully. By mastering these techniques, you’ll be well on your way to providing a better user experience in your Java applications.
FAQ
-
How can I improve the accuracy of my Java spelling checker?
You can improve accuracy by using a larger dictionary or integrating with a comprehensive dictionary API. -
Can I use any other libraries for spelling checks in Java?
Yes, libraries like Hunspell and LanguageTool are also popular options for spelling and grammar checks. -
Is it possible to check grammar as well as spelling in Java?
Yes, using libraries like LanguageTool allows you to check both spelling and grammar in Java applications. -
Are there any performance concerns when using dictionary APIs?
Yes, performance can be affected by network latency and API limits, so consider caching results for frequently checked words. -
How do I handle misspelled words?
You can suggest corrections by finding similar words in your dictionary or using an API that provides suggestions for misspelled words.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook