Java Lang Runtime exec() Method in Java
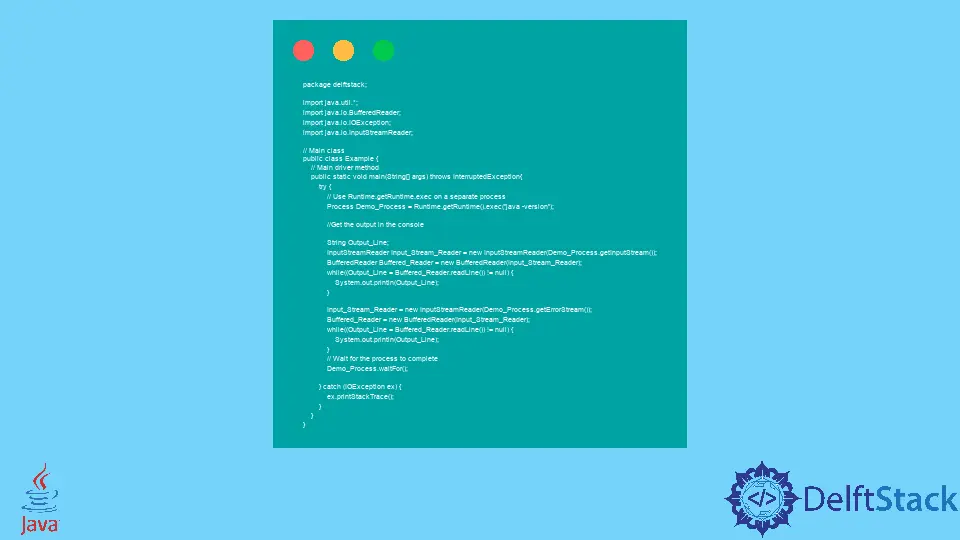
This tutorial demonstrates Java’s Runtime.exec()
method.
Java Lang Runtime exec()
Method in Java
The Java Lang Runtime class is used for the interaction between every Java application with an instance of class runtime and the environment interface. The Runtime.getRuntime.exec()
method is used to execute a string command in a process.
The Runtime.exec()
method can be implemented in the following ways:
Method | Action |
---|---|
exec(String cmd) |
This is used to execute a string command in a process. |
exec(String[] cmd) |
This is used to execute specific commands with arguments in a process. |
exec(String command, String[] envp, File dir) |
This is used to execute a single string command in a process with a specific environment and directory. |
exec(String command, String[] envp) |
This is used to execute a single string command in a process with a specific environment. |
exec(String[] cmdarray, String[] envp, File dir) |
This is used to execute string commands with arguments in a process with a specific environment and directory. |
exec(String[] cmdarray, String[] envp) |
This is used to execute string commands with arguments in a process with a specific environment. |
Let’s try an example to implement the Runtime.exec()
method in Java:
package delftstack;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.*;
// Main class
public class Example {
// Main driver method
public static void main(String[] args) throws InterruptedException {
try {
// Use Runtime.getRuntime.exec on a separate process
Process Demo_Process = Runtime.getRuntime().exec("java -version");
// Get the output in the console
String Output_Line;
InputStreamReader Input_Stream_Reader = new InputStreamReader(Demo_Process.getInputStream());
BufferedReader Buffered_Reader = new BufferedReader(Input_Stream_Reader);
while ((Output_Line = Buffered_Reader.readLine()) != null) {
System.out.println(Output_Line);
}
Input_Stream_Reader = new InputStreamReader(Demo_Process.getErrorStream());
Buffered_Reader = new BufferedReader(Input_Stream_Reader);
while ((Output_Line = Buffered_Reader.readLine()) != null) {
System.out.println(Output_Line);
}
// Wait for the process to complete
Demo_Process.waitFor();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
The code above runs the method Runtime.getRuntime.exec()
with the java -version
command and gets the output in the console. See output
java version "17.0.2" 2022-01-18 LTS
Java(TM) SE Runtime Environment (build 17.0.2+8-LTS-86)
Java HotSpot(TM) 64-Bit Server VM (build 17.0.2+8-LTS-86, mixed mode, sharing)
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook