Runnable VS Callable Interface in Java
-
The
Runnable
Interface in Java -
The
Runnable
Interface Example in Java -
The
Callable
Interface in Java -
The
Callable
Interface Example in Java -
Runnable
VSCallable
Interface in Java
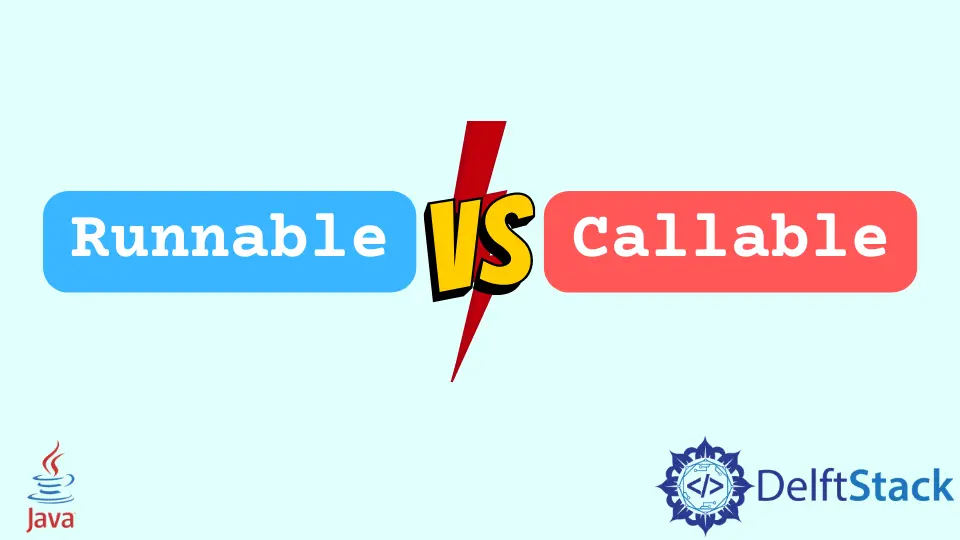
This tutorial introduces the difference between Runnable
and Callable
interfaces with examples in Java.
The Runnable
Interface in Java
Runnable
is an interface used to create and run threads in Java. It has a single abstract method run()
only and is also known as a Functional
interface.
You can see the signature of the Runnable
interface and its SAM method below.
@FunctionalInterface public interface Runnable
void run() // Single Abstract Method
The Runnable
Interface Example in Java
Every class that wants to create and run a thread must implement either a Runnable
interface or extend the Thread
class.
The Runnable
interface provides a run()
method that must be overridden in the base class. This method executes implicitly by the start()
method. See the example below.
public class SimpleTesting implements Runnable {
public static void main(String[] args) {
new Thread(new SimpleTesting()).start();
}
@Override
public void run() {
System.out.println("Running a thread");
}
}
Output:
Running a thread
The Callable
Interface in Java
The Callable
is an interface and is similar to the Runnable
interface. It also contains a single abstract method, call()
.
This interface is designed for classes whose instances are potentially executed by another thread. The signature of the Callable
interface and method is below:
The Executors
class contains utility methods to convert to Callable
classes from other common forms.
@FunctionalInterface public interface Callable<V>
V call() throws Exception
The Callable
Interface Example in Java
We implemented the Callable
interface and overridden its call()
method. We can get any object to the call()
method.
See the example below.
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class SimpleTesting implements Callable<Integer> {
public static void main(String[] args) throws InterruptedException, ExecutionException {
ExecutorService executorService = Executors.newSingleThreadExecutor();
SimpleTesting st = new SimpleTesting();
Future<Integer> future = executorService.submit(st);
int result = future.get().intValue();
System.out.println("product " + result);
}
@Override
public Integer call() throws Exception {
int product = 23 * 12;
return product;
}
}
Output:
product 276
Runnable
VS Callable
Interface in Java
The following table contains some points to understand Java’s Runnable
and Callable
interface.
Runnable |
Callable |
---|---|
The implementing class must override its run() method accordingly. |
The implementing class must override its call() method accordingly. |
The return type of the run() method is void hence does not return anything to the caller. |
The return type of the call() method is an Object. It can be a String, an Integer, etc. |
The run() method does not throw any exception. |
The call() method can throw an exception during execution. |
It can be used to create threads in Java. | It cannot be used to create threads. |