List of Rule Engines in Java
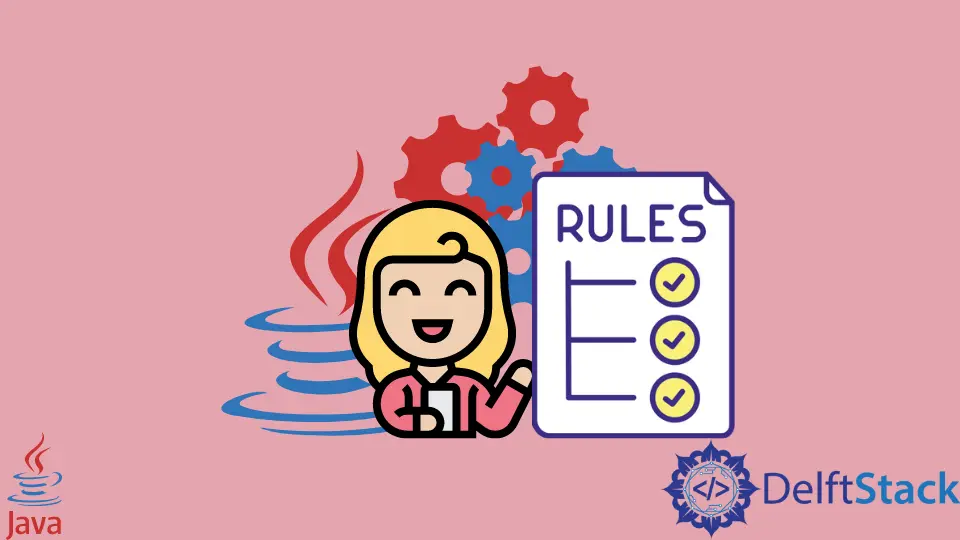
The Rule engines separate the business logic from the source code.
This tutorial describes some of the most popular rule engines of Java.
List of Rule Engines in Java
The Rule Engines make it easy to separate the business logic from the code. Most of the popular rule engines in Java implement the JSR94.
We can see the rule engines as a sophisticated if/then
statement. Here are some advantages of rule engines in Java:
- The rule engines provide great reusability when we keep them separate.
- The rule engines reduce the complexity because we don’t need to develop the source code.
- The rule engines provide flexibility where we can change the rules without changing anything in the source code.
Here are some famous rule engines in Java:
Drools
Drools, also known as business rules management system BRMS
, provide a core business rule engine with a web authoring and rules management application. It also provides full runtime support for the decision model with notation (DMN) and a plugin for Eclipse IDE.
Rule Book
The RuleBook provides a simple DSL that is flexible; if we have a larger collection of rules, we can build them into annotated POJOs, and the RuleBook will transform the package into a rule book. It is easy to use lambda-enabled specific language.
Let’s try a simple rule definition using the RuleBook:
The maven dependency for the rule book is:
<dependency>
<groupId>com.deliveredtechnologies</groupId>
<artifactId>rulebook-core</artifactId>
<version>${version}</version>
</dependency>
Now let’s see the rule book example. Let’s first create a rule:
public class DelftstackRule {
public RuleBook<Object> defineDelftstackRules() {
return RuleBookBuilder.create()
.addRule(rule -> rule.withNoSpecifiedFactType().then(f -> System.out.print("Delftstack")))
.addRule(rule
-> rule.withNoSpecifiedFactType().then(
f -> System.out.println("The Best Tutorial Site")))
.build();
}
}
The main method for the above rule will be:
public static void main(String[] args) {
DelftstackRule ruleBook = new DelftstackRule();
ruleBook.defineDelftstackRules().run(new FactMap<>());
}
Easy Rules
Easy Rules, a lightweight rule engine API, provides the rule abstraction to create rules based on actions and conditions. The EasyRule RulesEngine API runs through a set of rules to execute actions and test conditions.
It is also annotation and POJO based also supports composite rule constructions; it supports expression languages like SpEL and MVEL. Here is an example for EasyRule:
The Maven Dependency;
<dependency>
<groupId>org.jeasy</groupId>
<artifactId>easy-rules-core</artifactId>
<version>3.3.0</version>
</dependency>
The example can be:
@Rule(name = "Delftstack rule", description = "Always say Delftstack The Best Tutorial Site")
public class DelftstackRule {
@Condition
public boolean when() {
return true;
}
@Action
public void then() throws Exception {
System.out.println("Delftstack The Best Tutorial Site");
}
}
The code above creates a rule that every time it prints Delftstack The Best Tutorial Site
. To launch this code use the code below with the main function:
public class Launch {
public static void main(String... args) {
Facts facts = new Facts();
// create rules
Rules rules = new Rules();
rules.register(new DelftstackRule());
// create a rules engine and fire rules on known facts
RulesEngine Rules_Engine = new DefaultRulesEngine();
Rules_Engine.fire(rules, facts);
}
}
OpenL Tablets
The OpenL Tablets
is a business rule engine and a business rule management system. It contains Webstudio, Web services, rule repository, and Business Rules Engines.
Let’s see an example:
The Maven Dependency for OpenL Tablets
:
<dependency>
<groupId>org.openl</groupId>
<artifactId>org.openl.core</artifactId>
<version>${version}</version>
</dependency>
<dependency>
<groupId>org.openl.rules</groupId>
<artifactId>org.openl.rules</artifactId>
<version>${version}</version>
</dependency>
The example can be:
public class Main {
private DelftstackRules instance;
public static void main(String[] args) {
Main rules = new Main();
rules.process(aCase);
}
public void process(Case aCase) {
EngineFactory Engine_Factory = new RulesEngineFactory(
getClass().getClassLoader().getResource("rules.xls"), DelftstackRules.class);
instance = Engine_Factory.newEngineInstance();
instance.executePromotion(aCase, new Response());
}
}
The code above creates the delftstack rules from the Xls file.
These were a few popular rule engines. To download or for more info, please visit the links below:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook