How to Create a Range in Java
- Create and Check Whether a Number Is in the Range in Java
- Create a Range Using Guava Range in Java
- Create a Range Using Apache Range in Java
- Create a Range Using ValueRange in Java
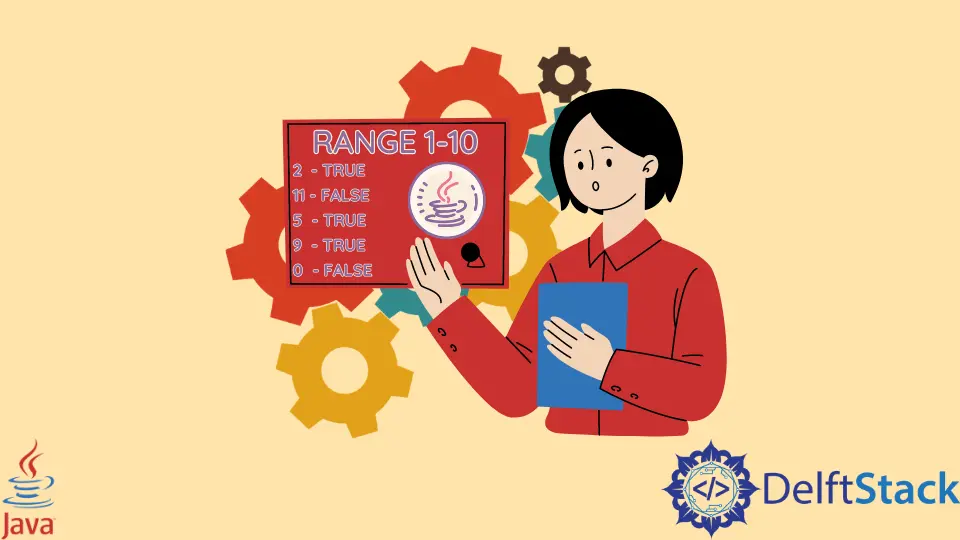
Various libraries in Java can create a range and check if a given number exists between the created range. We can also use the traditional ways in Java to check if a given number is contained between a minimum and maximum value of the range. Let’s use third-party libraries as well as traditional methods to achieve this task.
Create and Check Whether a Number Is in the Range in Java
This is the traditional way to check whether the number lies in the range. We pass as an argument. Here, we created a class, InRange
, which has a default constructor to initialize passed arguments to the member variables. It creates a range and checks if the number is within the range.
We create an instance of the InRange
class using the new
keyword and pass the lower and higher value of the range in the default constructor. Now we check if number 50
falls within 10
and 99
.
We call the containsInRange()
method, which returns a boolean checking if the number is greater than or equal to the lower range and smaller than or equal to the higher range using number >= lowerRange && number <= higherRange
statement. Thus, 50
falls in the given range, so true
is returned as output.
import org.w3c.dom.ranges.Range;
class InRange {
private int lowerRange;
private int higherRange;
public InRange(int low, int high) {
lowerRange = low;
higherRange = high;
}
public boolean containsInRange(int number) {
return (number >= lowerRange && number <= higherRange);
}
}
public class TestRange {
public static void main(String[] args) {
InRange inRange = new InRange(10, 99);
int number = 50;
System.out.println(
"Is number " + number + " in our range : " + inRange.containsInRange(number));
}
}
Output:
Is number 50 in our range : true
Create a Range Using Guava Range in Java
This is a third-party library. Guava Range
represents an interval with endpoints a
and b
, which form the boundary. We will check if a number falls in between a
and b
.
The maven dependency required for this library is given below.
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>29.0-jre</version>
</dependency>
We create a range
instance of this type using standard factory methods. The range created may be open or closed based on exclusive or inclusive endpoints.
The method open(a,b)
creates and returns a range that represents a < range < b. That means a
and b
are excluded. Whereas for the method closed(a, b
) the range is a
<= range <=b
, hence a
and b
are included.
The contains()
method returns true if the passed argument lies within the given range. For example, if we check whether instance range
contains number 8
using the contains()
method it returns false as it’s an exclusive range.
But at the same time calling the method contains(8)
on the range1
instance returns true for the same argument as it is an inclusive range. We can see this in the output.
import com.google.common.collect.Range;
public class TestRange {
public static void main(String[] args) {
Range<Integer> range = Range.open(1, 8);
Range<Integer> range1 = Range.closed(1, 8);
System.out.println(range.contains(3));
System.out.println(range.contains(8));
System.out.println(range1.contains(8));
}
}
Output:
true
false
true
Create a Range Using Apache Range in Java
Apache Range
is another third-party library to check if a number lies within the range. The maven dependency for this library is given below.
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.0.1</version>
</dependency>
Apache Range
class represents a range of values from a minimum to a maximum value (inclusive). We created a testRange
instance using the factory method between()
that represents a range from 10
to 34
i.e range includes these two values as well.
The contains()
method checks if a specified element is present within the range. It returns true if the range contains the element.
import org.apache.commons.lang3.Range;
public class TestRange {
public static void main(String[] args) {
Range<Integer> testRange = Range.between(10, 34);
System.out.println(testRange.c(2));
System.out.println(testRange.contains(32));
}
}
Output:
false
true
Create a Range Using ValueRange in Java
We can also use Java 8 ValueRange
, which creates a range inclusive of both minimum and maximum value. The isValidIntValue
method checks if the passed parameter lies within the valid range. It also validates if all values in the range fit in an int
.
import java.time.temporal.ValueRange;
public class TestRange {
public static void main(String[] args) {
ValueRange range = ValueRange.of(0, 2);
System.out.println(range.isValidIntValue(8));
}
}
Output:
false
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn