Properties File in Java
- Create and Set Values in Java Properties File
- Get a Property From the Java Properties File
- Get All the Keys and Values From the Java Properties File
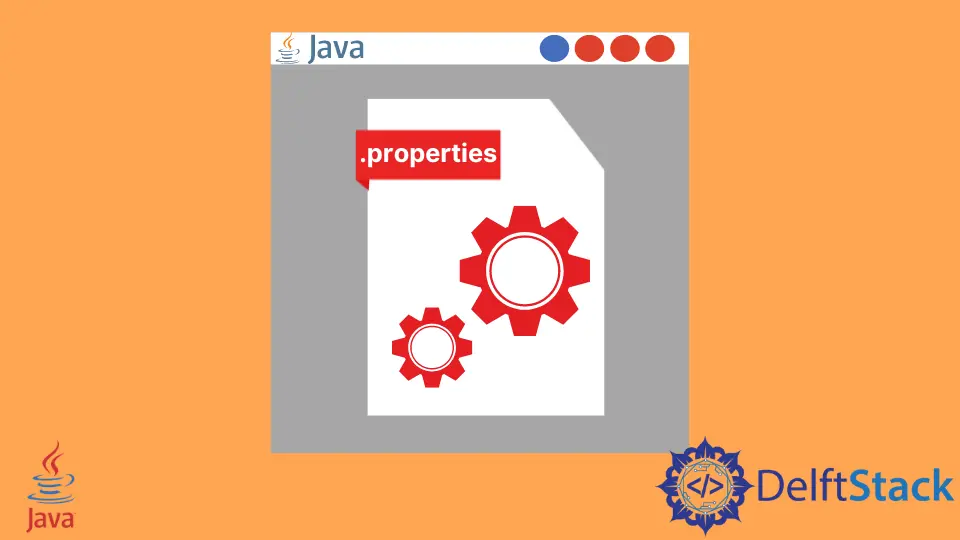
Properties in Java are configuration values that we need to use in Java applications.
A Properties file is a file that contains these values in key-value pairs. This type of file usually has a .properties
extension.
Create and Set Values in Java Properties File
In the first section of this tutorial, we write a program that creates a properties file and then sets the properties as key-value pairs.
Java provides us with a Properties
class of the java.util
package containing several methods to perform operations like setting or removing properties, listing all properties, etc.
Before we proceed to set the properties, we need to get the file properties from the directory where it is located, and if it does not exist, we create a new file. We create an object of FileOutputStream()
and pass the properties file’s path in its constructor that returns an OutputStream
instance.
We create a Properties
object using new Properties()
and call the setProperty()
method to set the property values in the file. The setProperty()
method takes two arguments, the first is the key, and the second is the value.
In the example, we set three properties and finally store the properties in the file we call the store()
method to pass the outputStream
and an optional comment as arguments. The properties file should be created and have the properties inserted in it.
import java.io.*;
import java.util.Properties;
public class JavaExample {
public static void main(String[] args) {
try {
OutputStream outputStream = new FileOutputStream("myConfigs.properties");
Properties properties = new Properties();
properties.setProperty("version", "1.1");
properties.setProperty("projectName", "PropertiesFileExample");
properties.setProperty("date", "2021/12/27");
properties.store(outputStream, "Properties are stored here");
System.out.println(properties);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
{date=2021/12/27, projectName=PropertiesFileExample, version=1.1}
myConfigs.properties
file:
#Properties are stored here
#Mon Dec 27 16 : 52 : 38 IST 2021
date = 2021 / 12 / 27 projectName = PropertiesFileExample version = 1.1
Get a Property From the Java Properties File
This section gives a specific property from the properties file using the Properties
class and its methods. Unlike the previous example, we use InputStream
instead of OutputStream
because we read the file in this program.
We create an object of the Properties
class, call the load()
method, and pass the InputStream
object as its argument. Once the file is loaded, we call the getProperty()
function and pass the key of the value that we want to fetch.
We get the value as a result that we print in the output.
import java.io.*;
import java.util.Properties;
public class JavaExample {
public static void main(String[] args) {
try {
InputStream inputStream = new FileInputStream("myConfigs.properties");
Properties properties = new Properties();
properties.load(inputStream);
System.out.println(properties.getProperty("date"));
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
2021/12/27
Get All the Keys and Values From the Java Properties File
list()
is a function of the Properties
class that prints the properties to an output stream. We read the properties file in the program, create a Properties
object, and load the file using the load()
function.
To print the properties, we call the list()
method and pass System.out
as an argument that is an output stream that prints on the console.
import java.io.*;
import java.util.Properties;
public class JavaExample {
public static void main(String[] args) {
try {
InputStream inputStream = new FileInputStream("myConfigs.properties");
Properties properties = new Properties();
properties.load(inputStream);
properties.list(System.out);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
-- listing properties --
date=2021/12/27
projectName=PropertiesFileExample
version=1.1
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn