How to Overwrite a File in Java
-
Overwrite a File in Java Using
FileWriter
-
Overwrite a File in Java Using
Files.write
-
Overwrite a File in Java Using
PrintWriter
-
Overwrite a File in Java Using
FileOutputStream
- Conclusion
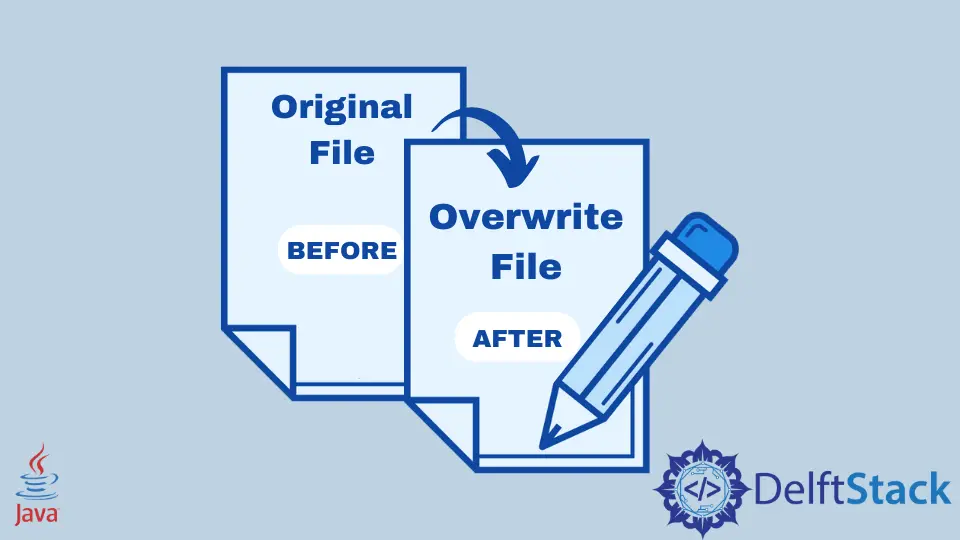
Overwriting a file is a fundamental operation in file handling, often required when updating or modifying the contents of an existing file.
This article will guide you through the process of overwriting a file in Java, providing a detailed and comprehensive explanation of the provided code.
Overwrite a File in Java Using FileWriter
Overwriting a text file is an easy operation in Java. You can try it by following the step-by-step process below.
-
First, we delete the file you want to overwrite.
-
We then create a new file with the same name.
-
Next, we write the new content in the new file using
FileWriter
.- We create a
FileWriter
instance calledOverwritten_File
and pass theNew_File
along withfalse
to its constructor. Thefalse
argument indicates that we want to overwrite the file if it already exists. - We use the
write
method to write theOverwritten_Content
to the file, effectively overwriting any existing content. - The
close
method is called to close theFileWriter
, ensuring that any buffered data is written to the file.
- We create a
Let’s try an example:
package delftstack;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
public class Overwrite_File {
public static void main(String[] args) {
File Old_File = new File("delftstack.txt");
Old_File.delete();
File New_File = new File("delftstack.txt");
String Overwritten_Content =
"Hello, This is a new text file from delftstack.com after overwriting the previous file.";
System.out.println(Overwritten_Content);
try {
FileWriter Overwritten_File = new FileWriter(New_File, false);
Overwritten_File.write(Overwritten_Content);
Overwritten_File.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
The code above will overwrite a file in Java. The previous file has the text:
Hello, This is a text file from delftstack.com before overwriting the file.
After running the code, the output will be the following.
Hello, This is a new text file from delftstack.com after overwriting the previous file.
Overwrite a File in Java Using Files.write
You can also use the Files.write
method from the java.nio.file
package to write content to a file and overwrite its existing content.
Let’s see the detailed steps to understand how the file overwriting process works.
-
We begin by importing the required packages:
java.io.IOException
for handling input/output exceptions andjava.nio.file.*
to work with file-related operations. -
We then specify the file path where we want to overwrite the file and define the new content that will replace the existing content in the file.
-
We use a
try-catch
block to handle potential exceptions that may occur during the file overwriting process. Inside thetry
block:- We create a
Path
instance by callingPaths.get(filePath)
to represent the file at the specified path. - We use
Files.write
to write the new content (overwrittenContent
) to the file represented by thePath
, but if the file already exists, this will overwrite its content. TheArrays.asList
method is used to convert the content into a list of strings. - We print a success message to indicate that the file has been overwritten successfully.
- We create a
Below is the complete working Java code that demonstrates how to overwrite a file using the Files.write
method:
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Arrays;
public class OverwriteFileUsingFilesWrite {
public static void main(String[] args) {
String filePath = "delftstack.txt";
String overwrittenContent =
"Hello, This is a new text file from delftstack.com after overwriting the previous file.";
try {
Path path = Paths.get(filePath);
Files.write(path, Arrays.asList(overwrittenContent));
System.out.println("File overwritten successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Let’s break down the code into detailed steps to understand how the file overwriting process works using FileOutputStream
.
-
We begin by importing the necessary package,
java.io
, which provides the classes for input/output operations. -
We then define the file path and the new content that will overwrite the existing file.
-
We convert the content to bytes and write it to the specified file.
- We convert the
overwrittenContent
string to bytes using thegetBytes
method. This is necessary becauseFileOutputStream
writes data in the form of bytes. - We create a
FileOutputStream
instance calledoutputStream
and pass the file path to its constructor. This allows theFileOutputStream
to write to the specified file. - We use the
write
method to write the content bytes to the file, effectively overwriting any existing content. - The
close
method is called to close theFileOutputStream
, ensuring that any buffered data is written to the file and resources are released. - If an
IOException
occurs, we catch it and print the stack trace to help with debugging.
- We convert the
After executing the code, we will have the following overwritten file as output:
Conclusion
These methods provide alternative ways to overwrite a file in Java. Depending on the specific use case or preferences, you can choose the method that best suits your needs.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook