How to Create a Mouse Listener in Java
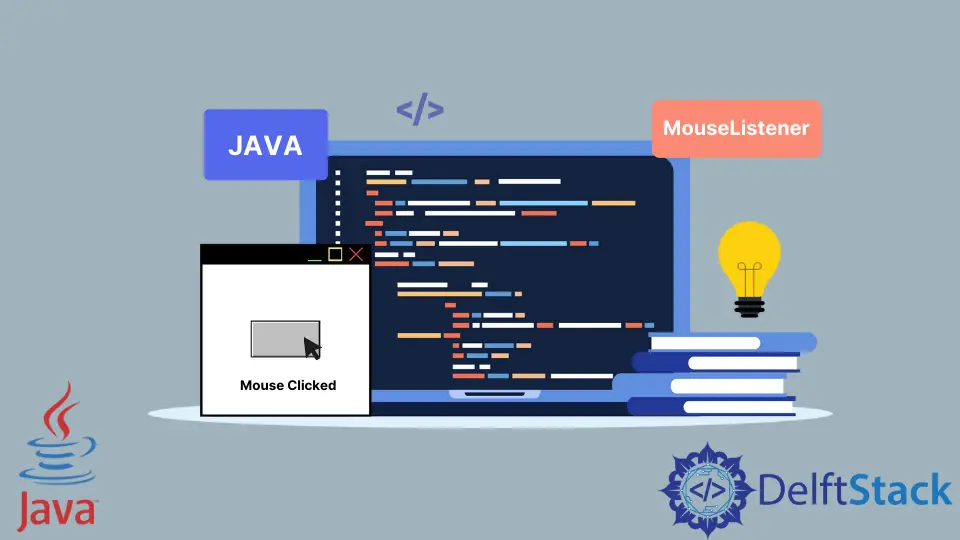
In Java, the MouseListener
class is notified when the mouse moves in a particular space.
This tutorial demonstrates how to create a MouseListener
interface.
Create a Mouse Listener in Java
MouseListener
gets notified when there is a state change. This change can be clicking, pressing, and releasing the mouse or entering and exiting a particular window area.
The MouseListener
is a part of the Java.awt.event
package.
The step by step mechanism of Java MouseListener
is given below:
- When we click or move the mouse, an object in the program helps implement the interface.
- That object is already registered with the listener. That is why an event is generated by pressing, clicking, or moving the mouse.
- When the event is generated, it helps in the invocation of one of the methods.
- The
Mouse
event is passed to the method after this invocation.
The MouseListener
has several methods which are invocated on different events. These methods are given in the table.
S.No | Method | Description |
---|---|---|
1 | void mouseClicked(MouseEvent e) |
This method is invoked when clicking the mouse - pressing and releasing. |
2 | void mouseEntered(MouseEvent e) |
This method is invoked when the mouse is moved and enters a component. |
3 | void mouseExited(MouseEvent e) |
This method is invoked when the mouse is moved and exits from a component. |
4 | void mousePressed(MouseEvent e) |
This method is invoked when the mouse button is pressed only on a component. |
5 | void mouseReleased(MouseEvent e) |
This method is invoked when the mouse button is released from a component. |
Now, let us try an example that will invoke all the methods described in the table.
Example:
package delftstack;
import java.awt.FlowLayout;
import java.awt.Frame;
import java.awt.Label;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
// The main class implements the MouseListener interface
public class Mouse_Listener implements MouseListener {
// create two labels one frame and one string
Label label1, label2;
Frame Demo_Frame;
String event_invo;
// Constructor
Mouse_Listener() {
Demo_Frame = new Frame("The Mouse Listener");
label1 = new Label("This is demo for the mouse listener events\n", Label.CENTER);
label2 = new Label();
// set the frame layout as flowlayout
Demo_Frame.setLayout(new FlowLayout());
// add labels to the frame
Demo_Frame.add(label1);
Demo_Frame.add(label2);
// Register the created class Mouse_Listener to catch as well as respond to the mouse events,
Demo_Frame.addMouseListener(this);
// set the size of frame 450x300
Demo_Frame.setSize(450, 300);
// visibility of the frame will be true
Demo_Frame.setVisible(true);
}
// mouseClicked method
public void mouseClicked(MouseEvent ev) {
event_invo += " The mouse button was clicked";
// set the text of label 2 as even_invo
label2.setText(event_invo);
// visibility will be true
Demo_Frame.setVisible(true);
}
// mouseEntered method
public void mouseEntered(MouseEvent ev) {
// when mouse enters the window
label2.setText("The mouse has entered the window");
// visibility will be true
Demo_Frame.setVisible(true);
}
// mouseExited method
public void mouseExited(MouseEvent ev) {
// when the mouse leaves the window
label2.setText("Mouse has left the window");
// visibility as true
Demo_Frame.setVisible(true);
}
// mousePressed method
public void mousePressed(MouseEvent ev) {
// when the mouse is pressed
label2.setText("Mouse button is pressed");
// set the visibility as true
Demo_Frame.setVisible(true);
}
// mouseReleased method
public void mouseReleased(MouseEvent ev) {
// set the string event_invo
event_invo = "The mouse button is released ";
label2.setText(event_invo);
// visibility as true
Demo_Frame.setVisible(true);
}
// main method
public static void main(String args[]) {
new Mouse_Listener();
}
}
Output:
The code above implements all the methods described above, which means the window will know when the mouse enters, exists, presses, releases, or clicks.
MouseListener
is usually used with Java swing to create an interface.
For example, we need to get the click position’s X
and Y
points. We can do it by combining MouseListener
and Swing
.
Let us implement this example.
package delftstack;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Mouse_Listener {
private JFrame Demo_Frame;
private JLabel Label_One;
private JLabel Label_Two;
private JPanel Control_Panel;
public Mouse_Listener() {
Create_GUI();
}
public static void main(String[] args) {
Mouse_Listener MouseListenerDemo = new Mouse_Listener();
MouseListenerDemo.Show_Mouse_Listener();
}
private void Create_GUI() {
Demo_Frame = new JFrame("Java SWING With MouseListener");
Demo_Frame.setSize(400, 400);
Demo_Frame.setLayout(new GridLayout(3, 3));
Label_One = new JLabel("", JLabel.CENTER);
Label_Two = new JLabel("", JLabel.CENTER);
Label_Two.setSize(350, 350);
Demo_Frame.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent windowEvent) {
System.exit(0);
}
});
Control_Panel = new JPanel();
Control_Panel.setLayout(new FlowLayout());
Demo_Frame.add(Label_One);
Demo_Frame.add(Control_Panel);
Demo_Frame.add(Label_Two);
Demo_Frame.setVisible(true);
}
private void Show_Mouse_Listener() {
Label_One.setText("X Y Points for MouseListener");
JPanel Demo_Panel = new JPanel();
Demo_Panel.setBackground(Color.green);
Demo_Panel.setLayout(new FlowLayout());
Demo_Panel.addMouseListener(new CustomMouseListener());
JLabel Msg_Label = new JLabel("Welcome to Delftstack.com", JLabel.CENTER);
Demo_Panel.add(Msg_Label);
Msg_Label.addMouseListener(new CustomMouseListener());
Demo_Panel.add(Msg_Label);
Control_Panel.add(Demo_Panel);
Demo_Frame.setVisible(true);
}
class CustomMouseListener implements MouseListener {
public void mouseClicked(MouseEvent e) {
Label_Two.setText("Mouse Clicked: (" + e.getX() + ", " + e.getY() + ")");
}
public void mousePressed(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
}
}
Output:
The code above will get the x
and y
points for the click position on a particular area. The code uses Java Swing
and MouseListener
.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook