How to Create Makefile in Java
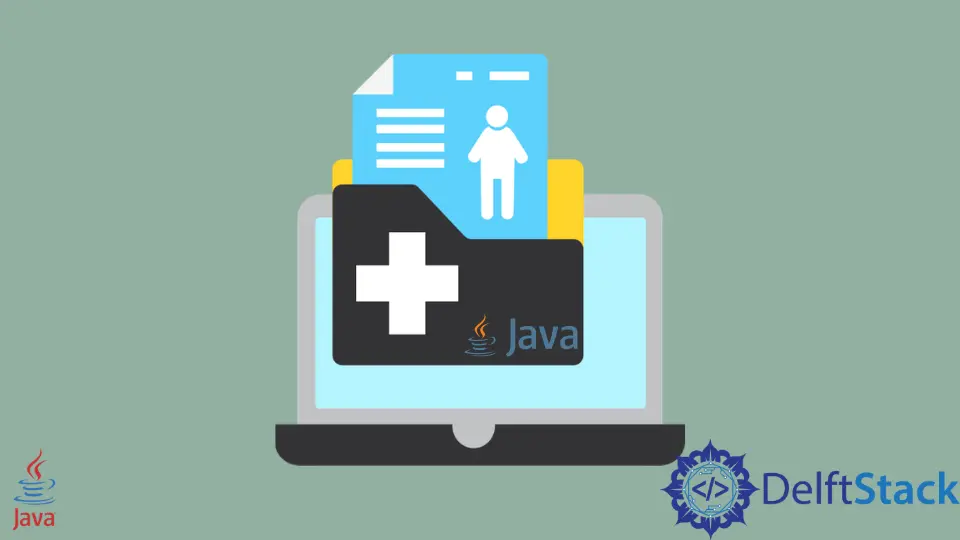
A Makefile
is a specially formatted file required by the make utility when used.
When we write programs for a large project, we might need to compile every program manually, which can be time-consuming, and this is where a makefile can make this task easy and fast.
Use the Makefile
to Manage Large Projects in Java
A Makefile is a way to manage large project files by compiling and linking them. A simple makefile contains variables and rules.
Below is a basic syntax for creating a rule.
target... : prerequisites...
<TAB>command...
In the above syntax, a target
is the program’s name to be generated. The prerequisites or dependencies are the files used to create the target.
We write the commands in command
that begins with a tab
. We can use variables in a makefile to store text strings to remove duplicate usage of commands and rules.
Compiling a project with several files is a time-taking task, and we have to repeat the same commands whenever we make any changes in the files.
This time can be saved using a makefile to compile the Java files whenever there is a change. Note that when a modification occurs in a single file, makefile compiles only the changed file instead of compiling every file.
Use the Makefile
in Java With an Example
We have three Java files for the example. The first Java file has three classes, while the two files contain single classes.
To compile all the files, we need to use the javac
command every time, but we use the makefile to compile these files.
In the following makefile, we first specify the compiler to use, javac
, and store it in a variable JC
. Next, specify the suffixes to use.
Now we create a target using the syntax we learned above, .java.class
is the target while $(JC) $*.java
is the command to execute to create the target.
We create another macro that contains all the Java files that we want to compile when the makefile is executed. Makefiles understand only tab space, or we can break the statement using the \
operator.
We specify the default
target with the CLASSES
macro. In the next statement, we create another target classes
that replaces the files containing .java
with .class
and store it in CLASSES
.
The last target is to clean the class files using the command $(RM) *.class
.
JC =javac
.SUFFIXES:.java .class
.java.class:
$(JC) $*.java
CLASSES = \
JavaExample.java\
JavaExample2.java\
JavaExample3.java
default:CLASSES
classes:$(CLASSES:.java=.class)
clean:\
$(RM) *.class
To execute the makefile, we use the following command in the command line.
make -f makefile
Here, make
is the utility command, while -f
is an argument for file and makefile
is the file name of our makefile.
When we execute the command, we get the below output.
javac JavaExample.java
javac JavaExample2.java
javac JavaExample3.java
After this, the class files are generated when there are changes, and we run the command,
Java Example Class:
public class JavaExample {
public static void main(String args[]) {
Motorcycle motorcycle = new Motorcycle();
System.out.println(motorcycle.getClassName());
}
}
class Vehicle {
String getClassName() {
return "Vehicle Class";
}
}
class Motorcycle extends Vehicle {
String getClassName() {
return "Motorcycle Class Child of " + super.getClassName();
}
}
Output:
Motorcycle Class Child of Vehicle Class
Java Example 2 Class:
public class JavaExample2 {
public static void main(String[] args) {
System.out.println("Java Example 2");
}
}
Output:
Java Example 2
Java Example 3 Class:
public class JavaExample3 {
public static void main(String[] args) {
System.out.println("Java Example 3");
}
}
Output:
Java Example 3
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn