How to Use Keycode in Java
-
Using
KeyListener
to Listen to Key Codes in Java -
Print Key Codes and Their Text Description Using
KeyEvent.getKeyText()
in Java
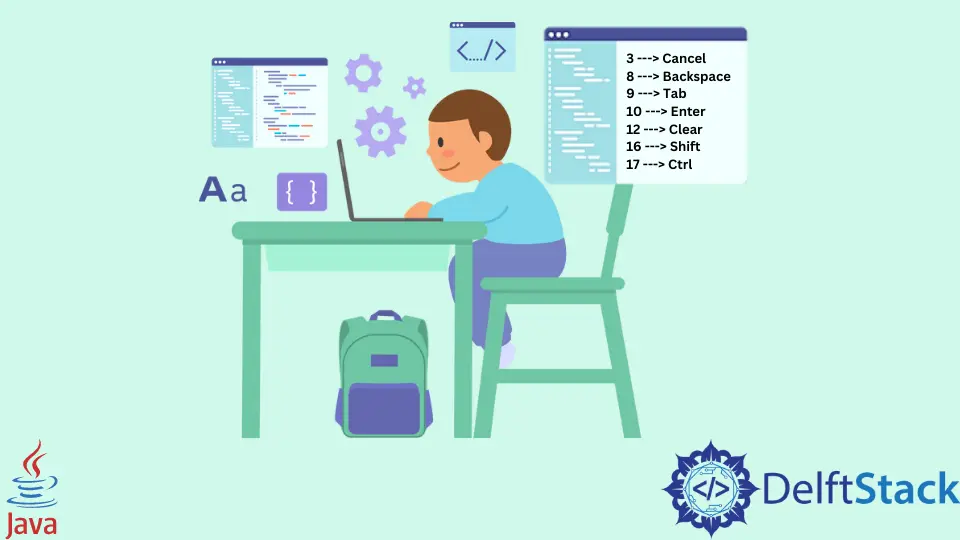
Today we will be looking at the key codes that represent the keys on our keyboard. A key code is an integer code assigned to a KeyEvent
object.
Using KeyListener
to Listen to Key Codes in Java
In this example, we create a frame and add an input field with two labels at its bottom. When we enter any key on our keyboard in the field, the labels will give some information about the keys.
In the program below, we create a class JavaKeycodeExample
that extends the Frame
class and implements the KeyListener
interface. The Frame
class provides us with a window to place the input field and the labels, while with the help of the KeyListener
interface, we can override its three methods that help us identify the key events.
In the JavaKeycodeExample
class, we create two labels, label1
and label2
, and a text field textField
that takes the input. We initialize and set the size of the AWT components to add them to the Frame
.
We add a KeyListener
to the textField
using addKeyListener()
. We implemented the KeyListener
interface, so we have to override its three methods that are keyPressed()
that records the key pressed, keyTyped()
that identifies the typed key, and the last method keyReleased()
invokes when the key that was pressed is released.
For this example, we mainly use the keyPressed()
method, which receives a KeyEvent
object e
whenever a key is pressed in the input field. To get the key code pressed, we call the getKeyCode()
method using the KeyEvent
object and concatenate the result with a string.
We also get the text that represents the key code using the method KeyEvent.getKeyText()
and pass e.getKeyCode()
. At last, we set both the strings to the labels label1
and label2
.
In the output, we can observe that we pressed the key k
on the keyboard in the text field; the first label prints the key code while the second label shows the key text.
import java.awt.*;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
public class JavaKeycodeExample extends Frame implements KeyListener {
Label label1;
Label label2;
TextField textField;
public JavaKeycodeExample() {
label1 = new Label();
label1.setBounds(120, 220, 200, 20);
label2 = new Label();
label2.setBounds(120, 250, 200, 20);
textField = new TextField();
textField.setBounds(100, 100, 200, 100);
textField.addKeyListener(this);
add(label1);
add(label2);
add(textField);
setSize(350, 350);
setLayout(null);
setVisible(true);
}
public void keyPressed(KeyEvent e) {
String keyCodeString = "Last Key Code Pressed : " + e.getKeyCode();
String keyCodeAsTextString = "You pressed : " + KeyEvent.getKeyText(e.getKeyCode());
label1.setText(keyCodeString);
label2.setText(keyCodeAsTextString);
}
@Override
public void keyTyped(KeyEvent e) {}
@Override
public void keyReleased(KeyEvent e) {}
public static void main(String[] args) {
new JavaKeycodeExample();
}
}
Output:
Print Key Codes and Their Text Description Using KeyEvent.getKeyText()
in Java
There are a lot of key codes representing the keys on the keyboard, and in this program, we print some of them. We get the key code and the key text that describes it using a for
loop that starts from 0
and runs until 50
.
In the loop, where every value of the loop may be a key code, we get the key text using the function KeyEvent.getKeyText()
and pass the key code value.
Note that not every integer value represents a key code and might return a key text of Unknown keyCode
; thus, we check if the string keyCodeText
contains the text or not. If it is a valid key code, it prints both the key code and the key code text.
In the output, we observe that the left side of the arrow is the keycode and the right side of the arrow is the text description of the key code.
import java.awt.event.KeyEvent;
public class JavaKeycodeExample {
public static void main(String[] args) {
for (int keyCode = 0; keyCode < 50; ++keyCode) {
String keyCodeText = KeyEvent.getKeyText(keyCode);
if (!keyCodeText.contains("Unknown keyCode")) {
System.out.println(keyCode + " ---> " + keyCodeText);
}
}
}
}
8 ---> Backspace
9 ---> Tab
10 ---> Enter
12 ---> Clear
16 ---> Shift
17 ---> Ctrl
18 ---> Alt
19 ---> Pause
20 ---> Caps Lock
21 ---> Kana
24 ---> Final
25 ---> Kanji
27 ---> Escape
28 ---> Convert
29 ---> No Convert
30 ---> Accept
31 ---> Mode Change
32 ---> Space
33 ---> Page Up
34 ---> Page Down
35 ---> End
36 ---> Home
37 ---> Left
38 ---> Up
39 ---> Right
40 ---> Down
44 ---> Comma
45 ---> Minus
46 ---> Period
47 ---> Slash
48 ---> 0
49 ---> 1
–>
Output:
3 ---> Cancel
8 ---> Backspace
9 ---> Tab
10 ---> Enter
12 ---> Clear
16 ---> Shift
17 ---> Ctrl
18 ---> Alt
19 ---> Pause
20 ---> Caps Lock
21 ---> Kana
24 ---> Final
25 ---> Kanji
27 ---> Escape
28 ---> Convert
29 ---> No Convert
30 ---> Accept
31 ---> Mode Change
32 ---> Space
33 ---> Page Up
34 ---> Page Down
35 ---> End
36 ---> Home
37 ---> Left
38 ---> Up
39 ---> Right
40 ---> Down
44 ---> Comma
45 ---> Minus
46 ---> Period
47 ---> Slash
48 ---> 0
49 ---> 1
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn