In-Memory Cache in Java
- Use the Guava Library for In-Memory Cache in Java
- Use the EhCache Library for In-Memory Cache in Java
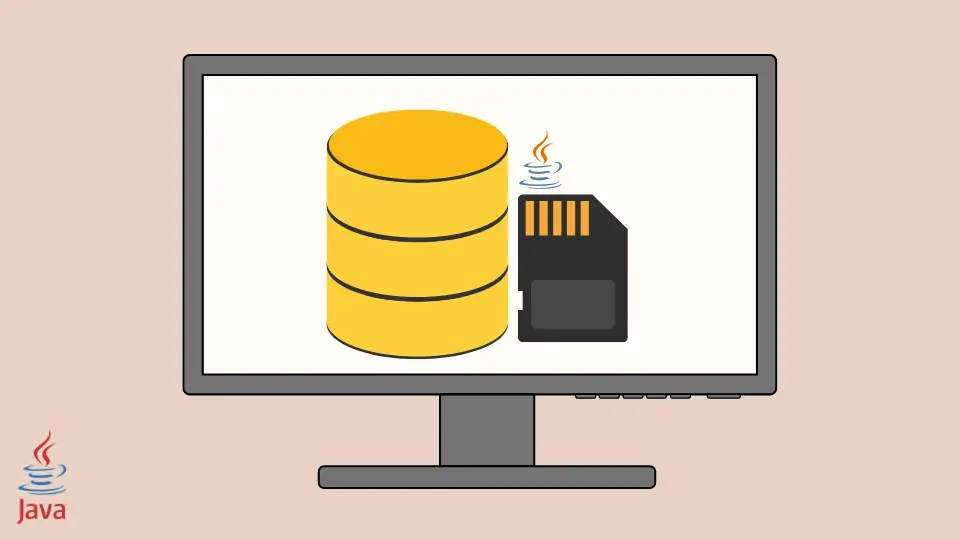
This tutorial will discuss the two libraries we can use for in-memory cache in Java.
Use the Guava Library for In-Memory Cache in Java
When fetching data from an API or database, and we want to access it frequently, fetching the same data whenever needed can be expensive. Caching becomes useful since it saves the often demanded data to access later readily.
To include the Guava library in our project, we use the following Maven dependency.
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>31.0.1-jre</version>
</dependency>
Example:
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
public class ExampleClass1 {
private final LoadingCache<String, String> loadingCache;
public ExampleClass1() {
final CacheLoader<String, String> loader = new CacheLoader<>() {
@Override
public String load(String key) {
return key.toUpperCase();
}
};
loadingCache = CacheBuilder.newBuilder()
.maximumSize(1500)
.expireAfterAccess(15, TimeUnit.MINUTES)
.build(loader);
}
public static void main(String[] args) throws ExecutionException {
ExampleClass1 cacheExample = new ExampleClass1();
cacheExample.loadingCache.put("key1", "value1");
cacheExample.loadingCache.put("key2", "value2");
System.out.println("Whole cache as map: " + cacheExample.loadingCache.asMap());
System.out.println("Get a single value using a key: " + cacheExample.loadingCache.get("key1"));
}
}
Output:
Whole cache as map: {key1=value1, key2=value2}
Get a single value using key: value1
We created an instance of LoadingCache
with the type parameters for the key and value in the code above. Inside the constructor, we override the load()
function of the CacheLoader
class that computes and retrieves the values.
We call the CacheBuilder
, a builder for LoadingCache
. We also call the newBuilder()
method. Set the maximum number of values the cache can contain and the expiration time of the cache to 15 minutes after accessing it.
Then we call the build()
method with the loader
instance. To insert and fetch the data from the cache, we create an object of the ExampleClass1
in the main()
method and call the put()
method to insert the values in a key-value pair format.
We can get the whole cache as a map using the asMap()
method, and to get a single value from its key, we use the get()
function with the key as its argument.
Use the EhCache Library for In-Memory Cache in Java
For this example, we use another caching library called EhCache that we can include in the project using the following Maven dependency.
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.9.6</version>
</dependency>
Example:
import org.ehcache.Cache;
import org.ehcache.CacheManager;
import org.ehcache.config.builders.CacheConfigurationBuilder;
import org.ehcache.config.builders.CacheManagerBuilder;
import org.ehcache.config.builders.ResourcePoolsBuilder;
public class ExampleClass1 {
public static void main(String[] args) {
CacheManager cacheManager = CacheManagerBuilder.newCacheManagerBuilder()
.withCache("exampleCache",
CacheConfigurationBuilder
.newCacheConfigurationBuilder(String.class,
String.class, ResourcePoolsBuilder.heap(50))
.build())
.build(true);
Cache<String, String> cache = cacheManager.getCache("exampleCache", String.class, String.class);
cache.put("First Name", "Sam");
cache.put("Last Name", "Maxwell");
String getFirstNameFromCache = cache.get("First Name");
String getLastNameFromCache = cache.get("Last Name");
System.out.println("First Name: " + getFirstNameFromCache);
System.out.println("Last Name: " + getLastNameFromCache);
cacheManager.close();
}
}
Output:
[main] INFO org.ehcache.core.EhcacheManager - Cache 'exampleCache' created in EhcacheManager.
First Name: Sam
Last Name: Maxwell
[main] INFO org.ehcache.core.EhcacheManager - Cache 'exampleCache' removed from EhcacheManager.
As we can see, we call the newCacheManagerBuilder()
method of the CacheManagerBuilder
class. Then we call the withCache()
method arguments; the first argument is an alias for the cache, and the second is the configuration for the cache of type CacheConfiguration
.
We configure the cache using the CacheConfigurationBuilder.newCacheConfigurationBuilder()
method in which we pass the type of the key and value. Then we pass the heap size called its build()
method.
Finally, we call the build()
method of the CacheManagerBuilder
class and pass true as its argument returns an instance of CacheManager
.
Once the manager is set, we call the getCache()
method and pass in the alias and the types of key-value to fetch the cache that returns Cache
with the type parameters. We call the put()
method to insert values to the cache in key-value pair using the cache object.
We fetch the values from the cache using the get()
method. At last, we close the cacheManager
to complete the stream.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn