ImageIO in Java
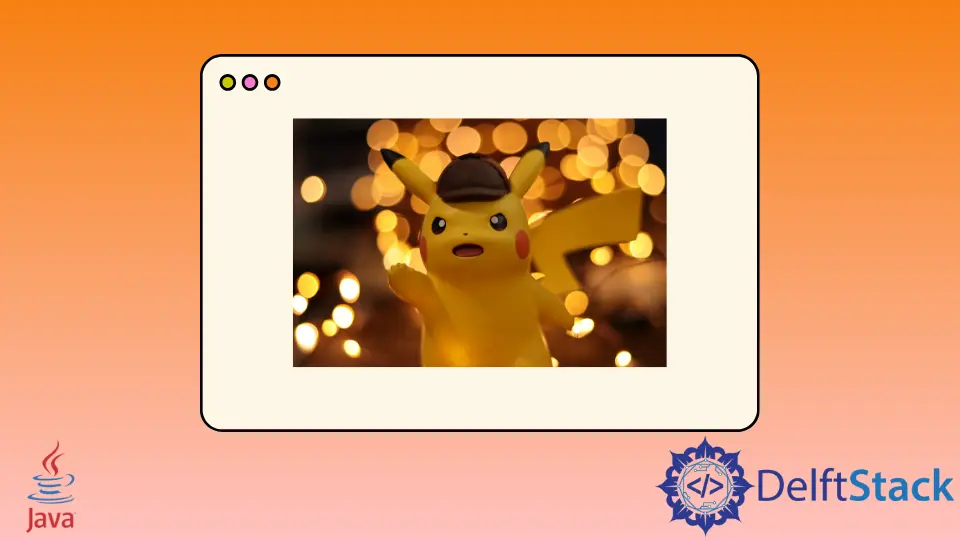
ImageIO
is a class in Java, a part of the javax.imageio
package containing various methods to perform operations like reading or writing an image.
We will learn to use the ImageIO
class to read and write an image in the following sections.
Use ImageIO
to Read an Image in Java
We use the read()
function of the ImageIO
class to read an image. In the program, we create an object of File
and pass the file’s path as an argument in the constructor.
We call the read()
method using ImageIO
and pass it in the file
object that returns the BufferedImage
object. We have successfully read the image, and we use a JFrame
and ImageIcon
to display the image in a window.
We create a function setImageToFrame()
and send the bufferedImage
as argument. In the setImageToFrame()
function, we create an object of ImageIcon
and set it as the icon of JLabel
using the setIcon()
function.
Once we add the label to the frame and run the program, we can see the image in the output.
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.*;
public class JavaExample {
public static void main(String[] args) {
try {
File file = new File(
"C:\\Users\\Rupam Saini\\IdeaProjects\\Java Examples\\src\\main\\java\\sample_image.jpg");
BufferedImage bufferedImage = ImageIO.read(file);
setImageToFrame(bufferedImage);
} catch (IOException e) {
e.printStackTrace();
}
}
static void setImageToFrame(BufferedImage image) {
ImageIcon imageIcon = new ImageIcon(image);
JFrame jFrame = new JFrame();
jFrame.setLayout(new FlowLayout());
jFrame.setSize(700, 500);
JLabel jLabel = new JLabel();
jLabel.setIcon(imageIcon);
jFrame.add(jLabel);
jFrame.setVisible(true);
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
Output:
We can also use the read()
function to read an image from a link.
We need to create a URL
object and use the link to the image as the argument constructor. We pass the URL
object to the read()
method.
URL url = new URL(
"https://images.unsplash.com/photo-1516475429286-465d815a0df7?ixlib=rb-1.2.1&q=80&fm=jpg&crop=entropy&cs=tinysrgb&dl=will-klinzman-oaCD9WYdNlU-unsplash.jpg&w=640");
BufferedImage bufferedImage = ImageIO.read(url);
Output:
Use ImageIO
to Write an Image and Change Format in Java
Another useful operation we can do using the ImageIO
class is to write an image and change its format. We first read the image as we do in the previous example and then call the write()
function of the ImageIO
class that takes three arguments.
The first argument of the write()
method is the BufferedImage
object. The second argument is the format or extension in which we want to write the new image. The last argument is the File
object that has the path to the new image with the new extension.
In our example, we change our image’s format from JPG
to PNG
. We have two images: the original image and the other image we created using the write()
method.
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class JavaExample {
public static void main(String[] args) {
try {
File file = new File(
"C:\\Users\\Rupam Saini\\IdeaProjects\\Java Examples\\src\\main\\java\\sample_image.jpg");
BufferedImage bufferedImage = ImageIO.read(file);
ImageIO.write(bufferedImage, "png",
new File(
"C:\\Users\\Rupam Saini\\IdeaProjects\\Java Examples\\src\\main\\java\\sample_image.png"));
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn