How to Create an HTTPS Server in Java
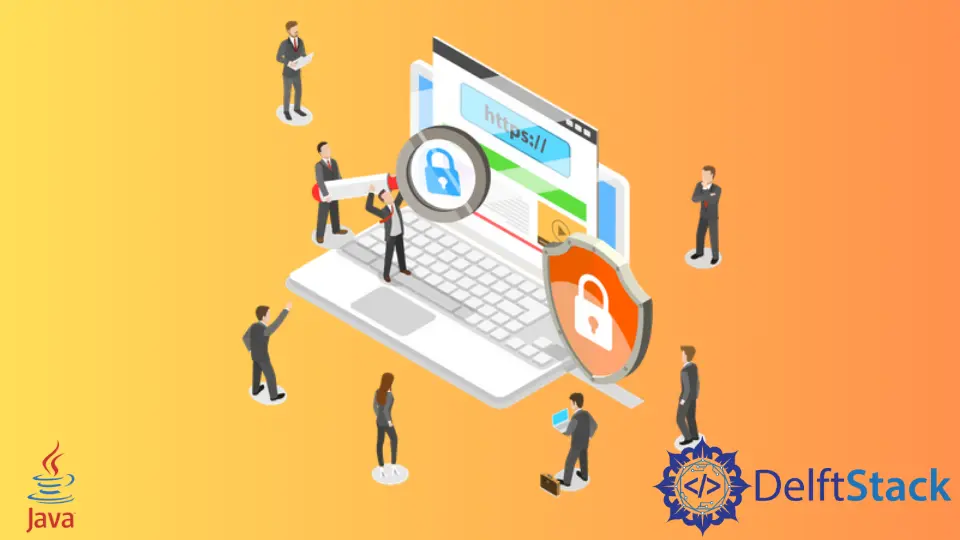
The Secure Hypertext Transfer Protocol (HTTPS) is a secured protocol that ensures safe communication through the internet. The HTTPS uses a pair of private keys and a digital certificate to verify the receiver and sender.
This tutorial will demonstrate creating a simple HTTPS server in Java.
Create an HTTPS Server in Java
Before creating an HTTPS server in JAVA, we have to make sure that we have generated the Keystore and Truststore, which the server will use. To generate them, follow the steps below:
-
Open Command Prompt as an administrator.
-
Go to the path of the Java bin directory in cmd.
-
Now run the following command:
keytool -genkeypair -keyalg RSA -alias selfsigned -keystore testkey.jks -storepass password -validity 360 -keysize 2048
-
This command will ask you your Lastname, Organizational unit, Organization, City, State, and two-letter country code. Provide all the info.
-
Type
yes
and press Enter as the last step.Output:
-
This means the
testkey.jks
file has been generated in the bin folder, copy it to your Java project folder to load it in the program.
Once the Keystore and Truststore are generated, we can create an HTTPS server in JAVA.
Example:
package delftstack;
import com.sun.net.httpserver.*;
import com.sun.net.httpserver.HttpExchange;
import com.sun.net.httpserver.HttpHandler;
import com.sun.net.httpserver.HttpsExchange;
import com.sun.net.httpserver.HttpsServer;
import java.io.*;
import java.net.InetSocketAddress;
import java.security.KeyStore;
import javax.net.ssl.KeyManagerFactory;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLEngine;
import javax.net.ssl.SSLParameters;
import javax.net.ssl.TrustManagerFactory;
public class HTTPS_Server {
public static class MyHandler implements HttpHandler {
@Override
public void handle(HttpExchange x) throws IOException {
String Response = "This is the response from delftstack";
HttpsExchange HTTPS_Exchange = (HttpsExchange) x;
x.getResponseHeaders().add("Access-Control-Allow-Origin", "*");
x.sendResponseHeaders(200, Response.getBytes().length);
OutputStream Output_Stream = x.getResponseBody();
Output_Stream.write(Response.getBytes());
Output_Stream.close();
}
}
public static void main(String[] args) throws Exception {
try {
// setup the socket address
InetSocketAddress Inet_Address = new InetSocketAddress(9000);
// initialize the HTTPS server
HttpsServer HTTPS_Server = HttpsServer.create(Inet_Address, 0);
SSLContext SSL_Context = SSLContext.getInstance("TLS");
// initialise the keystore
char[] Password = "password".toCharArray();
KeyStore Key_Store = KeyStore.getInstance("JKS");
FileInputStream Input_Stream = new FileInputStream("testkey.jks");
Key_Store.load(Input_Stream, Password);
// setup the key manager factory
KeyManagerFactory Key_Manager = KeyManagerFactory.getInstance("SunX509");
Key_Manager.init(Key_Store, Password);
// setup the trust manager factory
TrustManagerFactory Trust_Manager = TrustManagerFactory.getInstance("SunX509");
Trust_Manager.init(Key_Store);
// setup the HTTPS context and parameters
SSL_Context.init(Key_Manager.getKeyManagers(), Trust_Manager.getTrustManagers(), null);
HTTPS_Server.setHttpsConfigurator(new HttpsConfigurator(SSL_Context) {
public void configure(HttpsParameters params) {
try {
// initialise the SSL context
SSLContext SSL_Context = getSSLContext();
SSLEngine SSL_Engine = SSL_Context.createSSLEngine();
params.setNeedClientAuth(false);
params.setCipherSuites(SSL_Engine.getEnabledCipherSuites());
params.setProtocols(SSL_Engine.getEnabledProtocols());
// Set the SSL parameters
SSLParameters SSL_Parameters = SSL_Context.getSupportedSSLParameters();
params.setSSLParameters(SSL_Parameters);
System.out.println("The HTTPS server is connected");
} catch (Exception ex) {
System.out.println("Failed to create the HTTPS port");
}
}
});
HTTPS_Server.createContext("/test", new MyHandler());
HTTPS_Server.setExecutor(null); // creates a default executor
HTTPS_Server.start();
} catch (Exception exception) {
System.out.println("Failed to create HTTPS server on port " + 9000 + " of localhost");
exception.printStackTrace();
}
}
}
The code above creates a local host HTTPS server at port number 9000, which can be used for anything.
Output (in the IDE):
The HTTPS server is connected
Output (in the browser):
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook