Java Goto
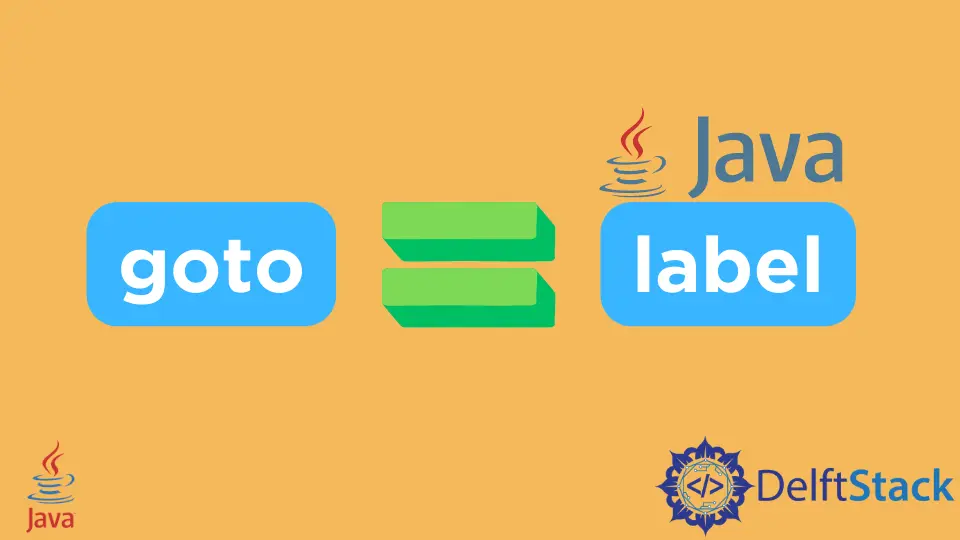
Unlike other programming languages, Java does not have goto
. Instead, Java contains the keyword label
.
The keyword label
is to change a program’s flow and jump to another section of the program based on the specified condition.
As we know, two basic keywords, break
and continue
, are used to change the direction of a loop. If you are working with loops, the keyword continue
will skip a specific part of code inside your loop based on the condition and the keyword break
will take you to program out of the loop based on the specific condition.
The keyword label
is only useful before the nested loop statements. You can also use the specified label name by combining the break
and continue
keywords.
In this article, we are going to see how we can use the keyword label
in our Java program and also we are going to discuss the topic by using necessary examples and explanations to make the topic easier.
Java Keyword label
With the Keyword Break
In our example below, we will learn how to use label
in our Java program. The example is very simple.
The code will be as follows:
class JavaGoto {
public static void main(String args[]) {
end:
for (int i = 0; i < 9; i++) {
if (i == 5) {
break end;
}
System.out.println("Current number is: " + i);
}
}
}