How to Get Screen Resolution in Java
- Find the Screen Resolution for Single Screen Window in Java
- Find the Screen Resolution for Multi-Screen Window in Java
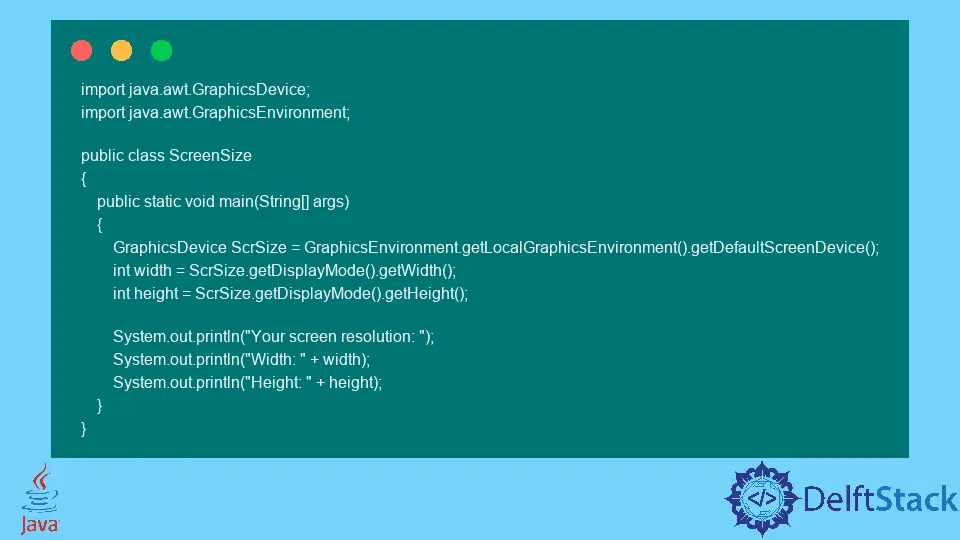
Sometimes you need to find the resolution of the screen for numerous purposes. You can easily find the size of the array by using Java.
This article will show how we can find the screen resolution in Java. Also, we will discuss the topic by following some examples and explanations to make the topic easier.
Find the Screen Resolution for Single Screen Window in Java
If you are working with a single-screen window, you need to follow this method to find the exact resolution of the screen.
In the example below, we will find the screen resolution; this example is for a single-screen window. The code for our example will be like the one below:
import java.awt.Dimension;
import java.awt.Toolkit;
public class ScreenSize {
public static void main(String[] args) {
Dimension ScrSize = Toolkit.getDefaultToolkit().getScreenSize();
int width = (int) ScrSize.getWidth();
int height = (int) ScrSize.getHeight();
System.out.println("Your screen resolution: ");
System.out.println("Width: " + width);
System.out.println("Height: " + height);
}
}
Let’s explain the code part by part. In this example we used the Dimension
to find the screen resolution by the line Dimension ScrSize = Toolkit.getDefaultToolkit().getScreenSize();
.
After that, we converted the height and width to the integer format by typecasting. Lastly, we printed the whole screen information.
When you run the above example code, you will get an output like the following:
Your screen resolution:
Width: 1366
Height: 768
Find the Screen Resolution for Multi-Screen Window in Java
If you are working with a multi-screen window, you need to follow this method to find the exact resolution of the screen. In our next example below, we will find the screen resolution.
This example is for the multi-screen window. The code for our example is shown below.
import java.awt.GraphicsDevice;
import java.awt.GraphicsEnvironment;
public class ScreenSize {
public static void main(String[] args) {
GraphicsDevice ScrSize =
GraphicsEnvironment.getLocalGraphicsEnvironment().getDefaultScreenDevice();
int width = ScrSize.getDisplayMode().getWidth();
int height = ScrSize.getDisplayMode().getHeight();
System.out.println("Your screen resolution: ");
System.out.println("Width: " + width);
System.out.println("Height: " + height);
}
}
Now we are going to explain the code part by part. In this example, we used GraphicsDevice
to find the screen resolution by the line:
GraphicsDevice ScrSize = GraphicsEnvironment.getLocalGraphicsEnvironment().getDefaultScreenDevice();
After that, we converted the height and width to the integer format by typecasting. Lastly, we printed the whole screen information.
When you run the above example code, you will get an output like the one below:
Your screen resolution:
Width: 1366
Height: 768
Above, we discuss two different methods for finding the screen’s resolution. You can choose any one of the methods based on the properties of the screening device.
Please note that the code examples shared here are in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn