Generic Interface in Java
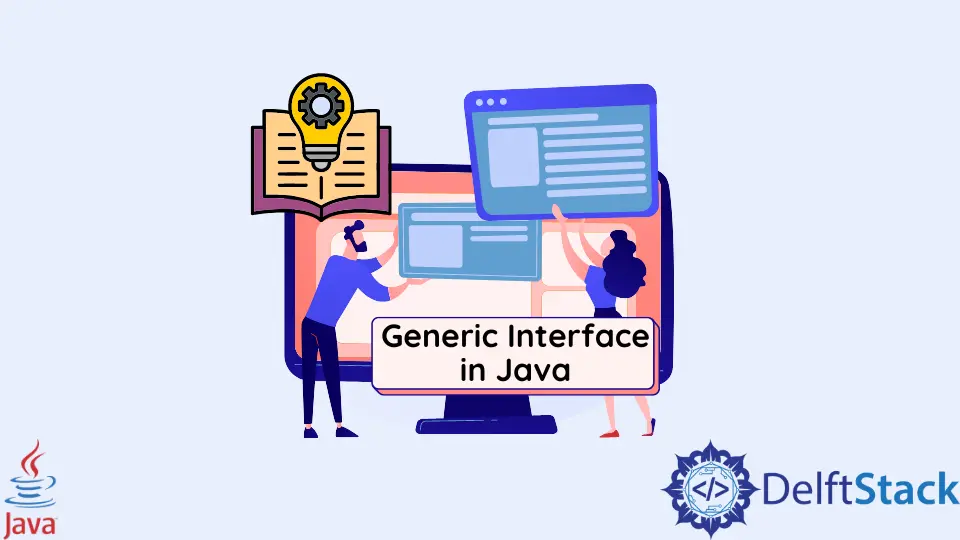
The Generic Interface
is the interface through which you can deal with abstract data types. It will help you to manipulate the Java Collections independently from representation details.
It is mainly used to achieve multiple inheritances in the form of hierarchy. The Generic Interface
can include all methods only.
Unlike commonly used classes, the Generic Interface
doesn’t contain any constructor.
By using the keyword implements
, you can easily make a connection with an Interface. You will get the benefits below from the Generic Interface
.
- You can easily implement different data types.
- It allows you to put constraints.
This article will discuss the Generic Interface
and how we can use it. We will discuss the topic using examples and explanations to make the topic easier.
Generic Interface in Java
Below we will see an example where we used Generic Interface
to find the max value from both the character array and integer array. The code for our example will be as follows:
interface FindMinMax<T extends Comparable<T>> {
T Max();
}
class MyClass<T extends Comparable<T>> implements FindMinMax<T> {
T[] vals;
MyClass(T[] o) {
vals = o;
}
public T Max() {
T Value = vals[0];
for (int i = 1; i < vals.length; i++) {
if (vals[i].compareTo(Value) > 0) {
Value = vals[i];
}
}
return Value;
}
}
public class GenericInterface {
public static void main(String args[]) {
Integer nums[] = {10, 6, 2, 8, 6};
Character chars[] = {'b', 'r', 'p', 'Z'};
MyClass<Integer> a = new MyClass<Integer>(nums);
MyClass<Character> b = new MyClass<Character>(chars);
System.out.println("The max number is: " + a.Max());
System.out.println("The max character is: " + b.Max());
}
}
In our above example, we first created an interface named FindMinMax
with an abstract method named Max()
. After that, we created another class named MyClass
where we defined that abstract method on the interface.
Finally, we created the class GenericInterface
, where we declared one integer array and one character array. Then we created two objects, one for numbers and another for chars
.
Lastly, we just printed the result as an output. Running the above code will show an output like the one below.
The max number is: 10
The max character is: r
An important note is that once you establish a bound, you don’t need to state it again using the implements
clause.
If you implement a class by using a Generic Interface
, then all classes must be generic so that they can take a type parameter passed to the interface.
Please note that the code examples shared here are in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn