How to Create Generators in Java
Sheeraz Gul
Feb 02, 2024
Java
Java Generator
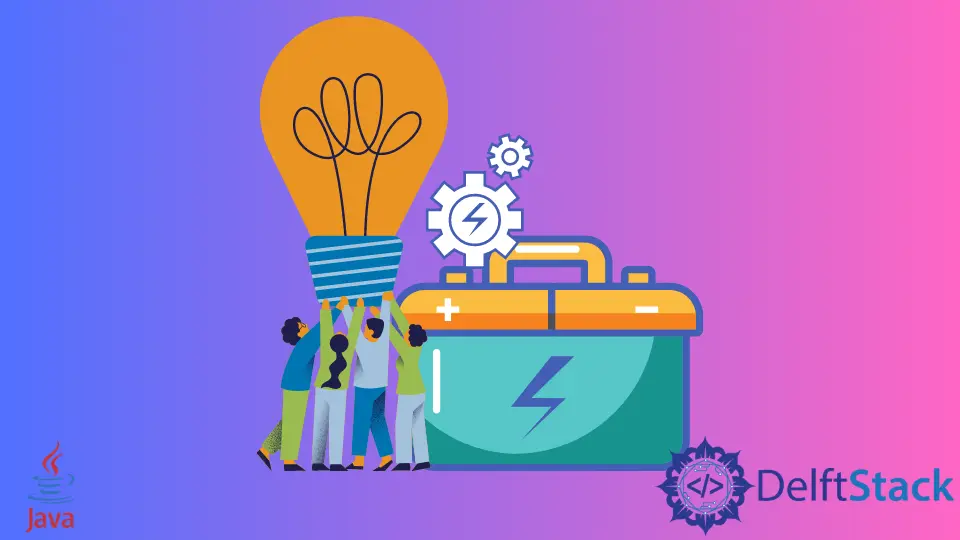
Generators are simple ways to create iterators. It can be described as an object or data which can be iterated over one value at a time.
Java does not provide any built-in functionality for generators like Python, but we can use an iterator to create one.
This tutorial will demonstrate how to create generators in Java.
Use Iterator to Create Generators in Java
Iterators are used to iterate through multidimensional arrays, which means they can perform the functionality of multi-layer loops.
package delftstack;
import java.util.Arrays;
import java.util.Iterator;
public class Java_Generator {
public static void main(String args[]) {
Integer[][][] Demo_Array =
new Integer[][][] {{{1, 2, null, 3}, null, {4}}, null, {{5}, {6}, null, {7}}};
Iterator<Integer> demo_iterator =
Arrays.stream(Demo_Array)
.filter(ab -> ab != null)
.flatMap(ab
-> Arrays.stream(ab)
.filter(ac -> ac != null)
.flatMap(ac -> Arrays.stream(ac).filter(ae -> ae != null)))
.iterator();
while (demo_iterator.hasNext()) {
System.out.println(demo_iterator.next());
}
}
}
The code above iterates through a three-dimensional array and prints the values neglecting the null values.
Output:
1
2
3
4
5
6
7
We can also use the Stream directly and set logic to iterate according to a particular pattern.
package delftstack;
import java.util.Iterator;
import java.util.stream.*;
public class Java_Generator {
public static void main(String args[]) {
Iterator<Integer> Demo_Generator =
Stream.iterate(new Integer[] {1, 2}, demo -> new Integer[] {demo[1], demo[0] + demo[1]})
.map(demo -> demo[0])
.iterator();
// Print the first 10 elements according to the given iterator logic
for (int x = 0; x < 10; x++) {
System.out.println(Demo_Generator.next());
}
System.out.println("First Iteration for 10 elements is finished here, Now the next 10");
// Print the first 10 elements according to the given iterator logic
for (int x = 0; x < 10; x++) {
System.out.println(Demo_Generator.next());
}
}
}