How to Force Garbage Collection in Java
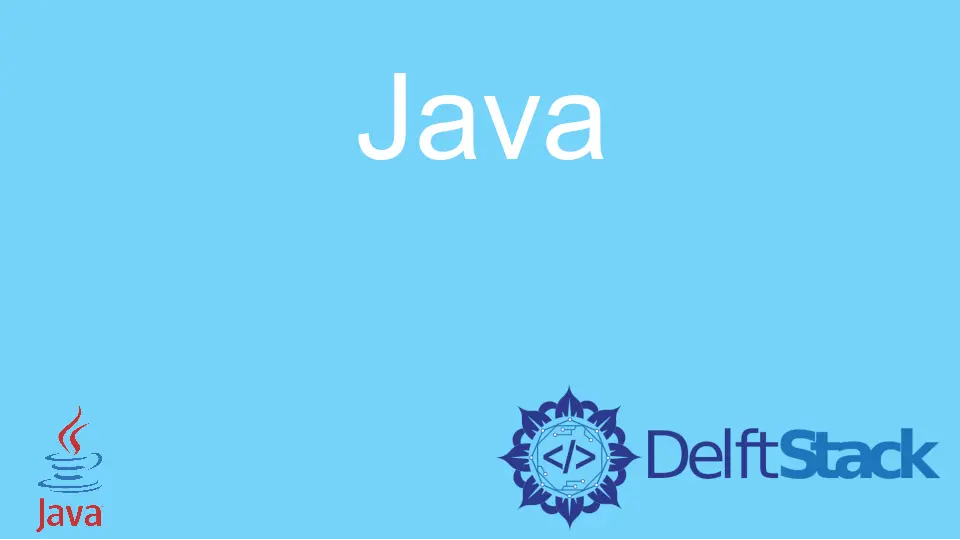
This tutorial demonstrates how to force garbage collection in Java.
Java Force Garbage Collection
Whenever the heap utilization approaches 100%, the risk of OutOfMemoryError
will increase to the maximum. At that time, the JVM will require to force the garbage collection and free up the memory.
Most of the time, we cannot force the JVM for garbage collection, but we can use a few methods to perform garbage collection whenever required.
This tutorial demonstrates the different methods of garbage collection in Java.
Call System.gc()
for Garbage Collection in Java
The System.GC()
method can be called anywhere in the Java code to perform garbage collection. It will prioritize the garbage collection, and whenever it is called a garbage collection will happen in seconds.
The syntax for this method is:
System.gc();
We only have to call System.gc()
to perform the garbage collection anywhere in the code. This will work in a similar way to force garbage collection.
Call Runtime.getRuntime().gc()
for Garbage Collection in Java
The Runtime.getRuntime().gc()
will call the System.gc()
, so it works in a similar way to prioritize garbage collection. The Runtime.getRuntime()
runs terminal commands in the Java code.
The methods Runtime.getRuntime().gc()
and System.gc
are the same. The syntax for this method is:
Runtime.getRuntime().gc();
Just call this method like the syntax, and the JVM will perform the garbage collection to free up the memory space.
Use jcmd
to Force Garbage Collection in Java
The jcmd
Java diagnostic command is a built-in utility from JDK that triggers a garbage collection routine. This garbage collection is triggered when the JVM can safely schedule a pause.
The command will throw the same error as jmap
if the JVM cannot perform the pause.
The syntax for this command is:
jcmd 1234 GC.run
Where the number is the process id, and GC.run
is used to invoke the garbage collection.
Use Java Mission Control or JConsole to Force Garbage Collection in Java
The Java Mission Control and JConsole provide a user-friendly interface that interacts with the jcmd
command. The Java Mission Control or JConsole can force the JVM for garbage collection.
The JConsole tool provides a button named Run Garbage Collection
on its memory management page. By clicking it, we can force JVM to perform garbage collection.
The Java Mission Control can help developers to select any jcmd
functionality, which also includes GC.run
, which can be executed by just clicking a button.
Both tools will invoke the jcmd
garbage collection command behind the scenes.
Use jmap
to Force Garbage Collection in Java
The JMAP (Java Memory Map) provides a method to print the heap’s histogram. And whenever the jmap
command is invoked, it will force the garbage collection routine, which is not considered the correct way to force a garbage collection.
If the JVM has a workload and garbage collection cannot be performed, then the jmap
command will throw an error.
See the command:
jmap -histo:live 1234
Where live
is the histogram option and the number in front of that is the process id.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook