Extends vs Implements in Java
-
Inherit a Class Using the
extends
Keyword in Java -
Inherit an Interface Using the
implements
Keyword in Java
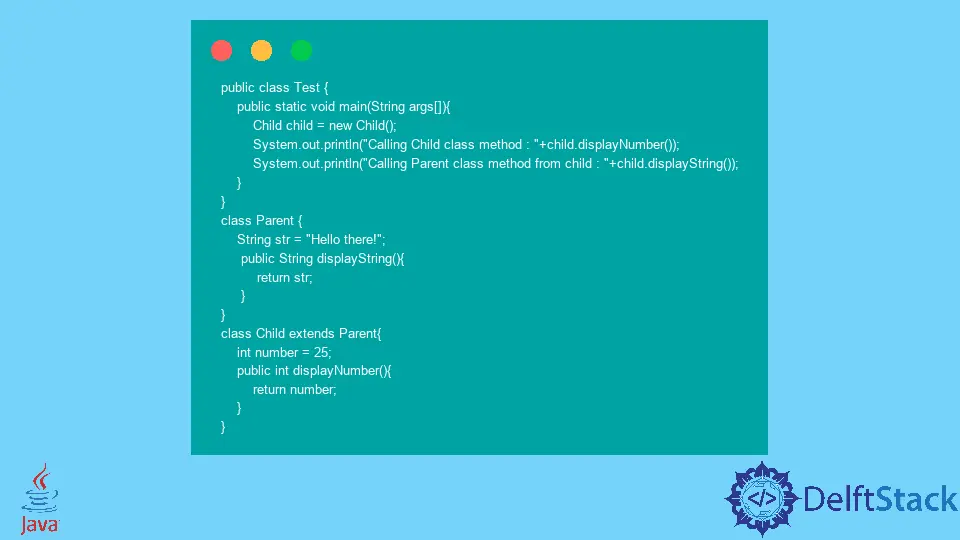
To reuse the properties and behaviors of a given parent class in Java, we use the concept of inheritance that is an important part of an object-oriented programming language. The idea behind this is code reusability. Both implements
and extends
keywords are used to create a new class in Java that has the attributes of the parent class.
We can have a better understanding of extends
and implements
keywords using the table given below.
Definition | Implementation | Class | Interface | Methods | |
---|---|---|---|---|---|
extends |
When a subclass(child) extends a base(parent) class, it allows the subclass to inherit the code defined in the base class. |
A class can inherit another class. An interface can inherit another interface using the keyword extends . |
A class can only extend one class. | Any number of interfaces can be extended by an interface. | The subclass class is extending a parent class may or may not override all the methods in the base class. |
implements |
The implements keyword is used to implement an interface. An interface contains abstract methods. The method body is defined in the class that implements it. |
Using the implements keyword a class can implement an interface. |
More than one interface can be implemented by a class. | An interface can never implement any other interface. | The class implementing an interface has to implement all the methods in the interface. |
Inherit a Class Using the extends
Keyword in Java
In the code below, we have a Parent
, which is the base class, and Child
as the subclass. The Child
class extends the Parent
class, which means that the Child
class now has access to the fields and methods defined inside the Parent
class.
In our Test
class, we create an object of the subclass class child
and call the method displayNumber()
on it. The method displayString()
, which is defined inside the parent class, can be executed by calling it on the same child
object. Hence, it fulfills the purpose of code reusability.
public class Test {
public static void main(String args[]) {
Child child = new Child();
System.out.println("Calling Child class method : " + child.displayNumber());
System.out.println("Calling Parent class method from child : " + child.displayString());
}
}
class Parent {
String str = "Hello there!";
public String displayString() {
return str;
}
}
class Child extends Parent {
int number = 25;
public int displayNumber() {
return number;
}
}
Output:
Calling Child class method : 25
Calling Parent class method from child : Hello there!
Inherit an Interface Using the implements
Keyword in Java
We have two interfaces, Cat
and Dog
, in the code below, each containing an abstract method inside it. A class Animals
implement both interfaces, so it must implement the method defined in the interfaces.
Hence the Animals
class implemented both interfaces and defined their behaviors which is the whole purpose of the interface in Java.
interface Cat {
public void meow();
}
interface Dog {
public void bark();
}
class Animals implements Dog, Cat {
@Override
public void meow() {
System.out.println("Cat meows");
}
@Override
public void bark() {
System.out.println("Dog barks");
}
}
public class Test1 {
public static void main(String args[]) {
Animals animals = new Animals();
animals.bark();
animals.meow();
}
}
Output:
Dog barks
Cat meows
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn