How to Encode a URL in Java
-
Encode a URL Using
URLEncoder
in Java -
Encode a URL Using
URL
,URI
andtoASCIIString()
in Java -
Encode a URL Using
URIBuilder
in Java
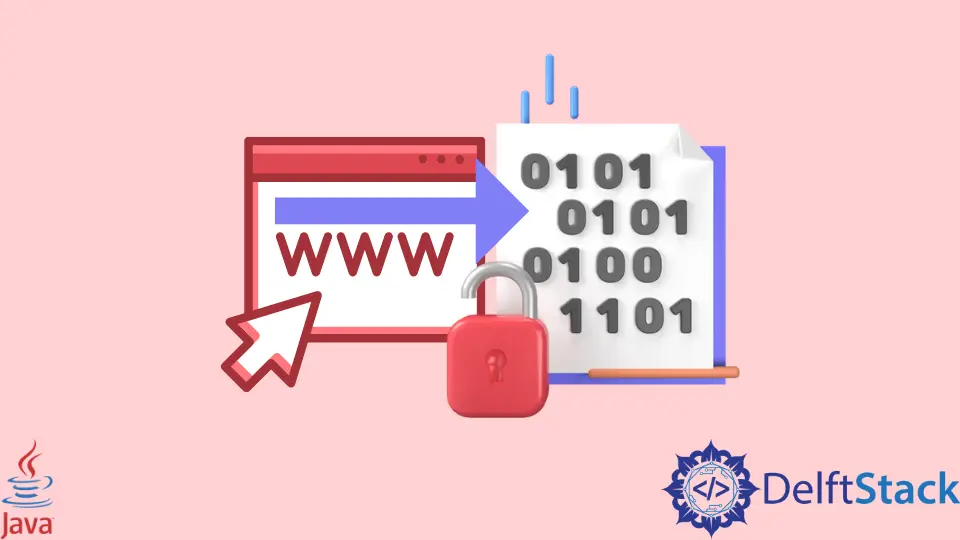
URLs cannot contain characters that are not included in the ASCII character set. When we want to send a URL over the Internet, we cannot append characters like whitespaces; this is why we use encoding to replace the invalid characters with valid characters like the plus sign or percentage sign. In the example programs below, we will see how to encode a URL using different methods.
Encode a URL Using URLEncoder
in Java
The utility class URLEncoder
helps us encode a given URL into the application/x-www-form-urlencoded
format. Below, we take a string q
that contains three words, separated by whitespaces.
If we concatenate q
at the end of a URL, it will be an invalid URL as a valid URL does not contain spaces. To fix this, we encode q
using the URLEncoder.encode()
method that accepts two arguments: first is the query string q
, and the second is the charset
to use.
The URLEncoder.encode()
method returns a string that we concatenate at the end of a URL as a query. When we print the final string encodedUrl
, it shows the whole valid URL, and a plus sign replaces the whitespaces.
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
public class EncodeUrl {
public static void main(String[] args) {
String q = "learn java language";
String encodedUrl = "www.google.com/search?q=" + URLEncoder.encode(q, StandardCharsets.UTF_8);
System.out.println("Encoded Url: " + encodedUrl);
}
}
Output:
Encoded Url: www.google.com/search?q=learn+java+language
Encode a URL Using URL
, URI
and toASCIIString()
in Java
The URL
class in Java constructs a URL and provides us with several useful methods to get information about the URL. We create the URL that returns a URL
object. Next, we call the URI
class’s constructor and pass the URL’s information like the protocol (https/http)
and the userinfo
using the getUserInfo()
method that contains details of authorization.
Now, we get the host using the getHost()
method and convert it into the ASCII Compatible Encoding (ACE) from Unicode. The next argument is the port fetched using the getPort()
method, then we get the path of URI, and the last two arguments are the query and reference.
Once all the arguments are passed into the URI
constructor, it returns a URI
object. This object is of the URI
type that we convert into an ASCII string using the toASCIIString()
method.
import java.net.*;
public class EncodeUrl {
public static void main(String[] args) throws MalformedURLException {
String q = "learn java language";
URL urlToEncode = new URL("https://www.google.com/search?q=" + q);
try {
URI uri = new URI(urlToEncode.getProtocol(), urlToEncode.getUserInfo(),
IDN.toASCII(urlToEncode.getHost()), urlToEncode.getPort(), urlToEncode.getPath(),
urlToEncode.getQuery(), urlToEncode.getRef());
String finalEncodedUrl = uri.toASCIIString();
System.out.println("Encoded Url: " + finalEncodedUrl);
} catch (URISyntaxException e) {
e.printStackTrace();
}
}
}
Output:
Encoded Url: https://www.google.com/search?q=learn%20java%20language
Encode a URL Using URIBuilder
in Java
In this example, we use the Apache HttpClient
library instead of native methods. To include the library in our project, we’ll use the following dependency.
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
We use the URIBuilder
class of the org.apache.http.client.utils
package. We create an object of URIBuilder
and pass the urlToEncode
class in its constructor. Next, we add our query q
as a parameter using the uriBuilder.addParameter()
method and pass the query parameter and its value.
In the end, we print UriBuilder
as a string that displays the whole encoded URL.
import java.net.*;
import org.apache.http.client.utils.URIBuilder;
public class EncodeUrl {
public static void main(String[] args) throws MalformedURLException {
String q = "learn java language";
String urlToEncode = "https://www.google.com/search";
try {
URIBuilder uriBuilder = new URIBuilder(urlToEncode);
uriBuilder.addParameter("q", q);
System.out.println("Encoded Url: " + uriBuilder.toString());
} catch (URISyntaxException e) {
e.printStackTrace();
}
}
}
Output:
Encoded Url: https://www.google.com/search?q=learn+java+language
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn