Embedded Database in Java
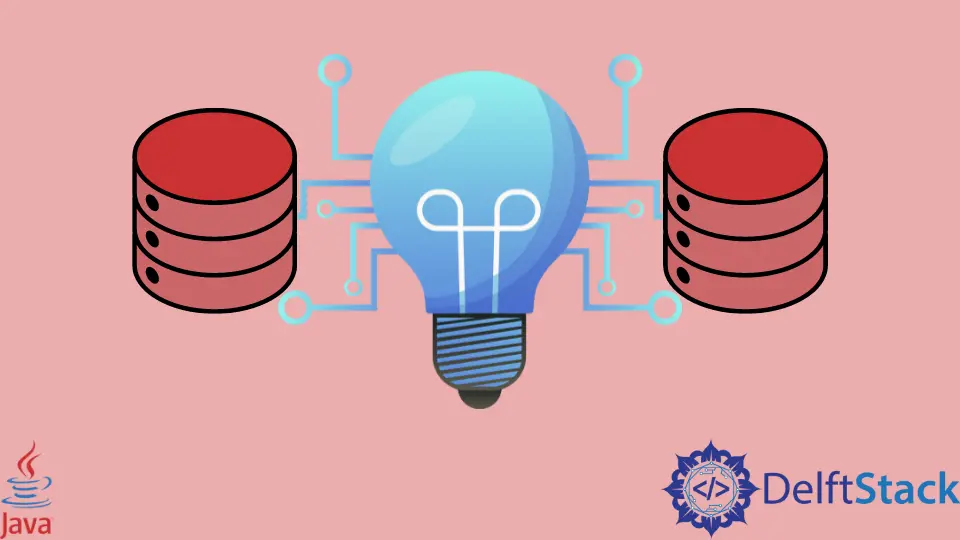
A database technology in which the database solutions are embedded into the application, not as the standalone, is known as an embedded database. These databases are lightweight, fast, and come with ease of configuration and improved testability which makes them helpful in the development and testing phase.
There are many types of embedded databases for Java. This tutorial compares a few best choices for embedded databases in Java.
The picture below demonstrates how the embedded databases work.
Apache Derby
The Apache Derby is an open-source database that is implemented in Java. This database is under the license of Apache.
The standards of this database are JDBC and SQL. It also supports client/server mode.
The derby supports all the features of relational databases.
We use derby.jar
to implement the Apache derby in Java. To make the connection follow the commands below.
// load driver
Class.forName("org.apache.derby.jdbc.EmbeddedDriver");
Connection c = DriverManager.getConnection("jdbc:derby:testdb1;create=true")
The create=true
will create the database if it does not exist and then make a connection; we only use this parameter when we want to create a database if it doesn’t exist.
Apache derby jar
can be downloaded from here.
HSQLDB (HyperSQL Database)
The HyperSQL is made on the SQL standard and JDBC specifications. HyperSQL supports all features that are used in a modern relational database.
It supports both embedded and server modes. This database is purely developed in Java.
The HyperSQL also supports PreparedStatement
and CallableStatement
; it also supports two-phase locking and multi-version concurrency control.
The HyperSQL comes in a jar
package which includes the HyperSQL, RDBMS Engine, and JDBC driver to embed the HyperSQL into the Java application. To make a connection of HyperSQL in Java, follow the commands below.
// load driver
Class.forName("org.hsqldb.jdbcDriver");
Connection connection = DriverManager.getConnection("jdbc:hsqldb:testdb", "sa", "");
The HyperSQL package can be downloaded from here.
H2
The H2 is also an open-source database based on SQL standards and JDBC API support. It also supports embedded and server modes, multi-version concurrency and row-level locking.
To make the connection with H2 in Java, follow the commands below.
// load driver
Class.forName("org.h2.Driver");
Connection connection = DriverManager.getConnection("jdbc:h2:~/test", "sa", "");
Where ~/
denotes the user home directory, compared to other databases, the H2 is quite comprehensive.
The H2 database can be downloaded from here.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook