Ellipsis in Java
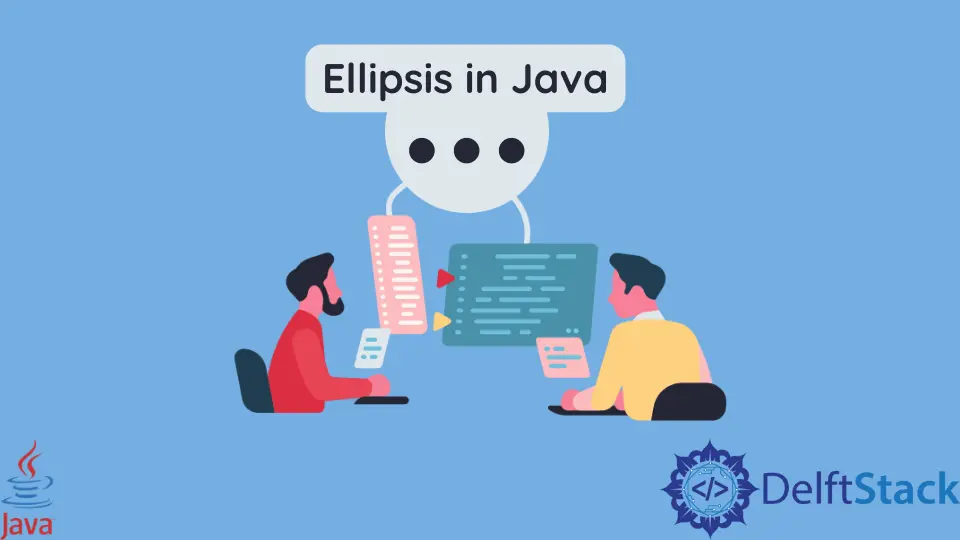
Ellipsis
is an uncommon Java syntax that new programmers need to learn. Even some of the experienced programmers don’t have much experience with it.
This could be very easy if you have several variables with the same datatypes and want to pass them in a method.
In this way, you don’t need to receive every single variable one by one. You need to include the ...
inside your method and have all the variables inside your method.
In this article, we will discuss the use of Ellipsis
or ...
. Also, we will describe the topic by using necessary examples and explanations to make the topic easier.
Ellipsis or ...
in Java
In the example below, we will see how we can use the Ellipsis
or ...
in our Java program. The code will be as follows:
class Ellipsis {
int Sum(int... numbers) {
int Sum = 0;
for (int number : numbers) {
Sum += number;
}
return Sum;
}
public static void main(String[] args) {
Ellipsis elp = new Ellipsis();
System.out.println("The sum is: " + elp.Sum());
System.out.println("\nThe sum is: " + elp.Sum(2, 2));
System.out.println("\nThe sum is: " + elp.Sum(9, 7, 10, 9, 10));
}
}
In the example above, we first created a class named Ellipsis
. After that, we created a method named Sum
where we passed all the numbers using the ...
.
Now we have created an object of the class and called the method with values. Lastly, we print all the necessary values.
When you run the Java program above, you will get an output like the one below.
The sum is: 0
The sum is: 4
The sum is: 45
One important note here, the use of Ellipsis
will only work if you have variables with the same data types.
Otherwise, it may show you an error. Besides, you should not create an overload like the one below.
Misuse of Ellipsis
or ...
in Java
Below we will see an example of the error if you misuse the Ellipsis
or ...
. Take a look at the below code.
class Ellipsis {
int Sum(int a, int... numbers) {
int sum = 0;
for (int number : numbers) {
sum += number;
}
return sum;
}
public static void main(String[] args) {
Ellipsis elp = new Ellipsis();
System.out.println("The sum is: " + elp.Sum() + "\n");
System.out.println("\nThe sum is: " + elp.Sum(2, 2));
System.out.println("\nThe sum is: " + elp.Sum(9, 7, 10, 9, 10));
}
}
In the example above, we overloaded the method Sum()
on the line int Sum(int a, int... numbers){
. This will create a runtime error.
When you try to run the above code, you will get an error like the one below.
error: Class names, 'Ellipsis', are only accepted if annotation processing is explicitly requested
1 error
Please note that the code examples shared here are in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn