What Does the <E> Mean in Java
-
Understanding
Generics
andType
Parameters -
Declaring
Generics
in Classes and Interfaces - Instantiating a Generic Class
-
Use
Generics
<E>
in Java to Create a NewList
-
Using
<E>
in Methods in Java - Wildcards and Bounds
-
Advantages of Using
<E>
Syntax in Java - Conclusion
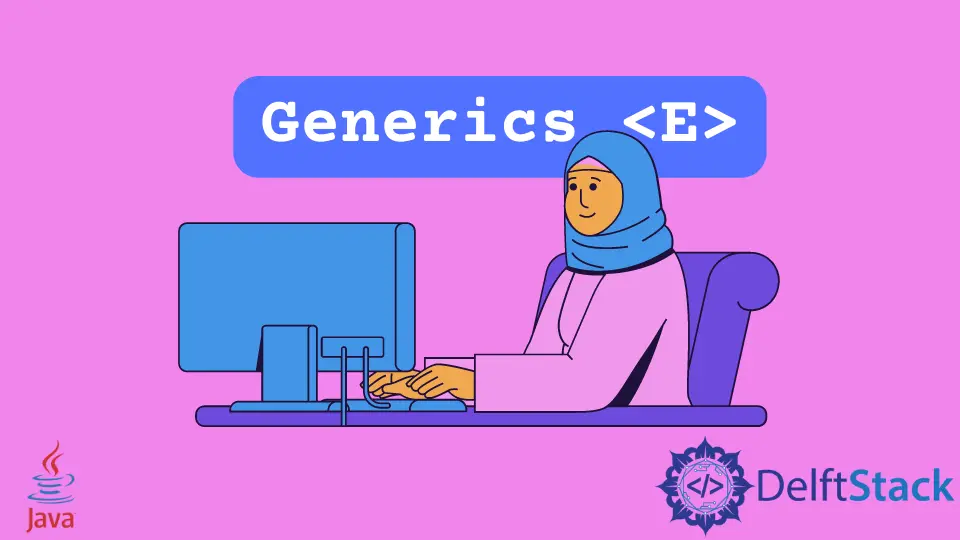
Java, as a versatile and object-oriented programming language, introduces a powerful mechanism known as generics. Generics enable developers to create flexible and reusable code by allowing classes, interfaces, and methods to work with any data type.
The <E>
syntax, commonly referred to as a type parameter, is a fundamental aspect of generics in Java. Generics
were added in Java 5 to improve the abstraction and provide a way to use a method, interface, or single class with different objects or data types.
There is a common naming convention related to Generics
like T
represents Type
, and V
is used for Value
. We look at E
, another type-parameter name used in Generics
that usually stands for Element
in this article.
In this comprehensive exploration, we will delve into the intricacies of the <E>
syntax, understanding its significance, applications, and the benefits it brings to Java programming.
Understanding Generics
and Type
Parameters
Generics
in Java were introduced with Java 5 to enhance the type-safety and reusability of code. They provide a way to create classes, interfaces, and methods that operate on a variety of data types without sacrificing compile-time type checking.
The <E>
syntax, where 'E'
stands for Element
, is a common naming convention used for the type parameter.
However, it’s essential to note that 'E'
is just a placeholder, and any valid identifier can be used. The choice of 'E'
is merely a convention to represent the generic element type.
Declaring Generics
in Classes and Interfaces
When declaring a class or interface with generics, the <E>
syntax is used within angle brackets following the class or interface name. Here’s a simple example:
public class Box<E> {
private E content;
public void setContent(E content) {
this.content = content;
}
public E getContent() {
return content;
}
}
In this example, the Box
class is declared with a generic type parameter <E>
. The content
variable and the getter and setter methods utilize this type of parameter.
This allows the Box
class to work with any data type without sacrificing type safety.
Instantiating a Generic Class
When creating an instance of a generic class, you specify the actual data type you want to use for the generic type parameter. This is done within the angle brackets during instantiation.
For example:
public class Main {
public static void main(String[] args) {
Box<String> stringBox = new Box<>();
stringBox.setContent("Hello, Generics!");
String content = stringBox.getContent();
System.out.println("Box content: " + content);
}
}
In this example, a Box
instance is created with the generic type parameter <String>
. This ensures that the content
variable inside the Box
class can only hold String
objects.
Use Generics
<E>
in Java to Create a New List
The best way to understand Generics
is by using a collection because, without Generics
, collections like lists used to take a lot of code to write. Still, we can create a List
or any other collection with very little code after introducing Generics
.
The generic name <E>
stands for Element
and is commonly used in the Java Collections
Framework. In the example below, we create a static method called newListWithElements()
that takes a generic type E
parameter with the variable arguments operator ...
called listElements
.
The newListWithElements()
method returns a List
of type <E>
, which is a generic type parameter. We also use a type parameter before the return type and after static
or any access modifier using a generic method.
Inside the function, we create an int
variable listElementsSize
and initialize it with the length of the listElements
parameter. If it is null
, we initialize it with zero.
Then we create an ArrayList
constructor and pass listElementsSize
, which is the size of the List
, which returns a List
that is of type <E>
.
Now we collect all the listElements
elements and create a new list using Collections.addAll()
that adds all the elements to the new list listOfElements
. We have a new list that we return from the method.
In the main()
function, we call newListWithElement()
three times with types like String
, Integer
, and Boolean
. Generics
make it possible to create a list of multiple object types with a single method.
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class JavaEGenerics {
public static void main(String[] args) {
List<String> newStringList = newListWithElements("element1", "element2", "element3");
List<Integer> newIntList = newListWithElements(11, 12, 13);
List<Boolean> newBooleanList = newListWithElements(true, false);
System.out.println(newStringList);
System.out.println(newIntList);
System.out.println(newBooleanList);
}
static <E> List<E> newListWithElements(E... listElements) {
int listElementsSize = 0;
if (listElements != null)
listElementsSize = listElements.length;
List<E> listOfElements = new ArrayList<>(listElementsSize);
if (listElements != null)
Collections.addAll(listOfElements, listElements);
return listOfElements;
}
}
Output:
[element1, element2, element3]
[11, 12, 13]
[true, false]
Using <E>
in Methods in Java
Generics
are not limited to classes and interfaces; they can also be used in methods. The <E>
syntax can be applied to method declarations to create generic methods.
Here’s an example:
import java.util.*;
public class GenericMethods {
public static <E> void printElement(E element) {
System.out.println("Element: " + element);
}
public static void main(String[] args) {
printElement("Hello, Generics!");
printElement(42);
printElement(3.14);
}
}
In this example, the printElement
method is declared with a generic type parameter <E>
. This allows the method to accept arguments of any data type.
Wildcards and Bounds
Java generics offer additional features, such as wildcards and bounds, which can further enhance their flexibility. Wildcards, denoted by ?
, allow for more flexibility when working with unknown generic types.
Bounds, on the other hand, restrict the types that can be used as type arguments. The <E>
syntax is often involved in specifying bounds and working with wildcards.
Here’s a brief example using wildcards:
import java.util.*;
public class WildcardExample {
public static double sum(List<? extends Number> numbers) {
double total = 0.0;
for (Number number : numbers) {
total += number.doubleValue();
}
return total;
}
public static void main(String[] args) {
List<Integer> integers = List.of(1, 2, 3, 4, 5);
List<Double> doubles = List.of(1.1, 2.2, 3.3, 4.4, 5.5);
System.out.println("Sum of integers: " + sum(integers));
System.out.println("Sum of doubles: " + sum(doubles));
}
}
In this example, the sum
method accepts a list of numbers, where the generic type is a subclass of Number
. The <E>
syntax is implicitly involved in the use of wildcards.
Advantages of Using <E>
Syntax in Java
1. Type Safety
Generics
provides compile-time type checking, reducing the chances of runtime errors related to data type mismatches. The <E>
syntax ensures that the correct data type is used throughout the code.
2. Code Reusability
With generics, classes, and methods become more versatile as they can be used with different data types. This enhances code reusability and promotes a modular programming approach.
3. Readability
Generics
improve code readability by making it clear which data types a class or method is designed to work with. The <E>
syntax serves as a visual indicator of the generic nature of the code.
4. Maintainability
Changes to the underlying data types can be made more easily without affecting the entire codebase. Generics
provide a level of abstraction that simplifies maintenance.
Conclusion
The <E>
syntax in Java generics is a cornerstone for creating flexible, type-safe, and reusable code. It allows developers to design classes, interfaces, and methods that can work with various data types without sacrificing compile-time type checking.
Understanding the power and flexibility that generics bring to Java programming and mastering the <E>
syntax empowers developers to write more modular, maintainable, and readable code. As generics continue to play a crucial role in modern Java development, a solid grasp of the <E>
syntax is essential for Java programmers aiming to build robust and flexible applications.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn