How to Delete Folders Using Java
-
Use
delete()
ofFile
Class to Delete Empty Folders Using Java - Delete Folders Recursively Using Java
- Use Java 8 Streams and NIO2 to Delete Folders Using Java
- Use Apache Common IO to Delete Folders Using Java
- Conclusion
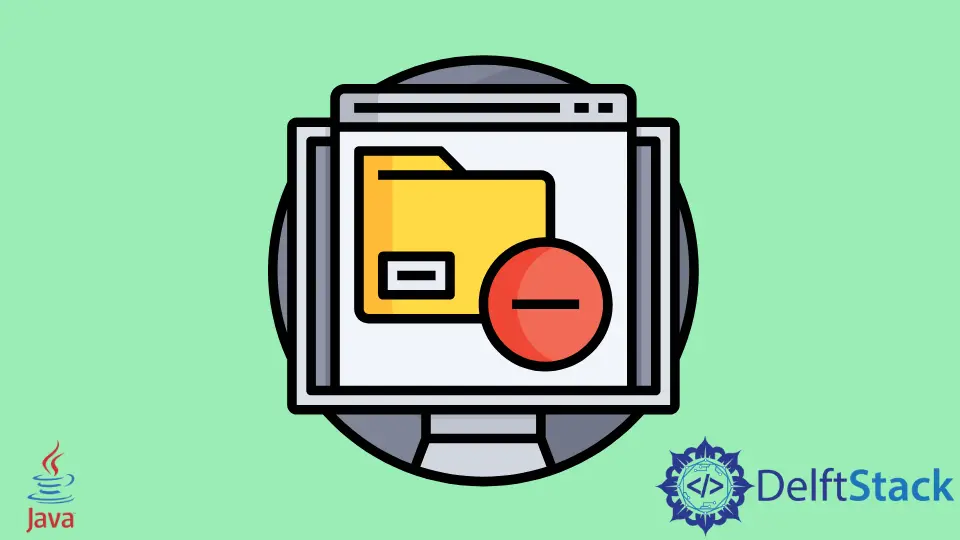
In this article, we will learn how to delete folders/directories using the Java programming language. There are multiple ways to do this. Let’s look at them one by one.
Use delete()
of File
Class to Delete Empty Folders Using Java
In Java, we have the java.io.File
class, which contains a method called delete()
used to delete files and empty directories.
Syntax:
file_object.delete()
Let’s assume that an empty directory with the name test
exists in our system’s D:
drive. Now, let’s see how to delete this using the delete()
method.
Example code:
import java.io.File;
public class Example {
public static void main(String[] args) {
String path = "D:\\test";
File obj = new File(path);
obj.delete();
}
}
When the above code is executed, we see that the folder test
is deleted from our system’s D:
drive.
In the above code, we have created a string variable that stores the directory’s path. Then we used this path to create our file object obj
.
At last, we have used the delete()
method, which deletes the directory at that path referenced by the file object obj
.
But what if the folder test
contains some files, let’s say file1.txt
and file2.txt
? In this scenario, when the above code is executed, nothing happens as the directory is not empty, and we know that the delete()
method only works for empty directories.
We can overcome this issue of deleting non-empty directories using recursive deletion.
Delete Folders Recursively Using Java
In this method, we will use the delete()
method along with recursion. The idea is to delete all the sub-folders and files present inside our test
directory first, and once it is empty, we can delete it using the delete()
method.
Directory structure:
test
/ | \
/ | \
/ | \
file1.txt | file2.txt
|
hero
/ \
file3.txt file4.txt
We have a test
folder in the D:
drive, which has two text files: file1.txt
and file2.txt
and a sub-folder hero
, which again contains two text files: file3.txt
and file4.txt
.
So here, we will delete file1.txt
, file2.txt
, and then we go inside the sub-folder hero
and delete file3.txt
and file4.txt
. As the sub-folder is empty, we can easily delete it.
After all this, the directory test
also becomes empty, which can be easily deleted using the delete()
method.
Algorithm:
-
Get all the files and sub-folders of the directory to be deleted.
-
All the children, which are not directories, meaning files should be deleted.
-
Exit from the recursion.
-
For each child, which is a sub-folder/sub-directory, recursively repeat steps 1 to 3.
-
Delete the main directory using the
delete()
method.
We have to use two functions to implement the above algorithm: listFiles()
and isDirectory()
.
The listFiles()
method returns an array of abstract paths (objects) of all the files and sub-folders in our target directory. The isDirectory()
is a boolean function that returns true
if the current object is a directory; else, it returns false
.
Example code:
import java.io.File;
public class Example {
static void recursiveDelete(File targetDirectory) {
File[] data = targetDirectory.listFiles();
for (File file : data) {
if (file.isDirectory())
recursiveDelete(file);
else
file.delete();
}
targetDirectory.delete();
}
public static void main(String[] args) {
String path = "D:\\test";
File obj = new File(path);
recursiveDelete(obj);
}
}
Use Java 8 Streams and NIO2 to Delete Folders Using Java
In this method, we can use the Files.walk(Path)
method that returns Stream<Path>
, which contains all the files and sub-folders in that path.
Example code:
import java.io.*;
import java.nio.file.*;
import java.util.*;
public class Example {
public static void main(String[] args) throws IOException {
Path path = Paths.get("D:\\test");
Files.walk(path).sorted(Comparator.reverseOrder()).forEach(data -> {
try {
System.out.println("deleting: " + data);
Files.delete(data);
} catch (IOException obj) {
obj.printStackTrace();
}
});
}
}
When the above code is executed, it deletes all the contents of the directory and then the directory itself in a Depth First Search fashion.
Output:
deleting: D:\test\hero\file4.txt
deleting: D:\test\hero\file3.txt
deleting: D:\test\hero
deleting: D:\test\file2.txt
deleting: D:\test\file1.txt
deleting: D:\test
Use Apache Common IO to Delete Folders Using Java
So far, all the methods we have seen are plain old Java methods that use some concepts of recursion along with file
and stream
methods. But we can use Apache Common IO FileUtils.deleteDirectory
to delete a directory.
The main advantage of using this over primitive Java methods is that the line of codes (LOC) is significantly less, making it an easy and more efficient way of writing.
To use Apache common IO, we must first add dependencies in our pom.xml
file.
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.11.0</version>
</dependency>
Example code:
import java.io.*;
import org.apache.commons.io.FileUtils;
public class Example {
public static void main(String[] args) throws IOException {
String path = "D:\\test";
File obj = new File(path);
FileUtils.deleteDirectory(obj);
}
}
Conclusion
This article has shown different ways of deleting directories using Java. We understood how to use recursion and Java 8 streams.
We also understood how using Apache Commons IO could be more efficient and time-saving where LOC (line of codes) greatly affects the performance of our project.