How to Copy Text to Clipboard in Java in Java
- Clipboard Functionality in Java
- Methods How to Copy Text to Clipboard in Java
- Best Practices for Clipboard Functionality in Java
- Conclusion
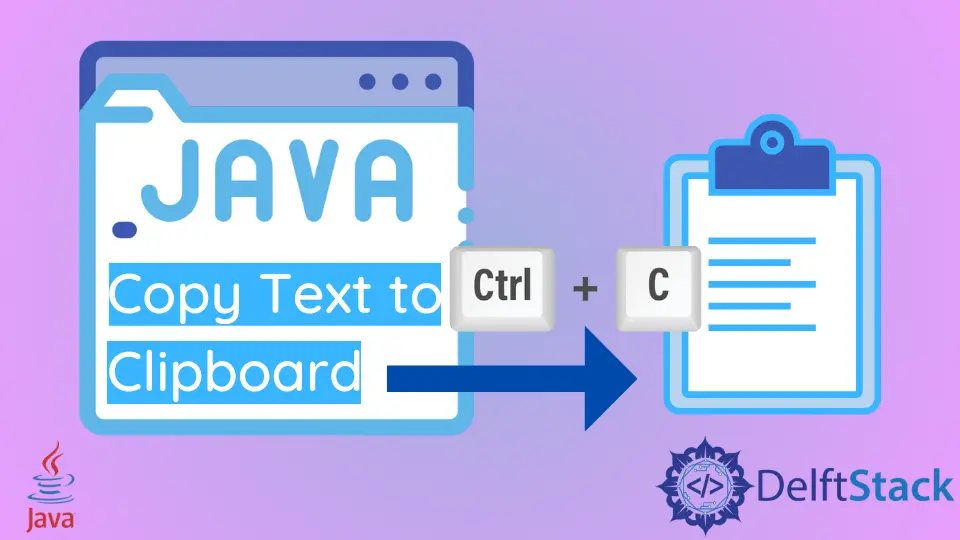
This article explores the crucial aspects of clipboard functionality in Java and delves into various methods for efficiently copying text, providing developers with versatile approaches for seamless integration in their applications.
Java provides several elements that display the text in a Java application. Multiple times, the text must be copied to the clipboard to export to other computer applications.
Clipboard Functionality in Java
Copying text to the clipboard in Java is crucial for enhancing user experience and facilitating seamless data transfer within applications. This functionality allows users to easily transfer information between different components of a program or between different applications running on the same system.
By utilizing the clipboard, developers can enable users to copy text from one input field or document and paste it into another without the need for manual typing, reducing errors and saving time. This feature is particularly valuable in applications that involve text editing, data manipulation, or any scenario where the exchange of textual information is a common requirement.
Additionally, copying to the clipboard in Java contributes to a more intuitive and efficient user interface, fostering a smoother interaction between users and software.
Methods How to Copy Text to Clipboard in Java
Clipboard
and StringSelection
Classes
Using the Clipboard
and StringSelection
classes in Java for copying text to the clipboard is crucial due to their simplicity and compatibility across various Java applications. These classes provide a standardized and platform-independent approach, ensuring consistent behavior.
The StringSelection
class allows easy encapsulation of text, while the Clipboard
class provides a uniform interface for clipboard operations. This method is preferred over alternatives for its reliability, ease of implementation, and adaptability, making it a versatile choice for developers seeking a straightforward and effective solution for clipboard interactions in Java applications.
Code Example:
import java.awt.Toolkit;
import java.awt.datatransfer.Clipboard;
import java.awt.datatransfer.StringSelection;
public class ClipboardExample {
public static void copyToClipboard(String text) {
StringSelection stringSelection = new StringSelection(text);
Clipboard clipboard = Toolkit.getDefaultToolkit().getSystemClipboard();
clipboard.setContents(stringSelection, null);
}
public static void main(String[] args) {
String textToCopy = "Hello, Clipboard!";
copyToClipboard(textToCopy);
System.out.println("Text copied to clipboard: " + textToCopy);
}
}
In the ClipboardExample
class, the copyToClipboard
method facilitates text copying by creating a StringSelection
object initialized with the specified text. This object is crucial as it acts as a Transferable
, necessary for placing data on the clipboard.
Access to the system clipboard is obtained using Toolkit.getDefaultToolkit().getSystemClipboard()
. Subsequently, the contents of the clipboard are set to our StringSelection
through the setContents
method.
In the main
method, this process is exemplified by creating sample text and invoking the copyToClipboard
method. Finally, a message is printed to signify the successful copying of the text to the clipboard.
Output:
This signifies that the text Hello, Clipboard!
has been successfully copied to the system clipboard using the Clipboard
and StringSelection
methods in Java.
This straightforward approach is particularly useful in scenarios where quick and efficient text transfer is essential, contributing to an improved user experience in Java applications.
ClipboardOwner
Interface
Utilizing the ClipboardOwner
interface in Java for copying text to the clipboard holds significance due to its ability to track ownership changes. Unlike alternative methods, the ClipboardOwner
interface enables developers to respond dynamically when the clipboard ownership is transferred, allowing for customized actions.
This adaptability is valuable in scenarios where applications need to react to changes in the clipboard state. It enhances control and responsiveness, making the ClipboardOwner
interface a preferred choice for developers seeking a flexible and interactive approach to manage clipboard interactions in Java applications.
Code Example:
import java.awt.Toolkit;
import java.awt.datatransfer.Clipboard;
import java.awt.datatransfer.ClipboardOwner;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.io.IOException;
public class ClipboardExample implements ClipboardOwner {
public void copyToClipboard(String text) {
Clipboard clipboard = Toolkit.getDefaultToolkit().getSystemClipboard();
clipboard.setContents(new StringSelection(text), this);
}
@Override
public void lostOwnership(Clipboard clipboard, Transferable contents) {
// Handle clipboard ownership changes if needed
}
public static void main(String[] args) {
ClipboardExample clipboardExample = new ClipboardExample();
String textToCopy = "Hello, Clipboard!";
clipboardExample.copyToClipboard(textToCopy);
System.out.println("Text copied to clipboard: " + textToCopy);
}
}
In the ClipboardExample
class, we implement the ClipboardOwner
interface to enable dynamic control over clipboard interactions. By obtaining a reference to the system clipboard using Toolkit.getDefaultToolkit().getSystemClipboard()
, we establish a connection.
The copyToClipboard
method sets the clipboard contents to a new StringSelection
, designating the current instance as the clipboard owner. The lostOwnership
method, invoked during ownership changes, offers a customizable way to handle such transitions.
In the main
method, we showcase usage by creating an instance of ClipboardExample
and copying text to the clipboard. The process concludes with a printed message affirming the successful text transfer to the clipboard.
This approach grants developers a flexible and interactive means to manage clipboard actions in Java applications.
Output:
This output signifies the successful copying of the text Hello, Clipboard!
to the system clipboard using the ClipboardOwner
method in Java. This approach provides a flexible means of managing clipboard ownership, making it especially useful in scenarios where monitoring changes to the clipboard is necessary.
JavaFX
Leveraging JavaFX applications for copying text to the clipboard in Java holds significance due to their seamless integration with graphical user interfaces (GUIs). JavaFX provides a user-friendly approach, simplifying the implementation of clipboard operations within interactive applications.
The ease of incorporating buttons, text fields, and event handling in JavaFX makes it an intuitive choice for developers. This method enhances the user experience, particularly in scenarios where graphical elements play a crucial role, offering a visually appealing and straightforward way to facilitate text copying in Java applications.
Code Example:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.input.Clipboard;
import javafx.scene.input.ClipboardContent;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ClipboardExample extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Clipboard Example");
TextField textField = new TextField();
Button copyButton = new Button("Copy to Clipboard");
copyButton.setOnAction(e -> copyToClipboard(textField.getText()));
VBox vBox = new VBox(10);
vBox.getChildren().addAll(textField, copyButton);
Scene scene = new Scene(vBox, 300, 150);
primaryStage.setScene(scene);
primaryStage.show();
}
private void copyToClipboard(String text) {
Clipboard clipboard = Clipboard.getSystemClipboard();
ClipboardContent content = new ClipboardContent();
content.putString(text);
clipboard.setContent(content);
}
public static void main(String[] args) {
launch(args);
}
}
In the ClipboardExample
JavaFX application, we begin by defining a class that extends the Application
class, serving as the application’s entry point. Within this application, we create essential user interface components like a TextField
for input and a button labeled Copy to Clipboard
.
The button is configured to trigger the copyToClipboard
method upon clicking, transferring the text from the TextField
to the clipboard. The method efficiently accesses the system clipboard, creates ClipboardContent
with the specified text, and sets the clipboard content.
To ensure a clean interface, the UI components are organized in a vertical box layout. The scene is then set up, and the primary stage is displayed with the specified dimensions, providing a simple and visually appealing JavaFX solution for copying text to the clipboard.
Output:
Upon running the application, a window titled Clipboard Example
will appear with a text field and a button. Users can input text into the field and click the Copy to Clipboard
button.
The specified text will be copied to the system clipboard, demonstrating the effectiveness of the JavaFX method for handling clipboard operations in a user-friendly manner. This approach is particularly valuable in applications where graphical user interfaces play a significant role.
Best Practices for Clipboard Functionality in Java
Input Validation
Ensure proper validation of input data before copying to the clipboard to prevent unintended behavior or security vulnerabilities.
Error Handling
Implement robust error handling mechanisms to gracefully manage scenarios where clipboard operations may fail or encounter issues.
Avoiding Sensitive Data
Exercise caution when dealing with sensitive information, and refrain from automatically copying sensitive data to the clipboard without explicit user consent.
Thread Safety
Consider thread safety, especially in multi-threaded applications, to prevent potential race conditions or unexpected behavior during clipboard operations.
User Feedback
Provide clear and concise feedback to users, confirming successful clipboard operations or notifying them of any errors.
Cross-Platform Compatibility
Ensure that clipboard functionality is compatible across different operating systems, adhering to Java’s write-once-run-anywhere principle.
Clipboard Ownership
When using the ClipboardOwner
interface, handle ownership changes appropriately to maintain control over clipboard interactions.
Resource Cleanup
Dispose of resources, such as Clipboard instances, properly to prevent memory leaks and ensure efficient resource utilization.
Integration with UI Frameworks
Adapt clipboard operations to seamlessly integrate with the chosen UI framework, such as JavaFX, for a cohesive user experience.
Testing and Validation
Thoroughly test clipboard functionality in various scenarios to validate its reliability and consistency across different use cases.
Conclusion
Mastering clipboard functionality in Java is pivotal for creating efficient and user-friendly applications. We explored various methods to copy text to the clipboard, each catering to different needs.
The use of the Clipboard
and StringSelection
classes provides a standard and versatile approach suitable for desktop applications. Introducing the ClipboardOwner
interface enhances flexibility by allowing dynamic responses to ownership changes, offering a more interactive experience.
In JavaFX applications, simplicity and graphical integration shine, making it an ideal choice for scenarios where a visually intuitive interface is crucial. Whether through standard Java or JavaFX, understanding these methods empowers developers to seamlessly implement clipboard interactions, ultimately contributing to a smoother and more engaging user experience in Java applications.