Command Line Parsing in Java
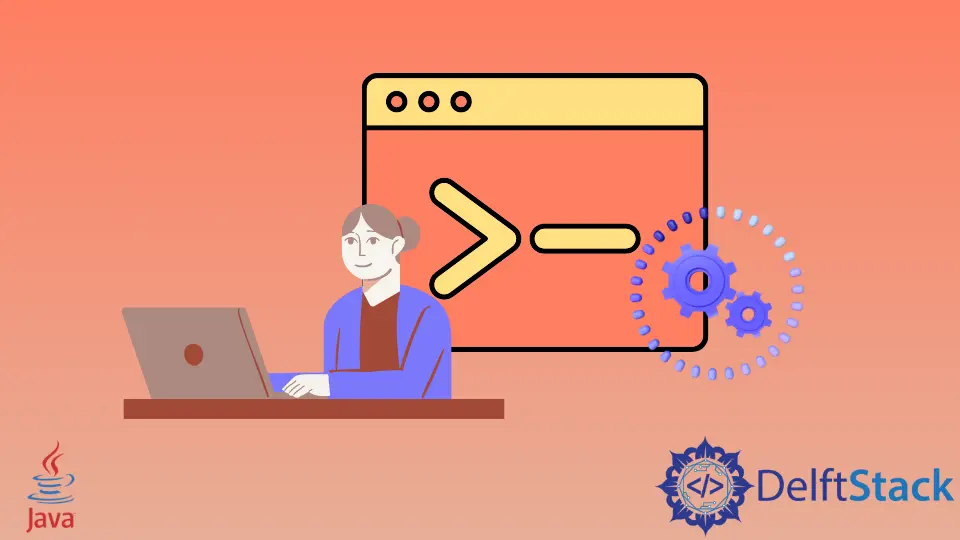
This article introduces how to execute command-line parsing in java.
Command-Line Parsing in Java
Sometimes, to execute Java programs, we require some basic inputs before we run the program. Usually, these inputs are provided by command line arguments. In java, command-line arguments are saved in String[] args
inside the main()
function.
In order to accept arguments from the terminal/command prompt, we need to define Options in the Java program. Every command-line argument you pass needs to be stored in Options. In the code example down below, the two options created implicate the phenomenon of copy and pasting. See the explanation.
import org.apache.commons.cli.*;
public class Main {
public static void main(String[] args) throws Exception {
Options options = new Options(); // Options Arguments which are Acceptable By Program.
Option source = new Option("s", "source", true, "source file path");
source.setRequired(true);
options.addOption(source);
Option destination = new Option("d", "Destination", true, "Destination file Path");
destination.setRequired(true);
options.addOption(destination);
CommandLineParser parser = new BasicParser();
// use to read Command Line Arguments
HelpFormatter formatter = new HelpFormatter(); // // Use to Format
CommandLine cmd = null;
try {
cmd = parser.parse(
options, args); // it will parse according to the options and parse option value
} catch (ParseException e) {
System.out.println(e.getMessage());
formatter.printHelp("utility-name", options);
System.exit(1);
}
String argument1 = cmd.getOptionValue("source");
String argument2 = cmd.getOptionValue("Destination");
System.out.println(argument1);
System.out.println(argument2);
}
}
There are two options created in the above program. One is the source, and the other is the destination. While creating the source option, we assigned its short-term argument as s
and named its source. The s
is a command which gets the value of source arguments. When running the program, the user must use -s
as a command followed by its value. See the output down below. We also set the requirement to true and gave the description as a source file path. Later we added this source option argument inside Options. Similarly, with the option destination, we performed the same thing featuring different values.
When it comes to parsing these two options, we created an object of CommandLineParser
. With the help of HelpFormatter
, we formatted the command arguments if we needed to display the exception.
Inside the try...catch
method, using the parse()
for parsing featuring the parameters options and args
. Then we simply get the option’s values using getOptionValue()
and pass the name of options inside parenthesis.
We are simply printing the values. On the other hand, you can run different programs using this approach.
To run the program, we have to use the following commands.
javac
- cp 'org.apache.commons.cli-1.2.0.jar' Main.java
java
- cp 'org.apache.commons.cli-1.2.0.jar' Main.java - s 'D://textfile.txt'
- d 'D://DuplicatFolder//'
Here, -s
and -d
are the commands. They both are followed by their values, respectively. For instance, in the above program, we will put source path for s
and destination for d
.
The above program will give the following output.
D://textfile.txt
D://DuplicatFolder//
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn