How to Generate All Possible Combinations in Java
- Use Recurrence to Generate All Possible Combinations in Java
- Use Include-Exclude to Generate All Possible Combinations in Java
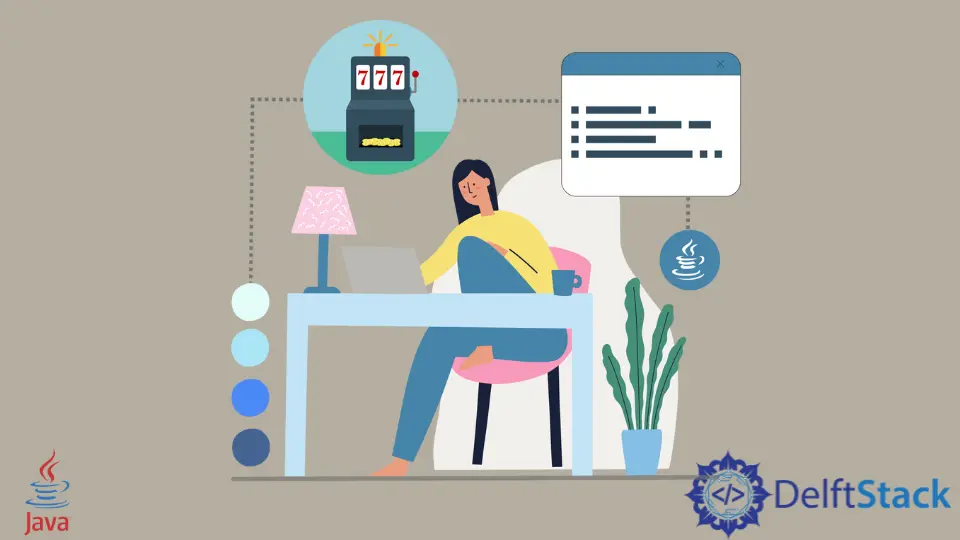
This tutorial demonstrates how to generate all possible combinations of the elements of an array in Java.
Use Recurrence to Generate All Possible Combinations in Java
First, we create an empty array that will store the outputs. The idea is to fix elements one by one and then use recurrence.
Finally, when the number of elements in the initial array becomes equal to the size of combinations, then we print the initial array. Let’s try to implement it in Java.
package delftstack;
import java.io.*;
public class Possible_Combinations {
static void CombinationPossible(int Input_Array[], int Empty_Array[], int Start_Element,
int End_Element, int Array_Index, int r) {
// Current combination is ready to be printed, print it
if (Array_Index == r) {
for (int x = 0; x < r; x++) {
System.out.print(Empty_Array[x] + " ");
}
System.out.println("");
return;
}
for (int y = Start_Element; y <= End_Element && End_Element - y + 1 >= r - Array_Index; y++) {
Empty_Array[Array_Index] = Input_Array[y];
CombinationPossible(Input_Array, Empty_Array, y + 1, End_Element, Array_Index + 1, r);
}
}
static void Print_Combination(int Input_Arrary[], int n, int r) {
int Empty_Array[] = new int[r];
CombinationPossible(Input_Arrary, Empty_Array, 0, n - 1, 0, r);
}
public static void main(String[] args) {
int Input_Array[] = {10, 30, 50, 70, 90, 100};
int r = 3;
int n = Input_Array.length;
Print_Combination(Input_Array, n, r);
}
}
The code above will generate all the possible combinations of the given array in the form of three numbers. See output:
10 30 50
10 30 70
10 30 90
10 30 100
10 50 70
10 50 90
10 50 100
10 70 90
10 70 100
10 90 100
30 50 70
30 50 90
30 50 100
30 70 90
30 70 100
30 90 100
50 70 90
50 70 100
50 90 100
70 90 100
Use Include-Exclude to Generate All Possible Combinations in Java
Similarly, we create an empty array and use the Pascal identity problem to generate all the possible combinations of an array.
In this method, we consider the elements of the given array and recure using the two cases. The first case is the element included in the current combination.
The second case is that element is excluded in the current combination. Let’s try to implement this method in Java.
package delftstack;
import java.io.*;
public class Possible_Combinations {
static void PossibleCombinations(
int Input_Array[], int n, int Length, int Array_Index, int Empty_Array[], int x) {
if (Array_Index == Length) {
for (int y = 0; y < Length; y++) System.out.print(Empty_Array[y] + " ");
System.out.println("");
return;
}
if (x >= n)
return;
Empty_Array[Array_Index] = Input_Array[x];
PossibleCombinations(Input_Array, n, Length, Array_Index + 1, Empty_Array, x + 1);
PossibleCombinations(Input_Array, n, Length, Array_Index, Empty_Array, x + 1);
}
static void Print_Combination(int Input_Array[], int n, int Length) {
int Empty_Array[] = new int[Length];
PossibleCombinations(Input_Array, n, Length, 0, Empty_Array, 0);
}
public static void main(String[] args) {
int Input_Array[] = {10, 30, 50, 70, 90, 100};
int Length = 3;
int n = Input_Array.length;
Print_Combination(Input_Array, n, Length);
}
}
The code above will generate all the possible combinations of the given array in the form of three numbers. See output:
10 30 50
10 30 70
10 30 90
10 30 100
10 50 70
10 50 90
10 50 100
10 70 90
10 70 100
10 90 100
30 50 70
30 50 90
30 50 100
30 70 90
30 70 100
30 90 100
50 70 90
50 70 100
50 90 100
70 90 100
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook