The Char equals Method in Java
-
Check Equal Char Using the
==
Equal Operator in Java -
Check Equal Char Using the
equals()
Method in Java -
Check Equal Char Using the
compare()
Method in Java -
Check Equal Char Using the
equalsIgnoreCase()
Method in Java -
Check Equal Char Using the
compareToIgnoreCase()
Method in Java - Conclusion
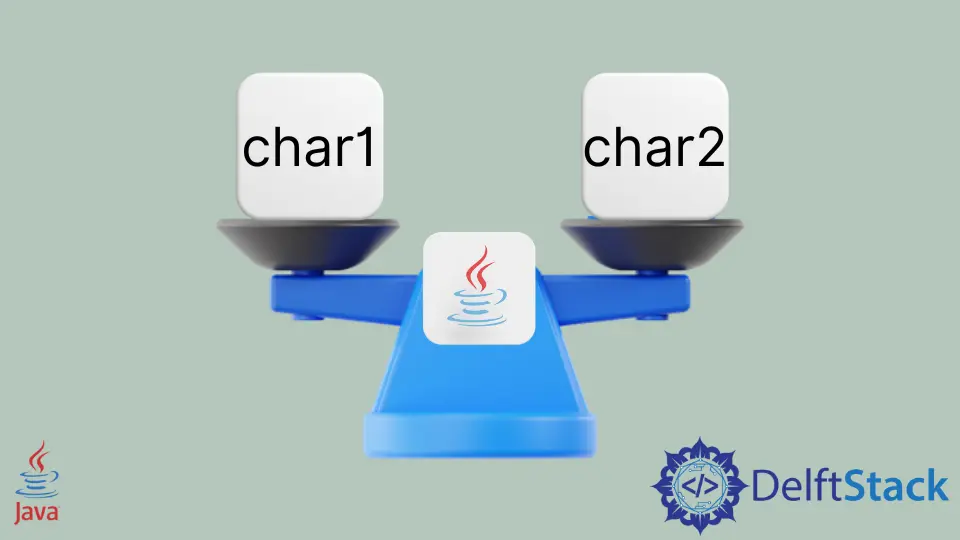
Character equality is a fundamental aspect of programming, and in Java, developers have multiple methods at their disposal to check whether two characters are equal or not.
This tutorial provides a comprehensive discussion of various approaches, offering flexibility based on specific requirements.
Let’s dive into the different methods available in Java for character comparison.
Check Equal Char Using the ==
Equal Operator in Java
In Java, checking whether two characters are equal can be achieved using the ==
equal operator.
This is a binary operator that tests for equality between two operands. When applied to primitive types, such as char
, it compares the actual values of the characters.
If the binary values of the characters are identical, the operator returns true
, indicating equality; otherwise, it returns false
. This direct comparison makes it a straightforward method for checking character equality in Java.
Let’s walk through an example that demonstrates how to use the ==
equal operator to check if two characters are equal or not.
public class CharEqualityExample {
public static void main(String[] args) {
char char1 = 'A';
char char2 = 'B';
boolean areEqual = (char1 == char2);
System.out.println("Are the characters equal? " + areEqual);
}
}
In this example, we begin by declaring two characters, char1
and char2
, which we want to compare. Next, we use the ==
equal operator to check whether the characters are equal.
In the line boolean areEqual = (char1 == char2);
, the ==
operator evaluates the equality of char1
and char2
. If the characters are equal, areEqual
is set to true
; otherwise, it is set to false
.
The output of the code will depend on the values assigned to char1
and char2
. If the characters are equal, the output will be:
Are the characters equal? true
If the characters are not equal, the output will be:
Are the characters equal? false
This illustrates a simple and effective way to check character equality in Java using the ==
equal operator.
Check Equal Char Using the equals()
Method in Java
When working with objects, including the Character
class, the ==
operator might not behave as expected due to the nature of objects and their references. In such cases, the equals()
method comes in handy.
The equals()
method is part of the Object
class, and it’s overridden in the Character
class to compare the actual content of two Character
objects.
The equals()
method is invoked on one object and takes another object as an argument for comparison. For characters, it checks if the Unicode values of the characters are the same.
The syntax is as follows:
boolean equals(Object obj);
If the characters are equal, it returns true
; otherwise, it returns false
. Using equals()
is particularly useful when dealing with object instances rather than primitive values.
Let’s see an example that illustrates the usage of the equals()
method.
public class CharEqualityExample {
public static void main(String[] args) {
Character char1 = 'A';
Character char2 = 'B';
boolean areEqual = char1.equals(char2);
System.out.println("Are the characters equal? " + areEqual);
}
}
Here, we declare two Character
objects, char1
and char2
, representing the characters we want to compare.
Then, in the line boolean areEqual = char1.equals(char2);
, the equals()
method is invoked on char1
, comparing it to char2
. If the characters are equal, areEqual
is set to true
; otherwise, it is set to false
.
Again, the output of the code will depend on the values assigned to char1
and char2
. For this specific example, the output will be:
Are the characters equal? false
Check Equal Char Using the compare()
Method in Java
Another method to check whether two characters are equal is by using the compare()
method, which is part of the Character
class. The compare()
method compares two characters based on their Unicode values.
It returns an integer value 0
if the characters are equal, a positive value if the first character is greater, and a negative value if the second character is greater.
The syntax for the compare()
method is as follows:
int compare(char x, char y);
The method takes two characters, x
and y
, as arguments and returns an integer value indicating their relationship. In the context of checking for equality, we can use the fact that compare()
returns 0
when the characters are equal.
Let’s explore a practical example demonstrating the usage of the compare()
method to check whether two characters are equal or not.
public class CharEqualityExample {
public static void main(String[] args) {
char char1 = 'A';
char char2 = 'A';
int result = Character.compare(char1, char2);
System.out.println("Are the characters equal? " + (result == 0));
}
}
After initializing the two characters, char1
and char2
, that we want to compare, the compare()
method is used to check for equality.
In the line int result = Character.compare(char1, char2);
, the compare()
method is invoked with char1
and char2
as arguments. If the characters are equal, the result
is set to 0
.
We then use (result == 0)
to determine if the characters are equal and print the result accordingly.
Here, the output will be:
Are the characters equal? true
Check Equal Char Using the equalsIgnoreCase()
Method in Java
When dealing with characters and the need for a case-insensitive comparison, the equalsIgnoreCase()
method comes to the rescue. This method is particularly useful when you want to check whether two characters are equal regardless of their case.
Unlike the standard equals()
method, equalsIgnoreCase()
ignores case differences, making it a valuable tool for scenarios where case sensitivity is not a concern.
The equalsIgnoreCase()
method is a part of the String
class but can be used with characters indirectly by converting them to strings. It is ideal for scenarios where you want to consider A
and a
(or any other uppercase-lowercase pair) as equal.
Let’s explore a practical example to understand how to use the equalsIgnoreCase()
method to check whether two characters are equal in a case-insensitive manner.
public class CharEqualityExample {
public static void main(String[] args) {
char char1 = 'A';
char char2 = 'a';
boolean areEqual = String.valueOf(char1).equalsIgnoreCase(String.valueOf(char2));
System.out.println("Are the characters equal? " + areEqual);
}
}
In this example, we initialize two characters, char1
and char2
, representing an uppercase and a lowercase. To perform a case-insensitive comparison, we convert these characters to strings using String.valueOf()
and then utilize the equalsIgnoreCase()
method.
The line boolean areEqual = String.valueOf(char1).equalsIgnoreCase(String.valueOf(char2));
creates a string representations of char1
and char2
using String.valueOf()
. We then use equalsIgnoreCase()
to compare these strings, ensuring a case-insensitive comparison.
The output of the code will be:
Are the characters equal? true
This result shows the case-insensitive nature of the equalsIgnoreCase()
method. It provides a solution for scenarios where case sensitivity is not desired.
Check Equal Char Using the compareToIgnoreCase()
Method in Java
In Java, when you need to perform a case-insensitive comparison between characters, the compareToIgnoreCase()
method offers a convenient solution. This method is part of the String
class but can be applied to characters by converting them to strings.
The compareToIgnoreCase
method is used to compare two strings lexicographically, ignoring their case differences. This makes it a valuable tool in scenarios where case sensitivity is not desired.
It returns an integer that indicates the difference between the ASCII values of the characters in the strings. If the result is 0
, the strings are considered equal.
Let’s explore a practical example to understand how to use the compareToIgnoreCase
method to check whether two characters are equal in a case-insensitive manner.
public class CharEqualityExample {
public static void main(String[] args) {
char char1 = 'A';
char char2 = 'a';
int result = String.valueOf(char1).compareToIgnoreCase(String.valueOf(char2));
boolean areEqual = (result == 0);
System.out.println("Are the characters equal? " + areEqual);
}
}
In this example, to perform a case-insensitive comparison, we convert the characters char1
and char2
to strings using String.valueOf
. We then use the compareToIgnoreCase
method to obtain the result of the comparison, which is stored in the integer variable result
.
Next, we check if the result is 0
to determine if the characters are equal, and the boolean variable areEqual
holds this result.
The output of the code will be:
Are the characters equal? true
This result indicates that the compareToIgnoreCase
method considers A
and a
as equal, providing a solution for scenarios where case sensitivity is not desired.
Conclusion
Choosing the appropriate method for character equality in Java depends on the specific needs of your application. Whether you’re comparing primitive characters or Character
class instances, understanding the strengths and considerations of each method empowers you to make informed decisions.
This article has equipped you with the approaches to determine character equality in various scenarios, ensuring accurate and efficient comparisons in your Java applications.