Bit Mask Operations in Java
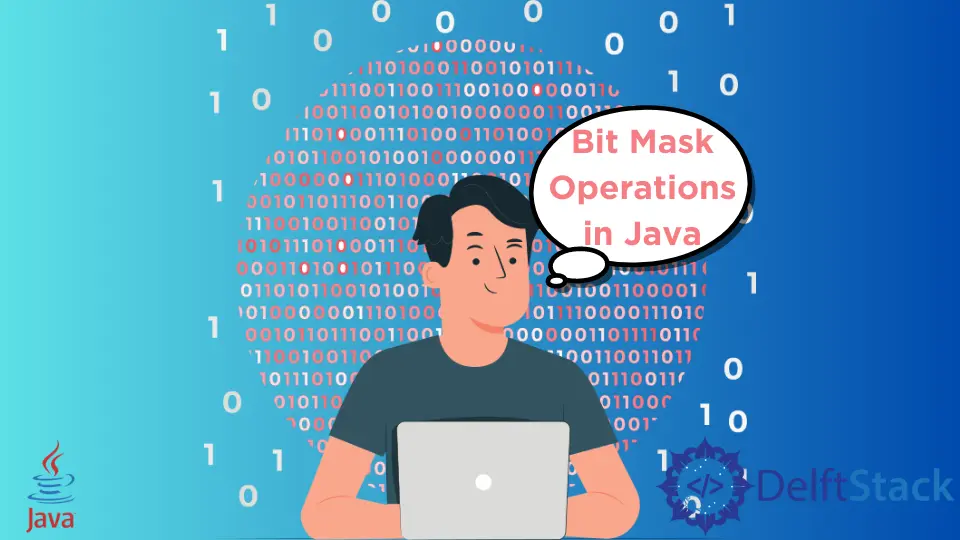
The bit mask is the binary representation of some data. In bit masking, we consider any data just some binary digits like 0s and 1s.
We can also use these binary digits as Booleans since 1
represents true
and 0
represents false
.
Bit Mask Operations in Java
Another definition of bit masking is visualizing a number or other data in binary representation. Some bits are set
and others are unset
where set
means true
or 1
and unset
means false
or 0
.
Let’s say we have a binary value 10011
. Here, the 1st, 4th, and 5th bits are sets
while the 2nd and 3rd are unsets
.
Let’s understand bit mask through an example. Say we have a set of four characters {w,x,y,z}
, and we want a different representation of the character string.
To create a mask of the 4 bits {_ _ _ _}
, each bit gives us the information whether we are referring to that character or not. To make it more simple, the binary combination 1001
means w,z
, 0000
means an empty string, and 1111
means w, x, y, z
.
Now that we have understood bits and bit masking, it’s time to learn about bitwise operators used to manipulate bits.
Bitwise Operators in Java
Java bitwise operators are utilized to perform the manipulation of bits. These operators can be used in any integral type like short, byte, long, char, int, etc.
We have different bitwise operators. Each of them is used for a different purpose.
These are the following:
- Bitwise AND (
&
) - Bitwise OR (
|
) - Bitwise Complement (
~
) - Bitwise XOR (
^
)
Bitwise AND (&
) Operator in Java
The AND operator is a bitwise operator denoted by &
. It does bit by bit calculation of two or more values and returns true
if both values are true
; otherwise, false
.
1(True) & 1(True) = 1(True)
0(False) & 0(False) = 0(False)
1(True) & 0(False) = 0(False)
0(False) & 1(True) = 0(False)
Example code:
package articlecodesinjava;
public class ArticleCodesInJava {
public static void main(String[] args) {
int a = 3;
int b = 4;
// bitwise AND
// 0011 & 0100=0000 = 0
System.out.println("a & b = " + (a & b));
}
}
Output:
a & b = 0
Bitwise OR (|
) Operator in Java
The OR operator is also a bitwise operator denoted by |
, but it is different from the AND (&
) operator. It returns true
if and only if a single value is true
; otherwise, false
.
1(True) | 1(True) = 1(True)
0(False) | 0(False) = 0(False)
1(True) | 0(False) = 1(True)
0(False) | 1(True) = 1(True)
Example code:
package articlecodesinjava;
public class ArticleCodesInJava {
public static void main(String[] args) {
int a = 3;
int b = 4;
// bitwise OR
// 0011 & 0100=0000 = 7
System.out.println("a | b = " + (a | b));
}
}
Output:
a & b = 7
Bitwise Complement (~
) Operator in Java
The bitwise complement (~
) operator, also known as the NOT
operator, can invert a bit pattern. It is a unary operator because it is used with a single value.
Suppose we have a binary number, 10110
, and we want to negate this with the bitwise complement (~
) operator. It becomes 01001
.
It has inverted 0s into 1s and 1s into 0s.
~ 1(True) = 0(False)
~ 0(False) = 1(True)
Example code:
package articlecodesinjava;
public class ArticleCodesInJava {
public static void main(String[] args) {
// Initial values
int a = 3, b;
// bitwise complement of a
// a = 011 where b = ~a, b =100
b = ~a;
System.out.println("~a = " + b);
}
}
Output:
~a = -4
Bitwise XOR (^
) Operator in Java
The Java bitwise XOR operator is a binary operator denoted by ^
.
It returns true
if the input binary values are both different. However, if the input values are the same, either 0s or 1s, the result is false
.
1(True) ^ 1(True) = 0(False)
0(False) ^ 0(False) = 0(False)
1(True) ^ 0(False) = 1(True)
0(False) ^ 1(True) = 1(True)
Example code:
package articlecodesinjava;
public class ArticleCodesInJava {
public static void main(String[] args) {
// Initial values
int a = 3;
int b = 4;
// bitwise xor
// 0011 ^ 0100 = 0111 = 7
System.out.println("a ^ b = " + (a ^ b));
}
}
Output:
a ^ b = 7
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn