What Is Java Bean
- Create JavaBeans in Java
- How to Implement/Access JavaBeans in Java
- Setters and Getters of JavaBeans in Java
- Advantages of JavaBean
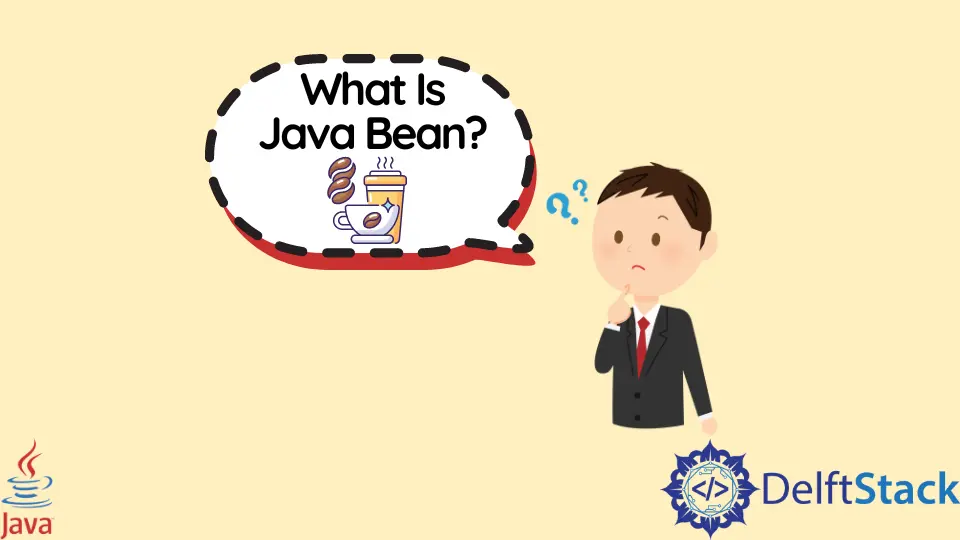
This tutorial introduces what is bean in Java and also lists some example codes to understand the topic.
JavaBeans are classes that encapsulate many objects into a single object (the bean). It has the following properties.
- import Serializable Interface
- private fields
- constructors
getter/setter
The serializability of a class is enabled by the class implementing the java.io.Serializable
interface. Classes that do not implement this interface will not have any of their state serialized or deserialized.
Fields should be private to prevent outer classes from modifying those fields easily. Instead of directly accessing those fields, usually, getter/setter methods are used.
It may have zero or more argument constructors to create an object.
It has getters and setters methods for accessing and modifying private fields.
This is the basic structure or draft of JavaBean in Java.
// Implements Serializable interface
public class SimpleTesting implements Serializable {
// private fields
// no-args constructor
// list of getters and setters
}
Create JavaBeans in Java
Here, we created a Javabean class SimpleTesting
with three private fields, one default constructor, and one parameterized constructor and getters and setters to set and get data. See the example below.
import java.io.Serializable;
public class SimpleTesting implements Serializable {
private int id;
private String name;
private int salary;
public SimpleTesting() {}
public SimpleTesting(int id, String name, int salary) {
this.id = id;
this.name = name;
this.salary = salary;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getSalary() {
return salary;
}
public void setSalary(int salary) {
this.salary = salary;
}
}
How to Implement/Access JavaBeans in Java
To implement JavaBeans, we can create an object of that class. Here, we passed values to the constructor and access values using getters. See the example below.
public class Main {
public static void main(String[] args) {
SimpleTesting st = new SimpleTesting(1, "Rohan", 100000);
System.out.println("id = " + st.getId());
System.out.println("name = " + st.getName());
System.out.println("salary = " + st.getSalary());
}
}
Output:
id = 1
name = Rohan
salary = 100000
Setters and Getters of JavaBeans in Java
Here, we are using setters methods to set values and then getter methods to get values. This is the strength of JavaBeans. See the example below.
public class Main {
public static void main(String[] args) {
SimpleTesting st = new SimpleTesting();
st.setId(1);
st.setName("Rohan");
st.setSalary(100000);
System.out.println("id = " + st.getId());
System.out.println("name = " + st.getName());
System.out.println("salary = " + st.getSalary());
}
}
Output:
id = 1
name = Rohan
salary = 100000
Advantages of JavaBean
Java Beans are used throughout Java EE as a universal contract for runtime discovery and access. For example, JavaServer Pages (JSP) uses Java Beans as data transfer objects between pages or between servlets and JSPs.