How to Show Animated GIF in Java
- Method 1: Using Java Swing
- Method 2: Using JavaFX
- Method 3: Using Third-Party Libraries
- Conclusion
- FAQ
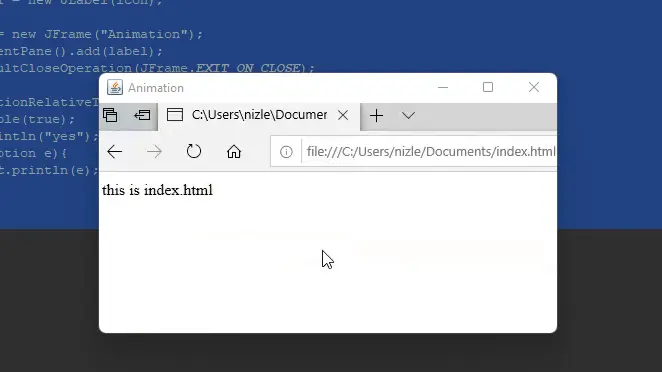
In the world of programming, visual elements like animated GIFs can significantly enhance user experience. If you’re a Java developer looking to incorporate animated GIFs into your applications, you’re in the right place.
This article will guide you through the process of displaying animated GIFs in Java, providing you with practical examples and insights. Whether you’re developing a desktop application or a web-based interface, understanding how to effectively use animated GIFs can elevate your project. So, let’s dive into the methods and see how you can bring your Java applications to life with animated visuals!
Method 1: Using Java Swing
Java Swing is a popular GUI toolkit for Java applications. It provides a straightforward way to display images, including animated GIFs. To show an animated GIF, you can utilize the JLabel
component, which allows you to easily add images to your user interface.
Here’s how you can do it:
import javax.swing.*;
import java.awt.*;
public class AnimatedGifExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Animated GIF Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 300);
ImageIcon gif = new ImageIcon("path/to/your/animated.gif");
JLabel label = new JLabel(gif);
frame.getContentPane().add(label);
frame.setVisible(true);
}
}
Output:
In this example, we first import the necessary Swing classes. We create a JFrame
to serve as the main window and set its default close operation and size. Next, we load the animated GIF using ImageIcon
, providing the path to your GIF file. We then create a JLabel
to hold the GIF and add it to the frame’s content pane. Finally, we set the frame visible to display the animated GIF. This method is efficient and straightforward, making it a great choice for Java developers.
Method 2: Using JavaFX
JavaFX is another powerful framework for building rich desktop applications in Java. If you prefer a more modern approach, JavaFX provides a robust way to display animated GIFs with its ImageView
class.
Here’s how to implement it:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class AnimatedGifJavaFX extends Application {
@Override
public void start(Stage primaryStage) {
Image gif = new Image("file:path/to/your/animated.gif");
ImageView imageView = new ImageView(gif);
StackPane root = new StackPane();
root.getChildren().add(imageView);
Scene scene = new Scene(root, 300, 300);
primaryStage.setTitle("Animated GIF in JavaFX");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Output:
In this JavaFX example, we extend the Application
class and override the start
method. We load the animated GIF using the Image
class, specifying the file path. An ImageView
is then created to display the GIF. We use a StackPane
layout to add the ImageView
to the scene. Finally, we set up the primary stage with the scene and show it. JavaFX is particularly advantageous for modern applications, offering a more flexible and visually appealing framework than Swing.
Method 3: Using Third-Party Libraries
If you want more control over GIF playback or need advanced features, consider using third-party libraries like GifView
. These libraries can simplify the process of displaying GIFs and provide additional functionality.
Here’s a simple implementation using a popular library:
import javax.swing.*;
import com.jhlabs.image.GifImage;
public class GifViewExample {
public static void main(String[] args) {
JFrame frame = new JFrame("GIF View Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 300);
GifImage gif = new GifImage("path/to/your/animated.gif");
JLabel label = new JLabel(new ImageIcon(gif.getImage()));
frame.getContentPane().add(label);
frame.setVisible(true);
}
}
Output:
In this example, we utilize the GifImage
class from a third-party library that handles GIFs efficiently. Similar to the previous methods, we create a JFrame
and load the GIF using the library. The ImageIcon
is used to wrap the image, which is then added to a JLabel
. This approach is particularly useful when you need more advanced features such as controlling playback speed or looping options. Make sure to include the library in your project dependencies to use this method effectively.
Conclusion
Incorporating animated GIFs into your Java applications can significantly enhance user engagement. Whether you choose Java Swing, JavaFX, or a third-party library, each method has its unique advantages. By following the examples provided, you can easily display animated GIFs and elevate your application’s visuals. Experiment with these methods and see which one fits your project best. Happy coding!
FAQ
-
How do I load a GIF from a URL in Java?
You can use theImageIO
class to read a GIF from a URL, similar to loading it from a file. -
Can I control the playback of GIFs in Java?
Yes, using third-party libraries allows you to control playback features like speed and looping.
-
What is the best method to display GIFs in a Java web application?
For web applications, consider using JavaFX or HTML5 with Java backend to display GIFs effectively. -
Are there performance concerns when using animated GIFs in Java?
Yes, large GIFs can impact performance, so optimizing the file size is recommended. -
Can I use animated GIFs in Java console applications?
No, animated GIFs require a graphical user interface, which console applications do not support.