Java でアニメーション GIF を表示する
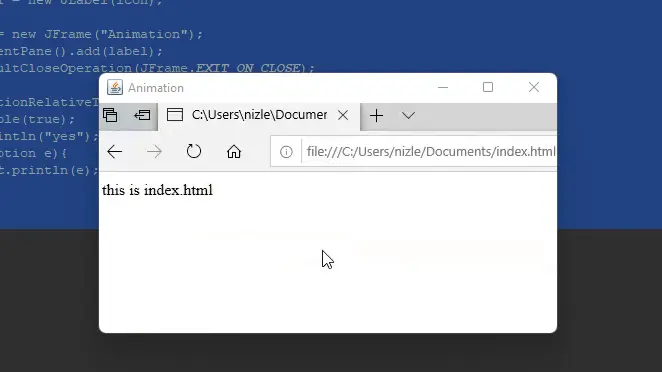
javax
パッケージの Swing
ライブラリ メソッドを使用して、Java でアニメーション GIF を表示できます。 この記事では、ユーザーが Java アプリケーションまたは別のウィンドウでアニメーション GIF を表示する方法を紹介します。
Javax.swing
ライブラリを使用して Java でアニメーション GIF を表示する
以下のコード例では、必要なライブラリをインポートしています。 javax.swing
ライブラリの jLabel
および jFrame
クラスを使用します。
また、Java.net
ライブラリの URL
クラスを使用しています。 URL から GIF を読み取るために、URL
クラスのオブジェクトを作成し、GIF の場所の URL を引数として渡しました。
その URL から、ImageIcon
クラスのオブジェクトを使用してイメージ アイコンを作成しました。
次に、Imageicon
から jLabel
を作成しました。 次に、jLabel
を表示するフレームを作成します。
その後、フレームに label
を追加して GIF を表示しました。 最後に、frame.setVisible(true)
を使用して visible
を true に設定しました。
コード例:
// import required libraries
import java.net.*;
import javax.swing.*;
public class TestClass {
public static void main(String[] args) {
try {
// create a new URL from the image URL
URL ImageUrl =
new URL("https://www.delftstack.com/img/HTML/page%20redirects%20to%20index.gif");
// Create image icon from URL
Icon imageIcon = new ImageIcon(ImageUrl);
// Create a new JLabel from the icon
JLabel label = new JLabel(imageIcon);
// Create a new JFrame to append the icon
JFrame frame = new JFrame("Animation");
// add a label to JFrame
frame.getContentPane().add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
// Show the JFrame
frame.setVisible(true);
} catch (Exception e) {
System.out.println(e);
}
}
}
出力:
上記の出力では、アニメーション GIF を示す新しいウィンドウ ポップアップが表示されます。
また、ユーザーがローカル コンピューターから GIF を表示したい場合は、次のコードに示すように、ImageIcon
オブジェクトを初期化するときに GIF のパスを指定できます。
Icon imageIcon = new ImageIcon("<path of GIF from local computer");
ローカル コンピューターから GIF を表示する場合は、URL
クラス オブジェクトを作成する必要はありません。
さらに、ユーザーは、GIF のウィンドウ フレームのサイズをカスタマイズし、必要に応じてフレームにカスタム ラベルを設定できます。