How to Align Text in Java
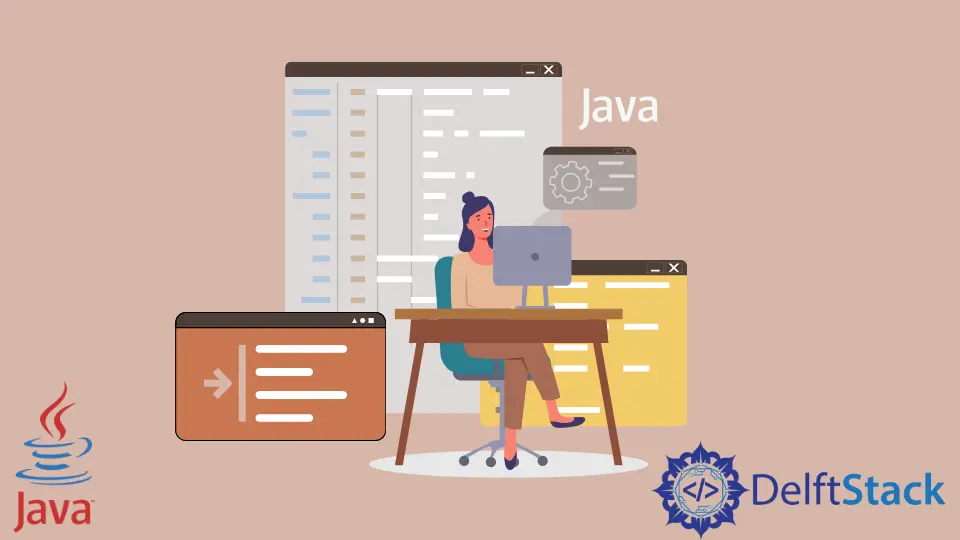
We can create our class, which will extend the class Java.text.Format
to align the text.
This tutorial demonstrates how to align text in Java.
Align Text in Java
The Format
is an abstract base class to format sensitive information like numbers, messages, dates, etc. We will implement our class named Text_Alignment
, which will extend the Format
class so we can format the text to align it.
This class will define three enums, Center
, Right
, and Left
, later used in a switch
condition as cases to align text according to the given instructions. This class uses a maximum number of characters for one line and then aligns each line.
See the example.
package delftstack;
import java.text.FieldPosition;
import java.text.Format;
import java.text.ParsePosition;
import java.util.ArrayList;
import java.util.List;
import java.util.ListIterator;
public class Text_Alignment extends Format {
private static final long serialVersionUID = 1L;
public enum Align_Text {
LEFT,
CENTER,
RIGHT,
}
// justification for formatting
private Align_Text Current_Alignment;
// maximum length of a line
private int Maximum_Chars;
public Text_Alignment(int Maximum_Chars, Align_Text alignment) {
switch (alignment) {
case LEFT:
case CENTER:
case RIGHT:
this.Current_Alignment = alignment;
break;
default:
throw new IllegalArgumentException("invalid justification");
}
if (Maximum_Chars < 0) {
throw new IllegalArgumentException("Maximum_Chars should be positive.");
}
this.Maximum_Chars = Maximum_Chars;
}
public StringBuffer format(
Object Input_Object, StringBuffer Align_Position, FieldPosition Ignore_Position) {
String Demo = Input_Object.toString();
List<String> Strings_List = Split_String(Demo);
ListIterator<String> List_Iterator = Strings_List.listIterator();
while (List_Iterator.hasNext()) {
String Wanted_String = List_Iterator.next();
// put the spaces in the right place.
switch (Current_Alignment) {
case RIGHT:
ALIGN(Align_Position, Maximum_Chars - Wanted_String.length());
Align_Position.append(Wanted_String);
break;
case CENTER:
int toAdd = Maximum_Chars - Wanted_String.length();
ALIGN(Align_Position, toAdd / 2);
Align_Position.append(Wanted_String);
ALIGN(Align_Position, toAdd - toAdd / 2);
break;
case LEFT:
Align_Position.append(Wanted_String);
ALIGN(Align_Position, Maximum_Chars - Wanted_String.length());
break;
}
Align_Position.append("\n");
}
return Align_Position;
}
protected final void ALIGN(StringBuffer Append_To, int Length) {
for (int i = 0; i < Length; i++) Append_To.append(' ');
}
String format(String Demo) {
return format(Demo, new StringBuffer(), null).toString();
}
// ParseObject will be required but it is not useful here.
public Object parseObject(String Source_String, ParsePosition position) {
return Source_String;
}
private List<String> Split_String(String Demo) {
List<String> List = new ArrayList<String>();
if (Demo == null)
return List;
for (int x = 0; x < Demo.length(); x = x + Maximum_Chars) {
int End_Index = Math.min(x + Maximum_Chars, Demo.length());
List.add(Demo.substring(x, End_Index));
}
return List;
}
public static void main(String[] args) {
String Demo_Text =
"DelftStack is a resource for everyone interested in programming, embedded software, and electronics."
+ "It covers the programming languages like Python, C/C++, C#, and so on in this website's first development stage."
+ "Open-source hardware also falls in the website's scope, like Arduino, Raspberry Pi, and BeagleBone."
+ "DelftStack aims to provide tutorials, how-to's, and cheat sheets to different levels of developers and hobbyists.";
// Align Left
Text_Alignment align = new Text_Alignment(50, Align_Text.LEFT);
System.out.println("This is the left alignment of the given text: ");
System.out.println(align.format(Demo_Text));
// Align Right
Text_Alignment align1 = new Text_Alignment(50, Align_Text.RIGHT);
System.out.println("This is the right alignment of the given text: ");
System.out.println(align1.format(Demo_Text));
// Align Center
Text_Alignment align2 = new Text_Alignment(50, Align_Text.CENTER);
System.out.println("This is the center alignment of the given text: ");
System.out.println(align2.format(Demo_Text));
}
}
The code above formats the given text in the Left
, Right
, and Center
alignment. See output:
This is the left alignment of the given text:
DelftStack is a resource for everyone interested i
n programming, embedded software, and electronics.
It covers the programming languages like Python, C
/C++, C#, and so on in this website's first develo
pment stage.Open-source hardware also falls in the
website's scope, like Arduino, Raspberry Pi, and
BeagleBone.DelftStack aims to provide tutorials, h
ow-to's, and cheat sheets to different levels of d
evelopers and hobbyists.
This is the right alignment of the given text:
DelftStack is a resource for everyone interested i
n programming, embedded software, and electronics.
It covers the programming languages like Python, C
/C++, C#, and so on in this website's first develo
pment stage.Open-source hardware also falls in the
website's scope, like Arduino, Raspberry Pi, and
BeagleBone.DelftStack aims to provide tutorials, h
ow-to's, and cheat sheets to different levels of d
evelopers and hobbyists.
This is the center alignment of the given text:
DelftStack is a resource for everyone interested i
n programming, embedded software, and electronics.
It covers the programming languages like Python, C
/C++, C#, and so on in this website's first develo
pment stage.Open-source hardware also falls in the
website's scope, like Arduino, Raspberry Pi, and
BeagleBone.DelftStack aims to provide tutorials, h
ow-to's, and cheat sheets to different levels of d
evelopers and hobbyists.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook