The intValue() Method in Java and Primitive Data Types Conversion
-
the
intValue()
Method in Java -
Difference Between
Int
andInteger
in Java -
String Conversion With the
intValue()
Method in Java
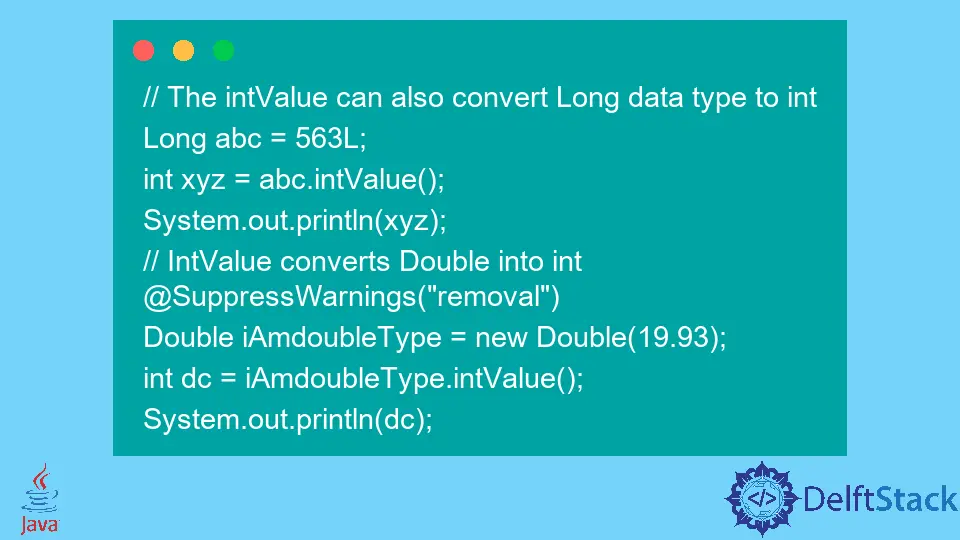
The intValue()
is a method in Java, and the int
is a primitive type in Java. It stores the binary value for the integer in variable type int
while Integer
is a class similar to any other programming language.
Like any other reference object type, Integer
variables contain references to Integer
objects.
the intValue()
Method in Java
Syntax:
public int intvalue();
This method is proper when converting this Integer
into an int
. To be precise, this method returns the numeric value represented by this object after converting it to the type int
.
The following code block does the same.
public class IntValueMethod {
public static void main(String[] args) {
@SuppressWarnings("removal") Integer test = new Integer(5000);
// This object
int converts = test.intValue();
// Returns this Iteger value in int type
System.out.println("The intValue returns the integer value in int " + converts);
}
}
It can work on other primitive data types too. Check the following code examples.
// The intValue can also convert Long data type to int
Long abc = 563L;
int xyz = abc.intValue();
System.out.println(xyz);
// IntValue converts Double into int
@SuppressWarnings("removal") Double iAmdoubleType = new Double(19.93);
int dc = iAmdoubleType.intValue();
System.out.println(dc);
Output:
The intValue returns the integer value in int 5000
563
19
Difference Between Int
and Integer
in Java
Precisely, the Integer
is a class that has only one field of type int
.
This class can be used when you need an int
to act like any other object, such as generic types or objectives.
Difference Example:
public class IntVsInteger {
public static void main(String[] args) {
// This works
int b = 100;
// This will now work
// Integer b = 100;
// Similarly, you can parse integer like:
Integer.parseInt("1");
// But you can not do it like:
// int.parseInt("1");
System.out.println("int b returns: " + b);
}
}
Output:
int b returns: 100
For example, byte=>Byte
, float=>Float
, double=>Double
, int=>Integer
and so on.
String Conversion With the intValue()
Method in Java
The scope of intValue()
depends on one’s requirements. You can solve complex problems with this method in real-time.
But first, you need to sharpen your concepts. Run the following program and then create some of your own.
public class ConvertStringswithIntValue {
@SuppressWarnings({"removal"})
public static void main(String[] args) {
String iAmstring = "4000";
// this integer takes the string and passes it over to the intValue for
// conversion
int iAmint = new Integer(iAmstring).intValue(); // intValue will convert string into int
System.out.println("The string value has successfully been converted to int value: " + iAmint);
}
}
Output:
The string value has successfully been converted to int value: 4000
Aside from this article, if you want to learn more about the strings, refer to this: Char vs String in Java, and if you are interested to learn more about Integers
, refer to this: Java Integer.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn