Introduction to Pointers in Java
- Pointer in Java
- No Pointer in Java
- Alternative: References in Java Language
- Disadvantages of Using References as Pointers in Java
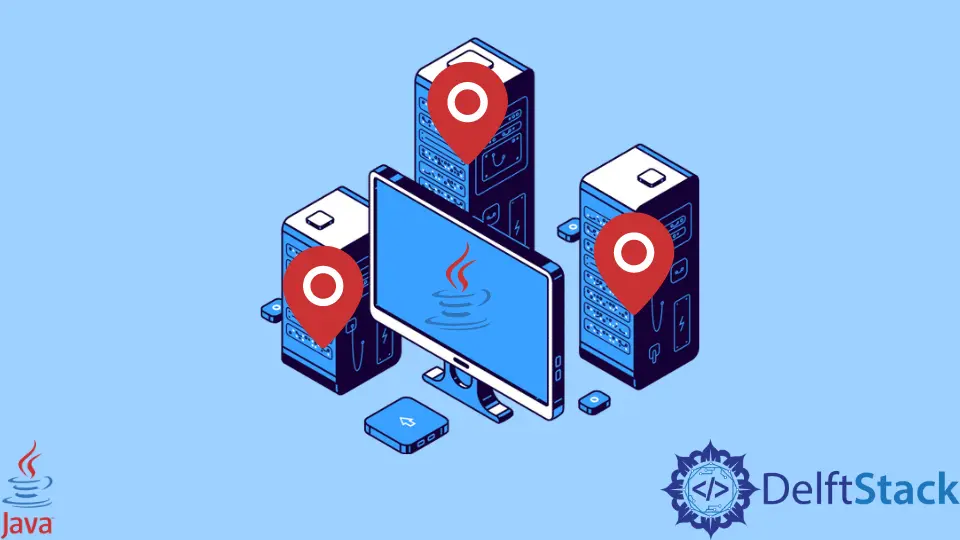
Today, we will learn about pointers in Java and how we can get an advantage by using them, but before that, we will see if they are available in Java; if not, what are the alternatives?
We will answer all these concerns in this article. We will also compare pointers with other languages called C and C++.
Pointer in Java
As we know, pointers are objects that store a memory address. They are handy in address management and memory allocation but only work if a language has pointer arithmetic.
Usually, languages have pointer arithmetic pre-programmed, but that’s not the case with Java. So it might sound a little shocking to some that Java language doesn’t have the concept of pointers.
Instead, the Java language has references as pointers. Remember that the pointers can be called references, but it is not the same the other way around, as references are not pointers.
Let us look into this concept in detail with examples further in this guide.
No Pointer in Java
The majority claim that Java is better off without pointers. It is because of the reasons mentioned below.
-
Arithmetic - As mentioned above, pointers use arithmetic to operate. Furthermore, a pointer works with memory management, which is fundamentally unsafe.
Many would argue otherwise, but it is, for a fact, unsafe to let pointers have access to the memory. For this reason, Java does not support pointer arithmetic. And no extra memory is used.
-
Manipulation - It is easy for users to misuse a pointer; manipulating an object through a pointer is also very risky. All in all, Java does not require pointers in its Object Oriented Programming.
Also, adding a pointer in the Java language would unnecessarily cause complexity to rise.
Alternative: References in Java Language
As mentioned above, the concept of pointers is unavailable in Java. So, instead of pointers, references are used as they work the same as pointers do.
The term pointer is referred to the languages C and C++. In Java, we cannot use pointers, so an alternative method is used called references. As mentioned, references work the same as a pointer, but their usage is slightly different.
When a reference is used, it is done using a function. A reference’s copy is copied to the stack of the function used to call an object and allows manipulation of the object as desired.
Even if users want to use a pointer, they are free to use References. Here is an example that explains the use of Reference in Java.
class Shape {
int length;
int breadth;
}
public class ShapesUse {
public static void main(String args[]) {
/*
Here, an example1 is used as a reference variable.
Which means it holds the address of the Shapes object
*/
Shape example1 = new Shape();
/*
example2 is another reference variable used,
and example2 is initialized with example1
example1 and example2 reference the same object,
so no duplication and extra memory are used.
*/
Shape example2 = example1;
example1.length = 10;
example2.breadth = 26;
System.out.println("length of example 1 is: " + example1.length);
System.out.println("breath of example 1 is: " + example2.breadth);
}
}
In this example, example1
and example2
are used as references. It is because they hold the address of the object shape
, and through this object, length
and breadth
is being called.
Remember that we can not use these references outside of this program. Following is the output of the code above. As we can see, the references work as desired.
Output:
length of example 1 is: 10
breath of example 1 is: 26
Disadvantages of Using References as Pointers in Java
Let us look into some disadvantages of not having a pointer in the Java language.
-
Explicit Use - Although references can be used as pointers, there is a disadvantage. We cannot use references explicitly. We cannot use these pointers outside the Java program’s scope.
Any action performed using references is implicit, so they are not visible. It is quite a disadvantage as pointers are used to access the resources, which we can do explicitly.
In C programming, we can subtract or add a pointer’s address to point to things. However, in Java, a reference can only point to one thing.
-
Strict Controlled - References are much more strictly controlled than C or C++. Unfortunately, this means that type casting is not available.
However, in pointers, we can type cast, for example,
int
, tochar
. But in Java, references can only point to an object of the same type. So, for example, we can only use astring
reference for an object of thestring
type.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn