Introduction to Integration Testing in Java
- Introduction to Integration Testing
- Importance of Integration Testing
- Types of Integration Testing
- Required Steps for Integration Testing
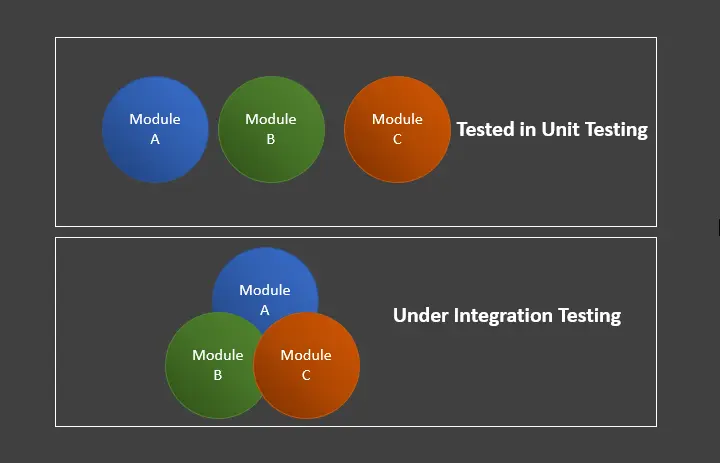
This tutorial introduces integration testing and highlights how we can differentiate it from unit testing. Further, it discusses various types of integration testing, considering their pros and cons.
Then, we will learn about the required steps to perform integration testing, followed by a real-world scenario to understand integration testing.
Introduction to Integration Testing
There are three types of testing in testing pyramid: unit testing, integration testing, and functional testing. This article will revolve around integration testing, which means we check if various modules work as expected when we combine them as a group.
Remember, software consists of various modules that multiple programmers have coded. Here, the primary aim of integration testing is to test an interface between two or multiple software modules by integrating them logically and testing them as a group.
Further, it determines the correctness of an interface by exposing defects in an interaction between various software components when we integrate them. The integration testing process starts once all software components have undergone unit testing.
See the following to visualize unit testing and integration testing.
Following are a few points to highlight how integration testing differs from unit testing.
Unit Testing | Integration Testing |
---|---|
Here, the developer is well aware of the software’s internal design. | Here, the tester does not know the software’s internal design. |
Every module is tested separately. | We combine all modules and test as a group. |
It is called white-box testing. | It is known as black-box testing. |
It is done by a developer. | It is done by a tester. |
Finding defects is easy here. | Exposing defects is difficult in integration testing. |
In unit tests, all project components are tested individually and independently without waiting for other components to be completed. | The integration test is performed once all parts are completed. |
It is cost-effective. Maintenance is also less costly. | It is expensive. Maintenance is also costly. |
Module specification is done initially. | Interface specification is performed at the beginning. |
It is all about exploring code in-depth. | It is about detailed visibility of the integration structure. |
Fast execution than integration testing. | Here, speed is comparatively slow due to modules’ integration. |
You can find more on it here.
Importance of Integration Testing
Though every software component is unit tested, defects can still be exposed for various reasons, increasing the importance of integration testing. Some of them are listed below.
- It enables better integration of software modules.
- By using integration testing, we can prevent modifications in data during software module transfers.
- It also overcomes various issues of manual testing.
- Integration testing also enables effective third-party testing.
- It is required to verify that all software components work in a unit and produce an output as expected.
- Finding defects in external hardware interfaces and software modules’ interfaces with a database is useful.
- Sometimes, a client changes the requirements during the software module’s development. In that case, there are chances that new requirements may not be tested at the unit testing level; hence, integration testing becomes essential here.
Types of Integration Testing
This article will discuss two approaches for integration testing, considering the pros and cons.
- Big Bang
- Incremental (further categorized into Top-down, Bottom-up, and Sandwich approaches)
Big Bang Approach for Integration Testing
In this approach, we integrate all software components to test as a unit known as an entity during testing. This integration process will not be executed until all the components in the unit test are completed.
Do not confuse it with system testing. We only test the integration of different software modules, not the whole system (performed in system testing).
Its most significant advantage is that we can integrate all software components and test them as one unit, while it is also hard to identify defects using the big bang approach. Therefore, it is convenient for all small systems.
We can visualize the big bang approach as follows. We have integrated six different modules and tested using the big bang.
Incremental Approach for Integration Testing
Using this approach, we integrate two or more software modules logically related to one another and then test them for the application’s proper functioning. Then, other related modules/components are integrated incrementally and tested.
This procedure continues until all logically related components are integrated and tested.
This approach is further categorized into top-down, bottom-up, and sandwich approaches. Let’s learn each of them below through the Stubs
and Drivers
.
Stub
- It is called by a module/component under test.Driver
- This calls for the module/component to be tested.
Top-Down Approach
Here, integration is performed from top to bottom following the software system’s control flow. Using this approach, we test higher-level components and then move toward lower-level components to check software functionality.
Here, we can use Stubs
if some components are not read yet. It is an organic way consistent with what will happen in the real environment.
Another advantage of this approach is that we can test critical modules on priority. This way, we can find defects on a higher level and fix them first.
On the other hand, testing major functions at the end is the only concern with this approach. We can visualize it in the following diagram.
Bottom-Up Approach
Here, we test lower-level modules first, which will be used to assist in testing the higher-level module. This procedure continues until we test all modules/components at the top level.
In the bottom-up approach, we use stimulator programs known as Drivers
. It is easy to find defects and errors at the lower level, but higher-level problems can only be found at the end when all components have been integrated and tested.
Sandwich Approach
This approach combines top-down and bottom-up approaches, therefore known as Hybrid Integration Testing. It uses Stubs
and Drivers
.
Here, top-level components are tested with lower-level components. At the same time, lower components/modules are integrated with the top-level modules/components and tested as the system.
We can visualize this approach as follows:
Required Steps for Integration Testing
The following steps are required regardless of what software testing strategy (discussed above) is being used:
-
Make an integration test plan.
-
Design test scenarios, cases, and scripts.
-
Execute all test cases, followed by exposing and reporting defects.
-
Keep track and re-test the defects.
-
Steps 3 and 4 are repeated until we accomplish successful integration.
Real World Example to Understand Integration Testing
Suppose we have a method called performPayment()
, which takes two parameters, amount
and service
of type double and PaymentService
, respectively.
In the unit test, we’ll test it by creating a mock for the service
argument, while the integration test will be a test where we use the actual external service
to ensure if that service
responds to our input data as expected.