Idempotent in Java
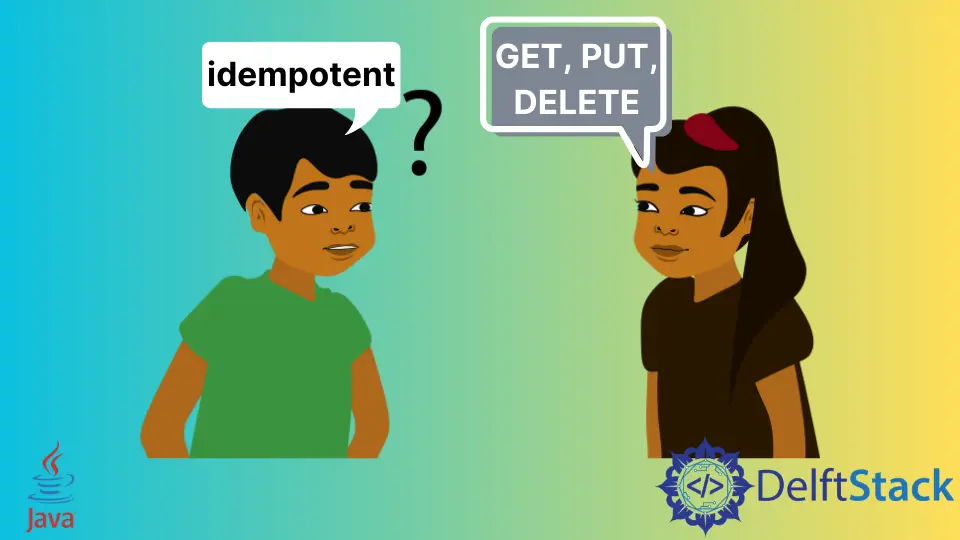
In the world of programming, the term “idempotent” often surfaces, especially when discussing operations in Java. But what does it really mean? Idempotence refers to the property of certain operations that can be applied multiple times without changing the result beyond the initial application. This concept is crucial, especially in APIs and distributed systems, where repeated requests can occur.
In this tutorial, we will explore the significance of idempotent operations in Java, how to implement them, and why they are essential for building robust applications. Whether you’re a seasoned developer or just starting, understanding idempotence will enhance your coding practices and make your applications more resilient.
What is Idempotence in Java?
Idempotence in Java is a concept that applies to methods or operations that can be called multiple times without altering the outcome after the first call. For example, if you have a method that sets a user’s status to “active,” calling that method repeatedly will not change the status beyond the initial activation. This property is particularly important in web services, where users may accidentally send the same request multiple times due to network issues or user actions.
Idempotent operations ensure that even if the same request is made repeatedly, the system state remains consistent. This is vital for maintaining data integrity and providing a reliable user experience. In Java, you can implement idempotence in various ways, including using unique identifiers for requests or checking the current state before performing an operation.
Implementing Idempotent Operations in Java
When working with Java, there are several strategies to implement idempotent operations effectively. Here are a few methods that can be employed:
Using Unique Request Identifiers
One common approach to achieve idempotence is by using unique request identifiers. This method involves generating a unique ID for each operation that can be stored and checked before executing the operation. Here’s a simple example:
import java.util.HashSet;
public class IdempotentService {
private HashSet<String> processedRequests = new HashSet<>();
public String processRequest(String requestId) {
if (processedRequests.contains(requestId)) {
return "Request already processed.";
}
processedRequests.add(requestId);
// Perform the actual operation here
return "Request processed successfully.";
}
}
In this example, we maintain a HashSet
to keep track of processed request IDs. When a new request comes in, we check if its ID is already in the set. If it is, we return a message indicating that the request has already been processed. If not, we add the ID to the set and proceed with the operation.
Output:
Request processed successfully.
The above code ensures that even if the same request is sent multiple times, it will only be processed once, maintaining idempotence.
Checking the Current State Before Execution
Another method to ensure idempotence is to check the current state of the resource before performing any operation. This approach is particularly useful in scenarios where the operation may change the state of the resource. Here’s how it can be implemented:
public class UserService {
private boolean isActive = false;
public String activateUser() {
if (isActive) {
return "User is already active.";
}
isActive = true;
return "User activated successfully.";
}
}
In this example, we have a UserService
class with an isActive
boolean flag. Before activating the user, we check if the user is already active. If they are, we return a message indicating that no action is needed. If not, we proceed to activate the user.
Output:
User activated successfully.
This method helps to ensure that the operation can be called multiple times without any unintended side effects, thus preserving the idempotent property.
Conclusion
Understanding idempotence in Java is essential for developers who want to build robust and reliable applications. By implementing idempotent operations, you can prevent unintended side effects from repeated requests, ensuring data integrity and consistency. Whether you choose to use unique request identifiers or check the current state before execution, the key is to design your methods in a way that they can handle multiple invocations gracefully. As you continue to develop your skills in Java, keep idempotence in mind, as it will significantly enhance the quality of your applications.
FAQ
-
What does idempotent mean in programming?
Idempotent refers to operations that can be performed multiple times without changing the result beyond the initial application. -
Why is idempotence important in web services?
Idempotence is crucial in web services to maintain data integrity and provide a consistent user experience, especially when requests may be repeated.
-
How can I implement idempotence in Java?
You can implement idempotence by using unique request identifiers or checking the current state before executing operations. -
Can idempotent operations improve application performance?
While idempotent operations focus on reliability and consistency, they can also improve performance by reducing unnecessary processing of repeated requests. -
Are all HTTP methods idempotent?
Not all HTTP methods are idempotent. For example, GET and PUT are idempotent, while POST is generally not.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook