How to Delay Few Seconds in Java
-
Make a Delay Using
Thread.sleep()
Method in Java -
Make a Delay Using
TimeUnit.sleep()
Method in Java -
Make a Delay Using
ScheduledExecutorService
in Java -
Make a Delay Using
ScheduledExecutorService
in Java
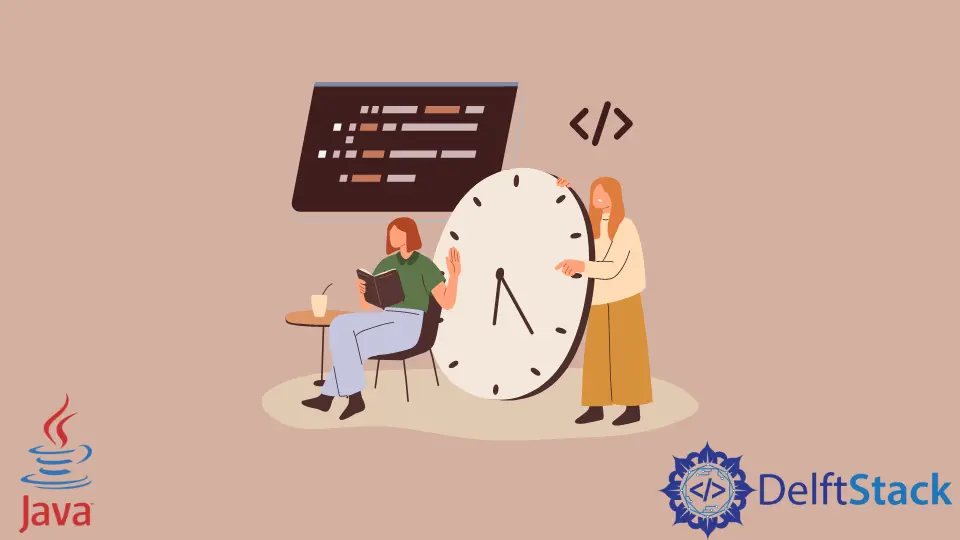
This tutorial introduces how to make a delay in Java and lists some example codes to understand it.
There are several ways to create delay, like TimeUnit.sleep()
, ScheduleAtFixedRate()
, and Thread.sleep()
methods, etc. Let’s see the examples.
Make a Delay Using Thread.sleep()
Method in Java
Thread
is a Java class that is used to create and execute tasks concurrently and provides a sleep()
method to pause the current execution for a while.
public class SimpleTesting {
public static void main(String[] args) {
try {
for (int i = 0; i < 2; i++) {
Thread.sleep(1000);
System.out.println("Sleep " + i);
}
} catch (Exception e) {
System.out.println(e);
}
}
}
Output:
Sleep 0
Sleep 1
Make a Delay Using TimeUnit.sleep()
Method in Java
In this example, we used the sleep()
method of TimeUnit
class, which is used to make an execution delay for the specified time. The TimeUnit
class belongs to a concurrent API package in Java.
import java.util.concurrent.TimeUnit;
public class SimpleTesting {
public static void main(String[] args) {
try {
for (int i = 0; i < 2; i++) {
TimeUnit.SECONDS.sleep(2);
System.out.println("Sleep " + i);
}
} catch (Exception e) {
System.out.println(e);
}
}
}
Output:
Sleep 0
Sleep 1
Make a Delay Using ScheduledExecutorService
in Java
Java provides a class ScheduledExecutorService
to schedule execution in a concurrent environment. We can use the run()
method inside it to execute the separate execution path. See the example below.
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class Main {
public static void main(String args[]) {
try {
final ScheduledExecutorService executorService = Executors.newSingleThreadScheduledExecutor();
executorService.scheduleAtFixedRate(new Runnable() {
@Override
public void run() {
executeTask();
}
}, 0, 1, TimeUnit.SECONDS);
} catch (Exception e) {
System.out.println(e);
}
}
private static void executeTask() {
System.out.println("Task Executing... ");
}
}
Output:
Task Executing...
Task Executing...
Task Executing...
Make a Delay Using ScheduledExecutorService
in Java
If you are working with Java 9 or higher version, you can use the method reference concept to call the method inside the scheduleAtFixedRate()
method. See the example below.
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class SimpleTesting {
public static void main(String[] args) {
try {
final ScheduledExecutorService executorService = Executors.newSingleThreadScheduledExecutor();
executorService.scheduleAtFixedRate(SimpleTesting::executeTask, 1, 2, TimeUnit.SECONDS);
} catch (Exception e) {
System.out.println(e);
}
}
private static void executeTask() {
System.out.println("Task Executing... ");
}
}
Output:
Task Executing...
Task Executing...
Task Executing...