How to Get Resource URL and Content in Java
-
Use the
getResource()
Function to Get Resource URL in Java -
Use
getResourceAsStream()
to Get Resource Content in Java
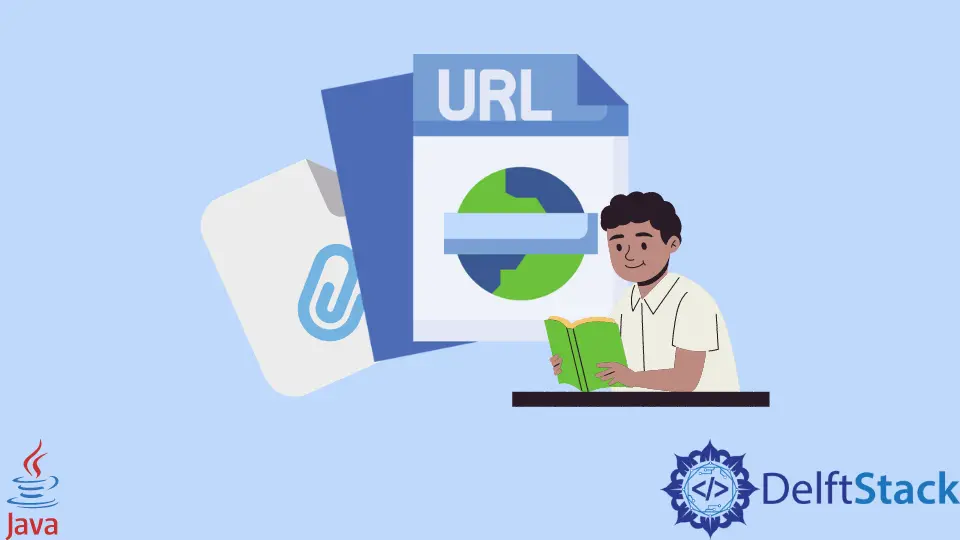
This tutorial will demonstrate how to get resource URLs and read resource files in Java with the getResource()
function.
Use the getResource()
Function to Get Resource URL in Java
We will use the getResource()
method in Java to get the URL of three files: image.png
, image1.png
, resourcetext.txt
.
We will pass resource URLs in strings in the body of the getResource()
function. The function then searches for the given resource string and returns an object containing a URL.
Syntax:
getResource(String);
public resource = yourclassname.getResource("Resource URL");
Example code:
/*//you will learn how to get image URL in the following program
//import statements
*/
import java.lang.*;
import java.net.URL;
public class getImageUrl {
public static void main(String[] args) throws Exception {
getImageUrl obj = new getImageUrl();
@SuppressWarnings("rawtypes") Class resource = obj.getClass();
URL imageurl = resource.getResource("/image.png");
System.out.println("Resource URL one is = " + imageurl);
URL imageurl2 = resource.getResource("/image2.png");
System.out.println("Resource URL two is = " + imageurl2);
URL texturl = resource.getResource("/textresource.txt");
System.out.println("Resource URL of the text file is = " + texturl);
}
}
Output:
Resource URL one is = file:/C:/Users/NAME/Desktop/JAVA/get%20resource%20url%20java/bin/image.png
Resource URL two is = file:/C:/Users/NAME/Desktop/JAVA/get%20resource%20url%20java/bin/image2.png
Resource URL of the text file is = file:/C:/Users/NAME/Desktop/JAVA/get%20resource%20url%20java/bin/textresource.txt
As we can see, we stored the three files in the string URL. Then we use the obj.getClass()
method to get the main class that receives the image URL.
The function getResource()
is the one that returns the URL.
Use getResourceAsStream()
to Get Resource Content in Java
Java reserves a method called getResourceAsStream()
to read files. The function returns an InputStream
object containing the specified resource of the class.
For the example below, we will use getResourceAsStream()
to read this file: /get resource URL java/src/readfile/GetResourceReadFile.java
. And the string getresourcefromhere = "readfile/example.json";
is where we store the JSON file.
Syntax:
private InputStream getFileFromResourceAsStream(String getresourcefromhere) {
ClassLoader cL = getClass().getClassLoader();
InputStream inputStream = cL.getResourceAsStream(getresourcefromhere);
return inputStream;
}
Check out the following complete program if you have understood the basic syntax.
This program works on every platform. You should pay attention to the main class and file directory management.
// import necessary packages
package readfile;
import java.io.*;
import java.nio.charset.StandardCharsets;
// start function
public class GetResourceReadFile {
private static final String String = null;
public static void main(String[] args) throws IOException, Exception {
GetResourceReadFile app = new GetResourceReadFile();
// get resource file
String getresourcefromhere = "readfile/example.json";
// use inputStream to return object containing resource
InputStream getresourceandreadit = app.getFileFromResourceAsStream(getresourcefromhere);
printInputStream(getresourceandreadit);
}
private InputStream getFileFromResourceAsStream(String getresourcefromhere) {
// load class
ClassLoader cL = getClass().getClassLoader();
InputStream inputStream = cL.getResourceAsStream(getresourcefromhere);
return inputStream;
}
private static void printInputStream(InputStream r) {
try (InputStreamReader sR = new InputStreamReader(r, StandardCharsets.UTF_8);
BufferedReader R = new BufferedReader(sR)) {
String GOT_IT = String;
// not necessary but will give you basic idea
if (GOT_IT == String) {
// you can print multiple files
while ((GOT_IT = R.readLine()) != null) {
// print file
System.out.println(GOT_IT);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
{
"File Name": "Demonstration File",
"File Type": "JSON FILE",
"File Reader": "getResource",
"File creation date:": 2/18/2022,
"Platform": {
"Langauge Type": "Programming",
"Langugae Name": "JAVA",
"Platform": "Oracle",
"Importance": "Very High"
},
"Development Environment": [
{ "JDK": "JAVA", "LATEST": "17" }
]
}
The whole program is the same as the previous example with URL. The only difference is the InputStream
and ClassLoader cL = getClass().getClassLoader();
.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn