Obtenga la URL del recurso y el contenido en Java
-
Use la función
getResource()
para obtener la URL del recurso en Java -
Use
getResourceAsStream()
para obtener contenido de recursos en Java
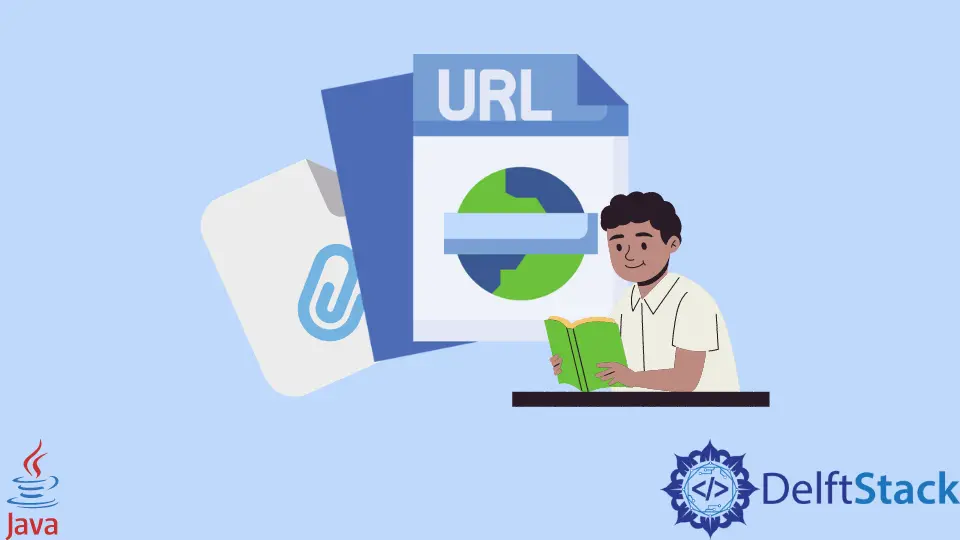
Este tutorial demostrará cómo obtener URL de recursos y leer archivos de recursos en Java con la función getResource()
.
Use la función getResource()
para obtener la URL del recurso en Java
Usaremos el método getResource()
en Java para obtener la URL de tres archivos: image.png, image1.png, resourcetext.txt.
Pasaremos las URL de los recursos en cadenas en el cuerpo de la función getResource()
. Luego, la función busca la cadena de recursos dada y devuelve un objeto que contiene una URL.
Sintaxis:
getResource(String);
public resource = yourclassname.getResource("Resource URL");
Código de ejemplo:
/*//you will learn how to get image URL in the following program
//import statements
*/
import java.lang.*;
import java.net.URL;
public class getImageUrl {
public static void main(String[] args) throws Exception {
getImageUrl obj = new getImageUrl();
@SuppressWarnings("rawtypes") Class resource = obj.getClass();
URL imageurl = resource.getResource("/image.png");
System.out.println("Resource URL one is = " + imageurl);
URL imageurl2 = resource.getResource("/image2.png");
System.out.println("Resource URL two is = " + imageurl2);
URL texturl = resource.getResource("/textresource.txt");
System.out.println("Resource URL of the text file is = " + texturl);
}
}
Producción :
Resource URL one is = file:/C:/Users/NAME/Desktop/JAVA/get%20resource%20url%20java/bin/image.png
Resource URL two is = file:/C:/Users/NAME/Desktop/JAVA/get%20resource%20url%20java/bin/image2.png
Resource URL of the text file is = file:/C:/Users/NAME/Desktop/JAVA/get%20resource%20url%20java/bin/textresource.txt
Como podemos ver, almacenamos los tres archivos en la cadena URL. Luego usamos el método obj.getClass()
para obtener la clase principal que recibe la URL de la imagen.
La función getResource()
es la que devuelve la URL.
Use getResourceAsStream()
para obtener contenido de recursos en Java
Java reserva un método llamado getResourceAsStream()
para leer archivos. La función devuelve un objeto InputStream
que contiene el recurso especificado de la clase.
Para el siguiente ejemplo, usaremos getResourceAsStream()
para leer este archivo: /get resource URL java/src/readfile/GetResourceReadFile.java
. Y la cadena getresourcefromhere = "readfile/example.json";
es donde almacenamos el archivo JSON.
Sintaxis:
private InputStream getFileFromResourceAsStream(String getresourcefromhere) {
ClassLoader cL = getClass().getClassLoader();
InputStream inputStream = cL.getResourceAsStream(getresourcefromhere);
return inputStream;
}
Consulte el siguiente programa completo si ha entendido la sintaxis básica.
Este programa funciona en todas las plataformas. Debe prestar atención a la clase principal y la gestión del directorio de archivos.
// import necessary packages
package readfile;
import java.io.*;
import java.nio.charset.StandardCharsets;
// start function
public class GetResourceReadFile {
private static final String String = null;
public static void main(String[] args) throws IOException, Exception {
GetResourceReadFile app = new GetResourceReadFile();
// get resource file
String getresourcefromhere = "readfile/example.json";
// use inputStream to return object containing resource
InputStream getresourceandreadit = app.getFileFromResourceAsStream(getresourcefromhere);
printInputStream(getresourceandreadit);
}
private InputStream getFileFromResourceAsStream(String getresourcefromhere) {
// load class
ClassLoader cL = getClass().getClassLoader();
InputStream inputStream = cL.getResourceAsStream(getresourcefromhere);
return inputStream;
}
private static void printInputStream(InputStream r) {
try (InputStreamReader sR = new InputStreamReader(r, StandardCharsets.UTF_8);
BufferedReader R = new BufferedReader(sR)) {
String GOT_IT = String;
// not necessary but will give you basic idea
if (GOT_IT == String) {
// you can print multiple files
while ((GOT_IT = R.readLine()) != null) {
// print file
System.out.println(GOT_IT);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Producción :
{
"File Name": "Demonstration File",
"File Type": "JSON FILE",
"File Reader": "getResource",
"File creation date:": 2/18/2022,
"Platform": {
"Langauge Type": "Programming",
"Langugae Name": "JAVA",
"Platform": "Oracle",
"Importance": "Very High"
},
"Development Environment": [
{ "JDK": "JAVA", "LATEST": "17" }
]
}
Todo el programa es el mismo que el ejemplo anterior con URL. La única diferencia es InputStream
y ClassLoader cL = getClass().getClassLoader();
.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn