How to Get HostName in Java
-
Use
InetAddress
Class to GetHostName
in Java -
Use
System
Class to GetHostName
in Java -
Use
Runtime
Class to GetHostName
in Java -
Use
ManagementFactory
to GetHostName
in Java -
Use
NetworkInterface
to GetHostName
in Java - Conclusion
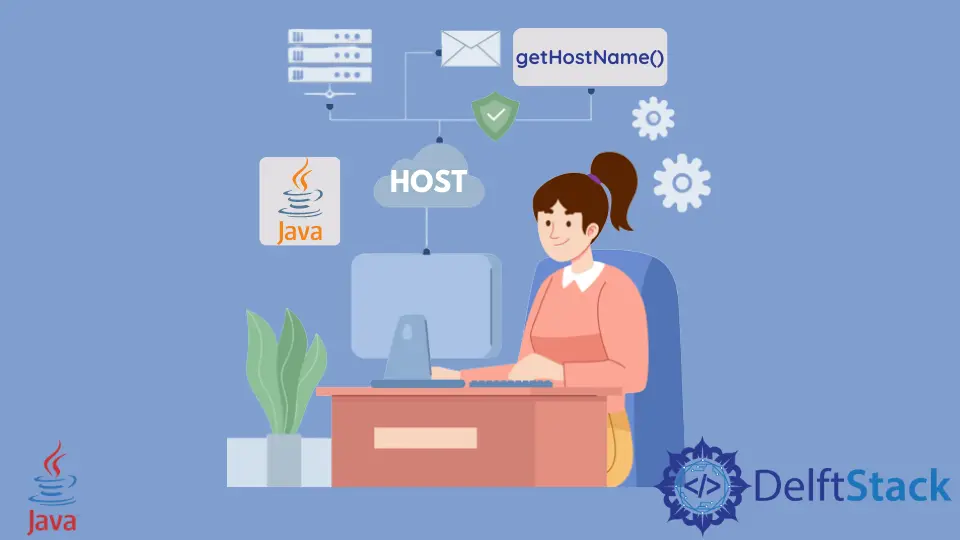
We look forward to gaining a comprehensive understanding of diverse methods for obtaining the hostname in Java through this article. By exploring various approaches, including InetAddress
, System
class, Runtime
, ManagementFactory
, and NetworkInterface
, the article aims to provide a well-rounded view of the available techniques.
Obtaining the hostname in Java is crucial for various reasons in the realm of application development. The hostname, which represents the unique name assigned to a device on a network, serves as a key identifier for the local machine.
In practical terms, knowing the hostname allows Java applications to customize their behavior based on the specific host environment. For instance, in network programming, applications may need to communicate with other devices by referring to them through their hostnames.
Additionally, system administration tasks often require the hostname for logging and monitoring purposes. Moreover, the hostname provides a means for applications to dynamically adapt to the host environment, ensuring optimal performance and functionality.
In essence, retrieving the hostname in Java is essential for creating versatile, context-aware applications that can seamlessly integrate with their host environments.
Use InetAddress
Class to Get HostName
in Java
Java’s InetAddress
class is a versatile tool for handling IP addresses and hostnames. Leveraging this class, we can easily obtain the hostname of the local machine.
The beauty of this approach lies in its simplicity and platform independence.
Let’s delve into a complete example:
import java.net.InetAddress;
public class HostnameExample {
public static void main(String[] args) {
try {
InetAddress localMachine = InetAddress.getLocalHost();
System.out.println("Hostname using InetAddress: " + localMachine.getHostName());
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this Java program, we start by importing the InetAddress
class, a useful tool for dealing with IP addresses and hostnames. Our class, named HostnameExample
, is designed to fetch and display the hostname.
The main
method is where the program execution begins. To retrieve the hostname, we use the InetAddress.getLocalHost()
method, obtaining an InetAddress
object representing the local machine.
We then invoke the getHostName()
method to extract the actual hostname. To handle potential exceptions like UnknownHostException
, we encapsulate the hostname retrieval in a try-catch
block.
Finally, the obtained hostname is printed to the console using System.out.println("Hostname using InetAddress: " + localMachine.getHostName());
.
Upon running this program, the output will display the hostname of the machine where the program is executed.
Hostname using InetAddress: YourHostName
The elegance of this method lies in its simplicity and cross-platform compatibility. By tapping into the capabilities of the InetAddress
class, we equip our Java applications with a robust mechanism for accessing essential information about the host environment.
Use System
Class to Get HostName
in Java
The System
class in Java provides access to various system-related properties and environment variables.
Leveraging this class, we can obtain the hostname in a platform-independent manner. The key lies in understanding the environment variables that store the hostname information.
Let’s dive into a complete example:
public class HostnameExample {
public static void main(String[] args) {
String hostname = System.getenv("COMPUTERNAME"); // On Windows
if (hostname == null || hostname.isEmpty()) {
hostname = System.getenv("HOSTNAME"); // On Unix/Linux
}
System.out.println("Hostname using System: " + hostname);
}
}
In our Java program, we kick things off by creating a class named HostnameExample
. The heart of the program lies in the main
method, serving as the starting point for execution.
To fetch the hostname, we employ a conditional approach.
Initially, we use System.getenv("COMPUTERNAME")
to access the hostname on Windows systems, extracting it from the COMPUTERNAME
environment variable. However, for non-Windows systems or instances where the COMPUTERNAME
variable is empty, we seamlessly shift to System.getenv("HOSTNAME")
to acquire the hostname on Unix/Linux platforms.
The conditional check ensures comprehensive coverage for both Windows and Unix/Linux scenarios, with the retrieved hostname assigned to the variable hostname
.
Finally, we display the obtained hostname on the console using System.out.println("Hostname using System: " + hostname);
. This approach provides a flexible and cross-platform method for Java applications to adapt to different operating environments.
When you run this program, the output will display the hostname of the machine, utilizing the appropriate environment variable based on the underlying operating system.
Hostname using System: YourHostName
This method proves versatile, catering to both Windows and Unix/Linux environments seamlessly.
Use Runtime
Class to Get HostName
in Java
Java’s Runtime
class provides an interface to interact with the runtime environment of the Java Virtual Machine (JVM). Leveraging this class, we can execute commands in the operating system’s shell, allowing us to obtain the hostname dynamically.
This approach provides flexibility and is platform-independent.
Let’s delve into a complete example:
public class HostnameExample {
public static void main(String[] args) {
try {
Process process = Runtime.getRuntime().exec("hostname");
java.util.Scanner scanner = new java.util.Scanner(process.getInputStream());
if (scanner.hasNext()) {
String hostname = scanner.next();
System.out.println("Hostname using Runtime: " + hostname);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
We commence by defining a class, succinctly named HostnameExample
, crafted specifically to house the logic for fetching hostnames. The program’s entry point is the main
method, orchestrating the entire execution.
To tap into the host environment, we employ the Runtime.getRuntime().exec("hostname")
method, which triggers the execution of the hostname
command in the operating system’s shell. This action yields a Process
object, essentially representing the spawned process.
Subsequently, a Scanner
object comes into play, initialized to read the output stream of the process, facilitating the capture of the elusive hostname. The hostname retrieval unfolds seamlessly with a check via scanner.hasNext()
, ensuring there is output to process.
Once confirmed, we acquire the hostname using scanner.next()
. The program concludes with the obtained hostname displayed on the console through System.out.println("Hostname using Runtime: " + hostname);
.
Upon running this program, the output will display the hostname of the machine where the program is executed.
Hostname using Runtime: YourHostName
This approach provides a dynamic and platform-independent method by executing shell commands.
By incorporating this technique into your applications, you empower them to dynamically adapt to the host environment, contributing to the development of more robust and flexible software solutions.
Use ManagementFactory
to Get HostName
in Java
Java’s ManagementFactory
class, introduced in Java 5, provides a wealth of information about the Java Virtual Machine (JVM) and its runtime environment.
Leveraging this class, we can access runtime information, including the hostname of the local machine. This approach is convenient and avoids the need for external processes or platform-specific commands.
Let’s delve into a complete example:
import java.lang.management.ManagementFactory;
public class HostnameExample {
public static void main(String[] args) {
String name = ManagementFactory.getRuntimeMXBean().getName();
String hostname = name.split("@")[1];
System.out.println("Hostname using ManagementFactory: " + hostname);
}
}
We commence by importing the ManagementFactory
class from the java.lang.management
package, setting the stage for the utilization of its capabilities. Our Java class, aptly named HostnameExample
, encapsulates the logic for retrieving and displaying the hostname.
To access runtime information, including the hostname, we employ the method ManagementFactory.getRuntimeMXBean().getName()
. This method yields a string encompassing details about the runtime environment, with the hostname embedded within.
With the obtained string, we perform a straightforward hostname extraction by splitting it using the @
symbol and selecting the second part. This step ensures the isolation of the hostname from the runtime information string.
The program culminates in a concise display of the extracted hostname on the console, achieved through System.out.println("Hostname using ManagementFactory: " + hostname);
. This method exemplifies a straightforward and effective approach for Java applications to dynamically access and showcase hostnames, demonstrating the seamless integration of simplicity and practicality.
Upon running this program, the output will display the hostname of the machine where the program is executed.
Hostname using ManagementFactory: YourHostName
This approach provides a concise and platform-independent method, harnessing the capabilities of the Java Management Extensions (JMX). By incorporating this technique into your applications, you enhance their awareness of the runtime environment, enabling them to dynamically adapt and respond to the host environment’s nuances.
Use NetworkInterface
to Get HostName
in Java
Java’s NetworkInterface
class is a powerful tool for interacting with network interfaces and their associated information. Leveraging this class, we can access details about the host, including the hostname.
This approach offers a versatile and platform-independent method for obtaining the hostname.
Let’s delve into a complete example:
import java.net.NetworkInterface;
import java.net.SocketException;
import java.util.Enumeration;
public class HostnameExample {
public static void main(String[] args) {
try {
Enumeration<NetworkInterface> networkInterfaces = NetworkInterface.getNetworkInterfaces();
while (networkInterfaces.hasMoreElements()) {
NetworkInterface networkInterface = networkInterfaces.nextElement();
if (!networkInterface.isLoopback() && networkInterface.isUp()) {
String hostname = networkInterface.getDisplayName();
System.out.println("Hostname using NetworkInterface: " + hostname);
break;
}
}
} catch (SocketException e) {
e.printStackTrace();
}
}
}
We kick off by importing the necessary classes, with a focus on integrating NetworkInterface
for streamlined interaction with network interfaces. The class, named HostnameExample
, takes center stage, containing the logic to effortlessly retrieve and showcase the hostname.
The program’s flow navigates through obtaining an enumeration of network interfaces using NetworkInterface.getNetworkInterfaces()
. A meticulous iteration over these interfaces follows, searching for a suitable one.
During this process, loopback interfaces (networkInterface.isLoopback()
) are skipped, and preference is given to an active interface (networkInterface.isUp()
).
The hostname retrieval phase is concise and efficient, accomplished through networkInterface.getDisplayName()
. This method ensures the seamless extraction of the desired hostname from the selected network interface.
The program culminates in the elegant display of the obtained hostname on the console, facilitated by System.out.println("Hostname using NetworkInterface: " + hostname);
.
Upon running this program, the output will display the hostname of the machine based on the selected network interface.
Hostname using NetworkInterface: YourHostName
This approach provides a dynamic and platform-independent method for accessing host information. By selecting an appropriate network interface, we can extract the hostname, enabling applications to be more aware and responsive to the host environment.
Conclusion
Obtaining the hostname in Java through methods such as InetAddress
, System
, Runtime
, ManagementFactory
, and NetworkInterface
is crucial for applications to dynamically adapt to and interact with their host environments.
Whether for network communication, system administration, or customization based on runtime conditions, accessing the hostname provides essential information for optimal program functionality. Each method offers its unique advantages, catering to diverse scenarios and contributing to the overall versatility and awareness of Java applications.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn