How to Generate MD5 Hash in Java
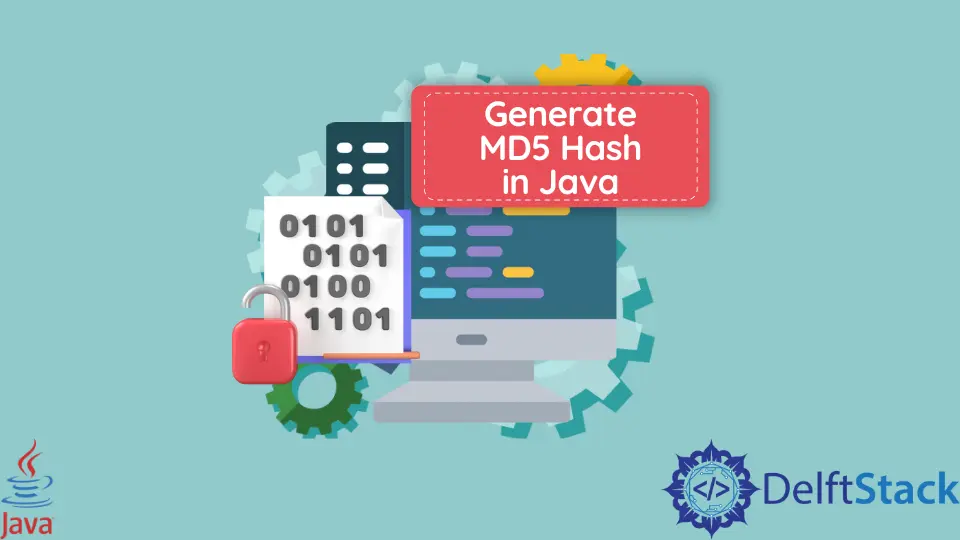
In this article, we will understand the MD5 algorithm to generate the hash of data and how we can produce the MD5 hash of data in Java.
MD5 Hashing Algorithm in Java
The MD5 hashing algorithm is a cryptographic hashing algorithm, and it is largely used as a checksum of the data files. We can use this algorithm to generate a 128-bit cryptographic hash of our data.
The MD5 hashing algorithm is widely used because it is much faster than the modern secure hashing algorithms.
Use MD5 Hash in Java
Java is widely used for file transfer and server-side programming; it is not surprising to find a library to generate MD5 hash. Java provides us a MessageDigest
class, a child class of the MessageDigestSpi
found in Java’s 'security'
package.
To generate the MD5 hash in Java,
-
Import the
MessageDisgest
class from the Java security package. -
Convert our data into a stream of bytes before getting the message digest.
-
Then, invoke the
getInstance()
method to create an instance of the MD5 hashing algorithm.Example :
public static MessageDigest getInstance(String algorithm) throws NoSuchAlgorithmException
-
We will invoke the
digest()
method by passing the data we want to get the MD5 hash.Example:
public byte[] digest(byte[] input)
-
Store the message digest as a stream of bytes into a byte array.
-
Lastly, convert the message digest from bytes to string.
Let us understand the above approach using a working code in Java.
Code Snippet:
import java.math.BigInteger;
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.*;
public class md5Test {
public static void main(String[] args) {
String str;
Scanner scan = new Scanner(System.in);
str = scan.nextLine();
System.out.println("Your input: " + str);
byte[] msg = str.getBytes();
byte[] hash = null;
try {
MessageDigest md = MessageDigest.getInstance("MD5");
hash = md.digest(msg);
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
StringBuilder strBuilder = new StringBuilder();
for (byte b : hash) {
strBuilder.append(String.format("%02x", b));
}
String strHash = strBuilder.toString();
System.out.println("The MD5 hash: " + strHash);
}
}
Output:
Hello, Peter
Your input: Hello, Peter
The MD5 hash: 945062a2fee23e0901b37fcb5cd952c9
Java is so awesome.
Your input: Java is so awesome.
The MD5 hash: 601835019da217140c2755c919ee18c2
Use MD5 Hash on Large Data in Java
If you have large data or read the data in chunks, then use the update()
method.
Example:
public void update(byte[] input)
Each time you read a chunk of data, you should call the update()
method by passing the current chunk. After all the data is read, use the following polymorphic form of the digest()
method.
Example:
public byte[] digest() // It means you will pass no parameter to the `digest()` method.
For demonstration, you can see the following example.
Code Snippet:
import java.math.BigInteger;
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.*;
public class md5Test {
public static void main(String[] args) {
String str;
Scanner scan = new Scanner(System.in);
System.out.println("Enter message:");
str = scan.nextLine();
System.out.println("Your input: " + str);
byte[] hash = null;
MessageDigest md = null;
try {
md = MessageDigest.getInstance("MD5");
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
md.update(str.getBytes());
System.out.println("Enter message:");
str = scan.nextLine();
md.update(str.getBytes());
hash = md.digest();
StringBuilder strBuilder = new StringBuilder();
for (byte b : hash) {
strBuilder.append(String.format("%02x", b));
}
String strHash = strBuilder.toString();
System.out.println("The MD5 hash: " + strHash);
}
}
Output:
Enter message:
Hello Java
Your input: Hello Java
Enter message:
I'm Peter
The MD5 hash: 9008f99fa602a036ce0c7a6784b240b1
Conclusion
One of the fundamental security measures that we should ensure while sharing the data is to ensure data integrity. Therefore, we need a hashing algorithm that produces a checksum of the data shared with the receiver to ensure integrity.
We have understood the method to generate the MD5 checksum using the MessageDigest
class and its methods. It’s best to be cautious when reading data in chunks so that you don’t end up with incorrect results.