How to Downcast in Java
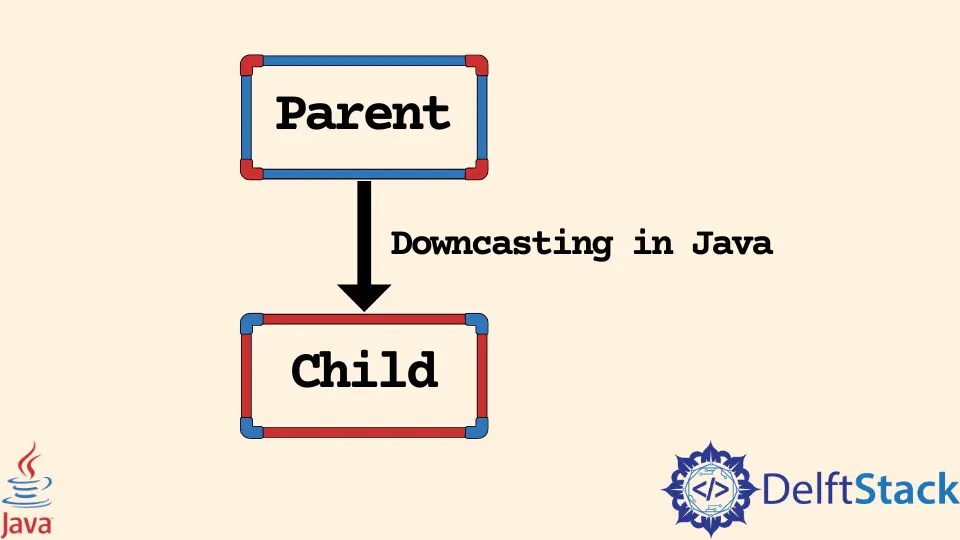
If you are working with Java, you may work with TypeCasting
, UpCasting
, or DownCasting
. The purpose of their use is to convert from one type to another.
This can be with variables or objects. For an object, there are two kinds of transformation available.
The first one is Parent to Child and the second one is Child to Parent.
Here, the conversion from the Child to Parent is known as the UpCasting
, and the conversion from Parent to a Child is known as the DownCasting
. We can implicitly or explicitly perform the UpCasting
, but we can only perform DownCasting
explicitly.
In this article, we will look at the details of DownCasting
. Also, we will describe the topic by using necessary examples and explanations to make the topic easier.
Downcasting in Java
Now we are going to see an example regarding DownCasting
.
class ParentClass {
String name;
void showMessage() {
System.out.print("Parent class method is called");
}
}
class ChildClass extends ParentClass {
int age;
@Override
void showMessage() {
System.out.print("The child class method is called");
}
}
class Downcasting {
public static void main(String[] args) {
ParentClass Parent = new ChildClass();
Parent.name = "Alex";
ChildClass Child = (ChildClass) Parent;
Child.age = 21;
System.out.print("Name:" + Child.name + " ");
System.out.println("Age:" + Child.age);
Child.showMessage();
}
}
Let’s explain the code part by part. First of all, we created a parent class named ParentClass
, and then we created a child class named ChildClass
and extends it with the ParentClass
.
On the ChildClass
, we performed overriding by @Override
.
After that, we created another class named Downcasting
, our controlling class. In the class, we created an object from the ParentClass
.
Then we downcast an object with the line ChildClass Child = (ChildClass)Parent;
.
Lastly, we print all the data. Now after executing the above Java program.
You will get an output like the one below.
Name:Alex Age:21
The child class method is called
Compiler Error When You Try to Downcast Implicitly
In the example above, we performed the downcasting explicitly. If you try to downcast implicitly, then you may get an error.
Check out the next example where we tried to downcast implicitly.
class ParentClass {
String name;
void showMessage() {
System.out.print("Parent class method is called");
}
}
class ChildClass extends ParentClass {
int age;
@Override
void showMessage() {
System.out.print("Child class method is called");
}
}
class Downcasting {
public static void main(String[] args) {
ParentClass Parent = new ChildClass();
Parent.name = "Alex";
ChildClass Child = new ParentClass();
Child.age = 21;
System.out.print("Name:" + Child.name + " ");
System.out.println("Age:" + Child.age);
Child.showMessage();
}
}
If you try to compile the above program, you will get an error like the one below.
/tmp/AMCRVnDhlV/Downcasting.java:22: error: incompatible types: ParentClass cannot be converted to ChildClass
ChildClass Child = new ParentClass();
^
1 error
Please keep in mind that DownCasting
needs to be done externally. By using DownCasting
, a child object can get the properties of its parent object.
Please note that the code examples shared here are in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn