How to Double max value in Java
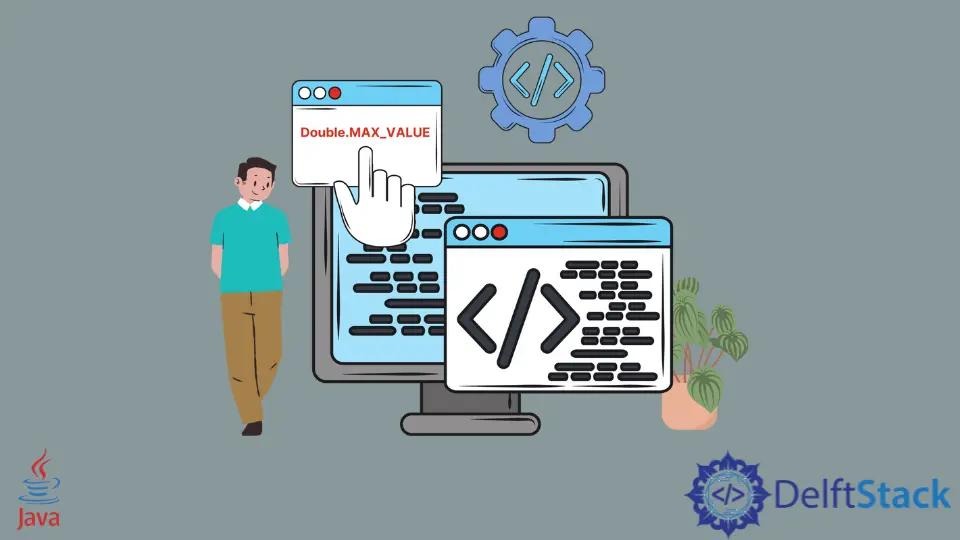
This tutorial introduces what is double max value in Java and how to calculate a max value for the double data type in Java.
Introduction to Double.MAX_VALUE
In Java, Double.MAX_VALUE
is a constant that represents the maximum finite value that can be stored in a double
data type. It is part of the Double
class, which is a wrapper class for the primitive data type double
.
The syntax to use Double.MAX_VALUE
is as follows:
double maxValue = Double.MAX_VALUE;
Here, maxValue
is a variable of type double
, and it is assigned the maximum finite value that can be represented by a double
.
In Java, Double.MAX_VALUE
is a constant representing the maximum finite positive value that can be represented by the double
data type. The value of Double.MAX_VALUE
is approximately 1.7976931348623157 x 10^308
.
To represent this in code:
public class SimpleTesting {
public static void main(String[] args) {
double max_value = Double.MAX_VALUE;
System.out.println("Double.MAX_VALUE: " + max_value);
}
}
When you run this code, it will output:
Double.MAX_VALUE: 1.7976931348623157E308
The E308
at the end of the output denotes that the value is in scientific notation, indicating that it is multiplied by 10 to the power of 308. This represents the upper limit of positive finite values that can be stored in a double
variable in Java.
Values greater than this would result in positive infinity when represented as a double
.
The double
data type in Java is a 64-bit double-precision floating-point type according to the IEEE 754 standard. It can represent a wide range of numerical values, including very large and very small numbers, with a high level of precision.
However, it has limitations due to the finite precision of floating-point representation.
Double.MAX_VALUE
is useful in scenarios where you need to represent or compare very large numbers within the limits of double-precision floating-point representation. For example, if you are working with computations involving extremely large numerical values and want to check if a particular value exceeds the maximum representable finite value, you can use Double.MAX_VALUE
for comparison.
Keep in mind that Double.MAX_VALUE
is not to be confused with positive infinity (Double.POSITIVE_INFINITY
), which represents a value that exceeds the maximum finite value that can be represented in a double
.
Java Double Max Value
Here, Double.MAX_VALUE
steps in as a sentinel, representing the maximum finite positive value that can be held by a double
. Its utility becomes apparent in scenarios where you need to ascertain if a calculated or user-inputted value falls within the bounds of representability.
Let’s delve into a practical example to grasp the usage of Double.MAX_VALUE
in a real-world scenario. Consider a simple Java program that validates user-inputted numerical values against the maximum representable value.
import java.util.Scanner;
public class MaxValueExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a numerical value: ");
double userInput = scanner.nextDouble();
if (userInput > Double.MAX_VALUE) {
System.out.println("Input exceeds the maximum representable value.");
} else {
System.out.println("Input is within the valid range.");
}
}
}
Let’s break down the code step by step. First, we begin by importing the Scanner
class to handle user input.
Next, we declare a class named MaxValueExample
. The program’s execution initiates from the main
method.
We proceed to initialize a Scanner
object for user input. The program prompts the user to enter a numerical value, and we capture and store it in the variable userInput
.
Finally, we compare this user input against the maximum representable value for a double
, Double.MAX_VALUE
, and based on the result, we print an appropriate message. This systematic breakdown helps us understand how each part contributes to the functionality of the Java program.
Assume the user enters a value:
Enter a numerical value: 150787654654
The program would output:
Input is within the valid range.
In this example, we’ve explored the practical application of Double.MAX_VALUE
by creating a program that ensures user input remains within the representable range of the double
data type. This simple yet effective usage showcases how understanding the limits of data types can enhance the robustness of your Java applications.
Conclusion
Understanding and utilizing Double.MAX_VALUE
is essential in scenarios where precision and range are critical. This article demonstrated a practical example, showing how to use this constant to validate and handle large numerical inputs effectively.
Whether you are dealing with financial calculations, scientific simulations, or input validation, harnessing the power of Double.MAX_VALUE
ensures your code remains resilient against excessively large numerical values.